How to Save Image From URL in PHP
-
Save Image From URL Using
file_get_contents()
andfile_put_contents()
in PHP -
Save Image From URL Using
cURL
-
Save Image From URL Using the
copy()
Function in PHP -
Save Image From URL Using
fread()
andfwrite()
in PHP -
Save a
gzip
Image in PHP
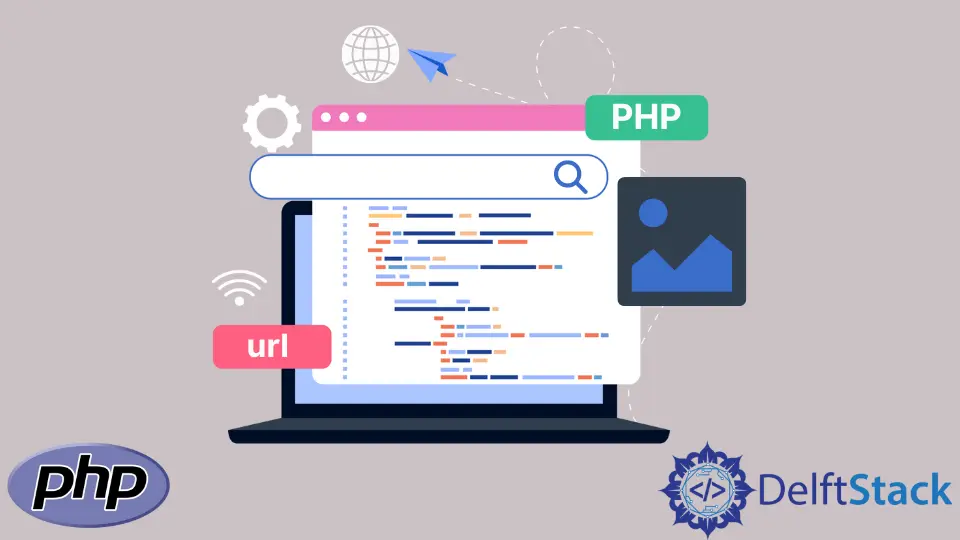
This article teaches five methods to save an image from a URL in PHP. These methods will use functions like file_put_contents()
, copy()
, fopen()
, fread()
, fwrite()
, and gzdecode()
.
Save Image From URL Using file_get_contents()
and file_put_contents()
in PHP
PHP file_get_contents()
will read a file into a string while file_put_contents()
can write that string to a file. Combining both functions will let you save an image from a URL.
First, you turn the image from the URL into a string using file_get_contents()
, and then you use file_put_contents()
to save this string into a file. The result will be your copy of the image from the URL.
In the following, we save an image from Imgur using file_get_contents()
and file_put_contents()
. Also, we rename the image to 01_computer_image.png
, but you can use another name and a valid image extension.
<?php
// The image is from Unsplash, and we've uploaded
// it to Imgur for this article.
$image_url = 'https://i.imgur.com/NFyDQTj.jpeg';
// Define the image location. Here, the location
// is the saved_images folder.
$image_location = 'saved_images/01_computer_image.png';
// Use file_put_contents to grab the image from
// the URL and place it into the image location
// with its new name.
if (file_put_contents($image_location, file_get_contents($image_url))) {
echo "Image saved successfully";
} else {
echo "An error code, please check your code.";
}
?>
Output:
Save Image From URL Using cURL
cURL
is a command-line tool to transfer data using network protocols. Since the image exists in the URL on a server, you can start the cURL
session that’ll save a copy to your computer.
In the following, we have a cURL
session that’ll save an image from an Imgur URL.
<?php
// Initiate a cURL request to the image, and
// define its location.
$curl_handler = curl_init('https://i.imgur.com/ZgpqSGm.jpeg');
$image_location = 'saved_images/02_apples.png';
// Open the file for writing in binary mode
$open_image_in_binary = fopen($image_location, 'wb');
// Define where cURL should save the image.
curl_setopt($curl_handler, CURLOPT_FILE, $open_image_in_binary);
curl_setopt($curl_handler, CURLOPT_HEADER, 0);
// Lets you use this script when there is
// redirect on the server.
curl_setopt($curl_handler, CURLOPT_FOLLOWLOCATION, true);
// Auto detect encoding for the response | identity
// deflation and gzip
curl_setopt($curl_handler, CURLOPT_ENCODING, '');
// Execute the current cURL session.
curl_exec($curl_handler);
// Close the connection and
curl_close($curl_handler);
// Close the file pointer
fclose($open_image_in_binary);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
Output:
Save Image From URL Using the copy()
Function in PHP
PHP copy()
function can copy a resource from one location to another. To save the image from the URL, supply copy()
with the URL and a new location.
To ensure you have the image, check for its existence using file_exists()
.
<?php
$image_url = 'https://i.imgur.com/CcicAAl.jpeg';
$image_location = 'saved_images/03_robot.png';
// Use the copy() function to copy the image from
// its Imgur URL to a new file name in the
// saved_images folder.
$copy_image = copy($image_url, $image_location);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
Output:
Save Image From URL Using fread()
and fwrite()
in PHP
PHP fread()
will read an open file, while fwrite()
will write to an open file. Knowing this, you can open the image URL using fopen()
, read the image using fread()
, then you can save it using fwrite()
.
This sounds a bit more complex than it looks. That’s why we’ve created a function to simplify the process.
The function works in the following ways:
- Open the image in read-binary mode using
fopen()
. - Open the image location in write-binary mode using
fopen()
. - Read the image using
fread()
. - Write the image to the image location.
- Close the handle to the opened image.
- Close the handle to the image location.
We’ve used this function to save a picture of a Mac.
<?php
// Define a custom function to grab an image
// from a URL using fopen and fread.
function save_image_from_URL($source, $destination) {
$image_source = fopen($source, "rb");
$image_location = fopen($destination, "wb");
while ($read_file = fread($image_source, 8192)) {
fwrite($image_location, $read_file, 8192);
}
fclose($image_source);
fclose($image_location);
}
// Set the image URL and its new destination on
// your system
$image_url = 'https://i.imgur.com/XGSex5B.jpeg';
$image_location = 'saved_images/04_Mac.png';
// Save the image to its new destination
$save_image = save_image_from_URL($image_url, $image_location);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
Output:
Save a gzip
Image in PHP
If an image has gzip
compression, the methods discussed in this article might not work. As a workaround, we’ve modified the first example that uses file_put_contents()
and file_get_contents()
.
This time, we grab the image headers and check for gzip
encoding. If true, we decode the image before saving it; otherwise, we copy the image using PHP copy()
function.
<?php
// Set the image URL and its new location
$image_url = 'https://i.imgur.com/PpJnfpL.jpeg';
$image_location = 'saved_images/05_Application_UI.png';
// Fetch all headers from the URL
$image_headers = get_headers($image_url);
// Check if content encoding is set
$content_encoding = isset($image_headers['Content-Encoding']) ? $image_headers['Content-Encoding'] : null;
// Set gzip decode flag
$gzip_decode = ($content_encoding === 'gzip') ? true : false;
// If the image is gzipped, decode it before
// placing it in its folder.
if ($gzip_decode) {
file_put_contents($image_location, gzdecode(file_get_contents($image_url)));
} else {
copy($image_url, $image_location);
}
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn