How to Use ODBC Functions in PHP
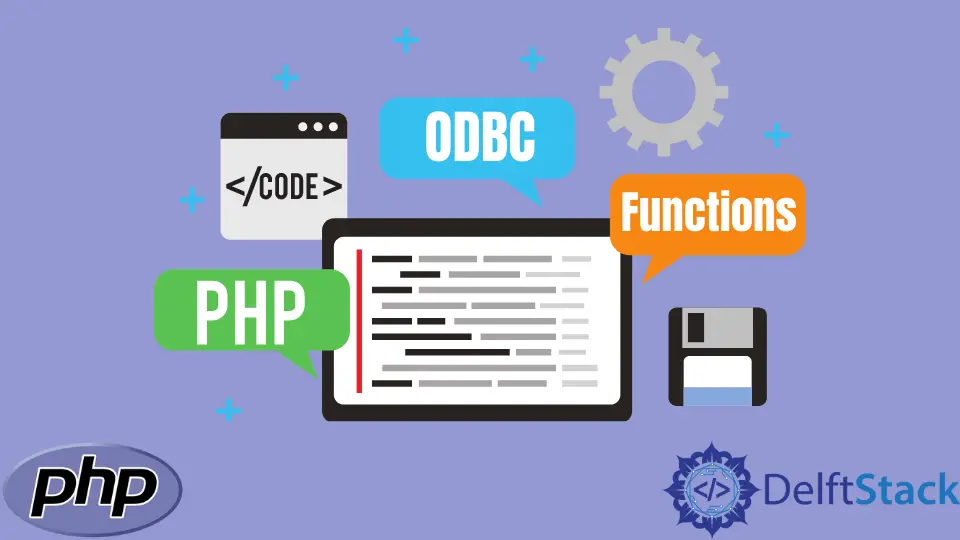
This article will introduce how to use ODBC and its functions in PHP.
Use ODBC Functions in PHP
Open Database Connectivity (ODBC) is an API that lets us access the database from a wide range of database applications. We can use the ODBC with MySQL database as well.
To work with the MySQL database, we need an ODBC connecter driver. We can download the driver from here.
PHP provides functions to connect the database with the ODBC. We can use the odbc_connect()
function to establish a connection the database.
Likewise, we can use the odbc_exec()
to execute the query. Assuming we have a driver installed and set up, we will demonstrate how to connect to a database and execute the query.
To establish a database connection, we need the details like driver, hostname, database, username, and password. The odbc_connect()
function takes these details as parameters.
For example, create variables $server
, $database
, $user
, $password
, and $driver
and store the relevant information in these variables.
Next, create a $connection
variable and assign the following values.
"DRIVER=$driver;
SERVER=$server;
DATABASE=$database";
Next, use the odbc_connect()
function and provide the variables $connection
, $user
and $password
as the parameters in order. Assign the function to a $conn
variable.
The variable returns a boolean value.
Now, we will check if the connection to the database is established and run a query. Write an if
condition with $conn
as a parameter.
Inside, create a $mail
variable and store the email that will be checked against the database. Next, create a $query
variable and write a SQL query in it.
The query is shown below.
SELECT COUNT(*) AS userEmail FROM users WHERE email = '$mail'
The query returns the number of entries in the users
table whose email
field has the value of the $mail
variable. After writing the query, execute it with the odbc_exec()
function.
Write the variables $conn
and $query
as the parameters in the function. Next to the function, use the or
operator and the die
function that contains the odbc_errormsg()
function.
Assign the whole operation to the $result
variable. If the query fails, the script will die, displaying the error message.
Next, check the $result
variable with the if
condition and display result found
with echo
inside the if
block.
Here, we used the database odbcd
, and the server is localhost. The username is root with no password.
The database has the table users
in it. The table contains the following data.
+----+---------+--------+-----------------+
| id | role | name | email |
+----+---------+--------+-----------------+
| 1 | admin | harry | admin@email.com |
| 2 | user | joe | user@email.com |
+----+---------+--------+-----------------+
The database matches the email in the $mail
variable, displaying the result shown in the output section. In this way, we can run the ODBC functions communicating with the SQL database in PHP.
$server = "localhost";
$database = "odbcdb";
$user = "root";
$password = "";
$driver = "MYSQL ODBC 3.51 DRIVER"
$connection = "DRIVER=$driver; SERVER=$server; DATABASE=$database";
$conn = odbc_connect($connection, $user, $password);
if ($conn) {
$mail = 'user@email.com';
$query = "SELECT COUNT(*) AS userEmail FROM users WHERE email = '$mail'";
$results = odbc_exec($conn, $query) or die(odbc_errormsg());
if( $results ) {
echo 'result found';
}
}
Output:
result found
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn