How to Check if Function Exists in PHP
-
Use
function_exists()
to Check Availability of Built-In Function in PHP -
Use
function_exists()
to Check User Defined Functions in PHP - Check All the Functions That Exist in the Environment in PHP
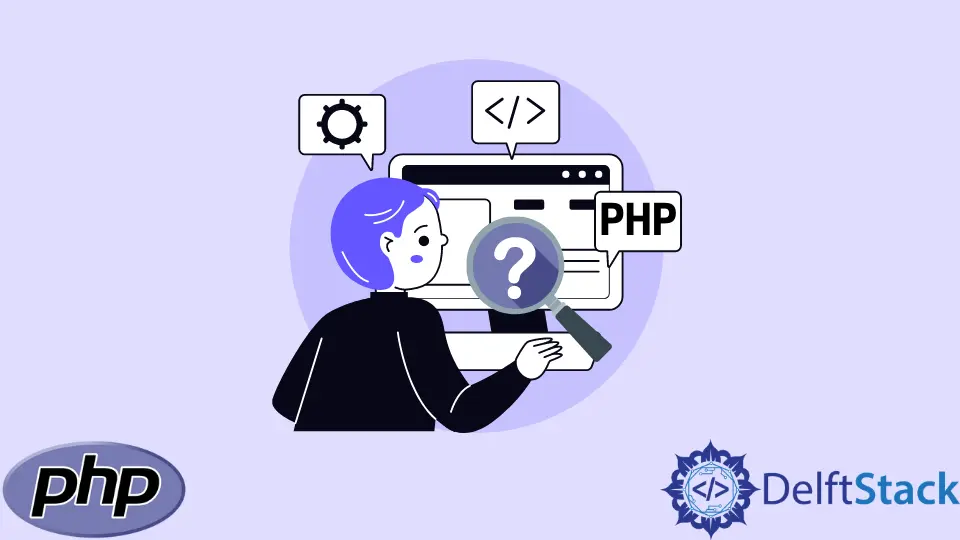
PHP has a built-in function called function_exists()
to check if a function exists or not which can check any built-in or defined functions. This tutorial demonstrates how to use function_exists()
in PHP.
Use function_exists()
to Check Availability of Built-In Function in PHP
The return value of function_exists()
is boolean. Let’s check for the functions from different libraries.
Example:
<?php
//Check a function from cURL library
if (function_exists('curl_close'))
{
echo "The function curl_close is available.<br>";
}
else
{
echo "The function curl_close is not available.<br>";
}
//Check a function from gettext library
if (function_exists('gettext()'))
{
echo "The function gettext() is available.<br>";
}
else
{
echo "The function gettext() is not available.<br>";
}
//Check a function from ftp library
if (function_exists('ftp_alloc'))
{
echo "The function ftp_alloc() is available.<br>";
}
else
{
echo "The function ftp_alloc() is not available.<br>";
}
//Check a function from GD library
if (function_exists('imagecreatefromgif'))
{
echo "The function imagecreatefromgif is available.<br>";
}
else
{
echo "The function imagecreatefromgif is not available.<br>";
}
?>
The code checks the functions from cURL
, gettext
, ftp
, and gd
library. Two of the libraries are enabled, and the other two are disabled.
Output:
The function curl_close is available.
The function gettext() is not available.
The function ftp_alloc() is not available.
The function imagecreatefromgif is available.
Use function_exists()
to Check User Defined Functions in PHP
function_exists()
can check for the functions defined before or after checking.
Example:
<?php
function demofunction()
{
//Anything
}
if (function_exists('demofunction')) {
echo "demofunction exists<br>";
}
else{
echo "demofunction doesn't exists.<br>";
}
if (function_exists('demofunction1')) {
echo "demofunction1 exists<br>";
}
else{
echo "demofunction1 doesn't exists.<br>";
}
function demofunction1()
{
//Anything
}
?>
The code above checks for two functions. One is defined before and one after the checking.
Output:
demofunction exists
demofunction1 exists
Check All the Functions That Exist in the Environment in PHP
PHP also has a built-in function to check all the functions in the environment.
Example:
<?php
var_dump( get_defined_functions());
?>
The output will get all the functions that exist in the environment. The output is a very large array, so we minimized it to a few members.
Output:
array(2) { ["internal"]=> array(1224) { [0]=> string(12) "zend_version" [1]=> string(13) "func_num_args" [2]=> string(12) "func_get_arg" [3]=> string(13) "func_get_args" [4]=> string(6) "strlen" [5]=> string(6)...
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook