How to Call Function From String in PHP
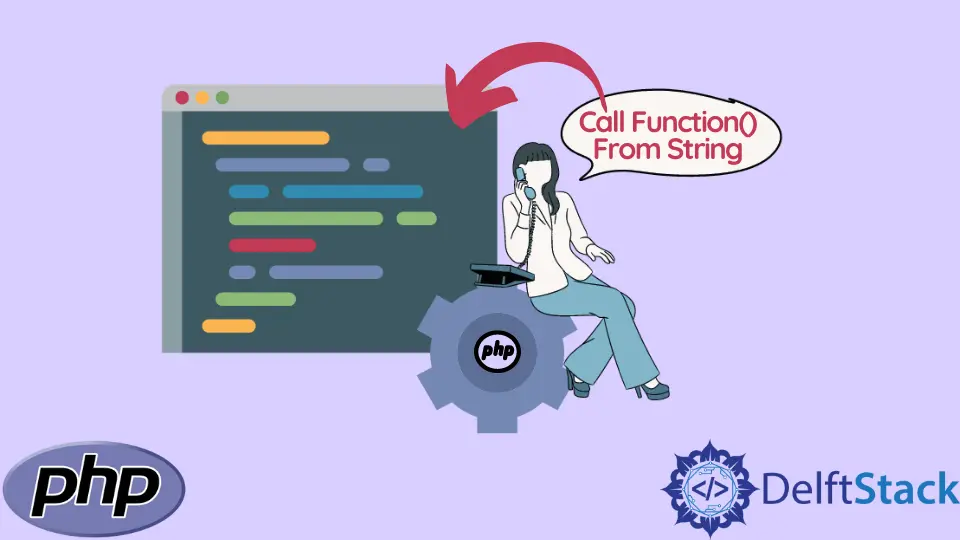
PHP is a versatile scripting language that allows developers to create dynamic web applications with ease. One of its powerful features is the ability to call functions using strings or variables. This capability can enhance your code’s flexibility and maintainability, making it easier to work with dynamic data.
In this tutorial, we will explore various methods to call functions from strings in PHP. Whether you’re a beginner or an experienced programmer, understanding this concept will help you write cleaner and more efficient code. Let’s dive into the different approaches to achieve this and see how they work with practical examples.
Using Variable Functions
One of the simplest ways to call a function by its name stored in a variable is by using variable functions. In PHP, if you assign the name of a function to a variable, you can invoke that function by referencing the variable. This method is straightforward and very effective for dynamic function calls.
Here’s a quick example:
function greet() {
return "Hello, World!";
}
$functionName = "greet";
$result = $functionName();
echo $result;
Output:
Hello, World!
In this example, we defined a function called greet
that simply returns a greeting message. We then assigned the string “greet” to the variable $functionName
. By using $functionName()
to call the function, we effectively invoked greet
, and the output was the expected greeting. This method is particularly useful when you need to call different functions based on user input or other dynamic conditions.
Using Call User Function
Another powerful method for calling functions from strings in PHP is by using the call_user_func()
function. This built-in PHP function allows you to call a function by name, passing any arguments as needed. This method provides additional flexibility, especially when you want to call functions that may take parameters.
Here’s how you can implement it:
function add($a, $b) {
return $a + $b;
}
$functionName = "add";
$result = call_user_func($functionName, 5, 10);
echo $result;
Output:
15
In this code, we created a function called add
that takes two parameters and returns their sum. By using call_user_func()
, we passed the function name as a string along with the parameters 5 and 10. The result was 15, demonstrating how this method can be used to call functions dynamically while also providing arguments. This is particularly useful in scenarios where function names are determined at runtime, making your code more adaptable.
Using Call User Func Array
When you need to call a function and pass an array of parameters, call_user_func_array()
is the way to go. This function allows you to call a function with an array of parameters instead of passing them one by one. This can be particularly beneficial when dealing with a variable number of arguments.
Here’s an example:
function multiply($a, $b) {
return $a * $b;
}
$params = [4, 5];
$result = call_user_func_array('multiply', $params);
echo $result;
Output:
20
In this example, we defined a function called multiply
that takes two parameters and returns their product. Instead of passing the parameters directly, we created an array called $params
containing the values 4 and 5. By using call_user_func_array()
, we passed the function name and the array of parameters, resulting in the output of 20. This method is especially handy when the number of arguments can vary, allowing for more flexible function calls.
Conclusion
Calling functions from strings in PHP opens up a realm of possibilities for writing dynamic and maintainable code. Whether you opt for variable functions, call_user_func()
, or call_user_func_array()
, each method offers unique advantages that can enhance your programming experience. Understanding these techniques will not only improve the flexibility of your applications but also help you write cleaner and more efficient code. As you continue to explore PHP, keep these methods in mind, and you’ll find yourself creating more adaptable solutions.
FAQ
-
How can I call a function stored in a variable in PHP?
You can call a function stored in a variable by simply referencing the variable followed by parentheses, like$variableName()
. -
What is the purpose of call_user_func() in PHP?
Thecall_user_func()
function allows you to call a function by its name as a string, enabling you to pass arguments dynamically. -
Can I use call_user_func_array() for functions with variable arguments?
Yes,call_user_func_array()
is ideal for calling functions that require an array of parameters, allowing for a flexible number of arguments. -
What are the advantages of calling functions dynamically?
Calling functions dynamically allows for more adaptable code, making it easier to handle different scenarios, user inputs, and varying function requirements. -
Are there performance implications when using these methods?
While these methods offer flexibility, they may have slight performance overhead compared to direct function calls. However, the trade-off is often worth it for the added flexibility.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook