How to Properly Format a Number With Leading Zeros in PHP
- Use a String to Substitute the Number
-
substr()
to Add Leading Zeros in PHP -
printf()
/sprintf()
to Add Leading Zeros in PHP -
str_pad()
to Add Leading Zeros in PHP
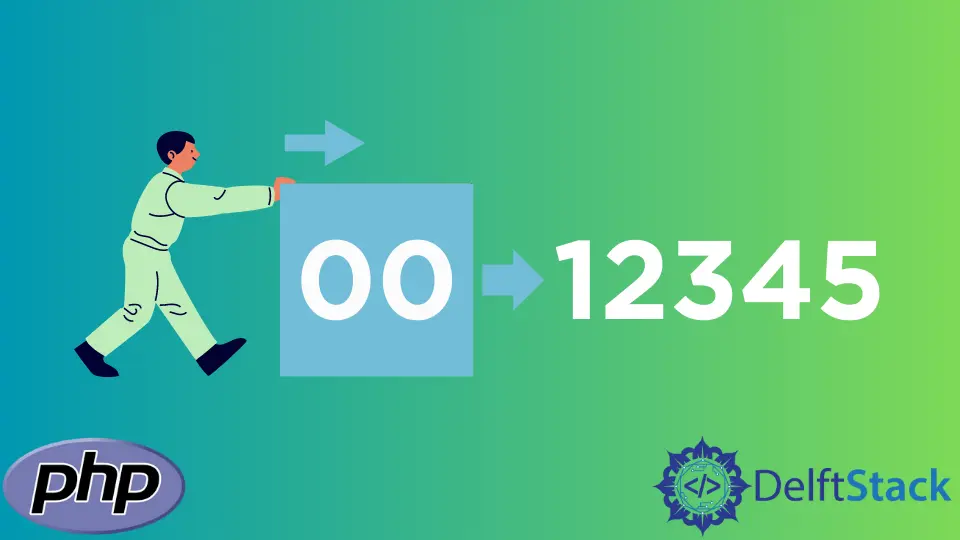
In PHP, numbers or integers with leading zeros can have different values with unexpected results.
Example:
$number = 0987654321; //this is an octal number
$number = 0x987654321 // this is a hexadecimal number
$number = 0b0987654321 // this is a binary number
To make sure that the number will not lose its natural
meaning, there are several ways to try like using a string instead of a number or by using different functions such as substr
, printf()
/ sprintf()
and str_pad
.
Use a String to Substitute the Number
The easiest approach; just simply to use string as a substitute for the number.
$number = "0987654321";
When To Use:
- There’s no required length of the output.
- There’s no exception to the number that it always need a leading zero.
substr()
to Add Leading Zeros in PHP
This method clips the numbers from the left when the string length exceeded.
If the start
is negative, the returned string will start from the start'th
character from the end of the string.
Example:
$number = 98765;
$length = 10;
$string = substr(str_repeat(0, $length).$number, - $length);
//output: 0000098765
When To Use:
- When there’s a fixed length of the output string.
- Adding zeros when the string is less than the length.
printf()
/sprintf()
to Add Leading Zeros in PHP
To pad an output for a fixed length, when the input is less than the length, and return the string when input is greater.
Example:
$length = 10;
$char = 0;
$type = 'd';
$format = "%{$char}{$length}{$type}"; // or "$010d";
//print and echo
printf($format, 987654321);
//store to a variable
$newFormat = sprintf($format, 987654321);
// output: 0987654321
In the example, the fixed length is set to 10 and the input length is 9, so it adds one zero
in the left if using printf()
/sprintf
.
sprintf()
Parameter Values
Parameter | Description |
---|---|
format (Required) |
The string and how to format the variables. Possible format values: %% - Percent sign%b - Binary%c - Character referenced to ASCII%d - decimal number (negative or positive)%e - Lowercase scientific notation%E - Uppercase scientific notation%u - Unsigned decimal number%f - Float number (local settings aware)%F - Float number (not local settings aware)%g - shorter version of %e and %f %G - shorter version of %E and %F %o - Octal%s - String%x - Hexadecimal (lowercase)%X - Hexadecimal (uppercase) |
arg1 (Required) |
To be inserted at the first % sign |
arg2 (Optional) |
To be inserted at the second % sign |
argg++ (Optional) |
To be inserted at the third, fourth, etc. % sign |
Note:
- If the input string length is greater than or equal to the pad length, it will only return the string - no characters will be omitted.
- Padding is only added the length of the input is less than the padding length.
str_pad()
to Add Leading Zeros in PHP
This method will pad a string to a new length of specified characters.
Example:
$length = 7;
$string = "12345";
echo str_pad($string,$length,"0", STR_PAD_LEFT);
//output: 0012345
The example above will add the zero
to the specified string until it matches the specified length (which is 7
in this case).
str_pad()
Parameters List
Parameter | Description |
---|---|
string |
The string to pad |
length |
Specifies the new length of the string. Note: If the value is less than the length of the string, nothing will happen. |
pad_string |
Specifies the string to use for padding. The default value is whitespace. |
pad_type |
Specifies where to pad the string. Accepted Values: STR_PAD_BOTH - This will pad both sides of the string.STR_PAD_LEFT - Pad the left side of the stringSTR_PAD_RIGHT - Pad to the right side of the string |