PHP의 URL에서 이미지 저장
-
PHP에서
file_get_contents()
및file_put_contents()
를 사용하여 URL에서 이미지 저장 -
cURL
을 사용하여 URL에서 이미지 저장 -
PHP에서
copy()
기능을 사용하여 URL에서 이미지 저장 -
PHP에서
fread()
및fwrite()
를 사용하여 URL에서 이미지 저장 -
PHP에서
gzip
이미지 저장
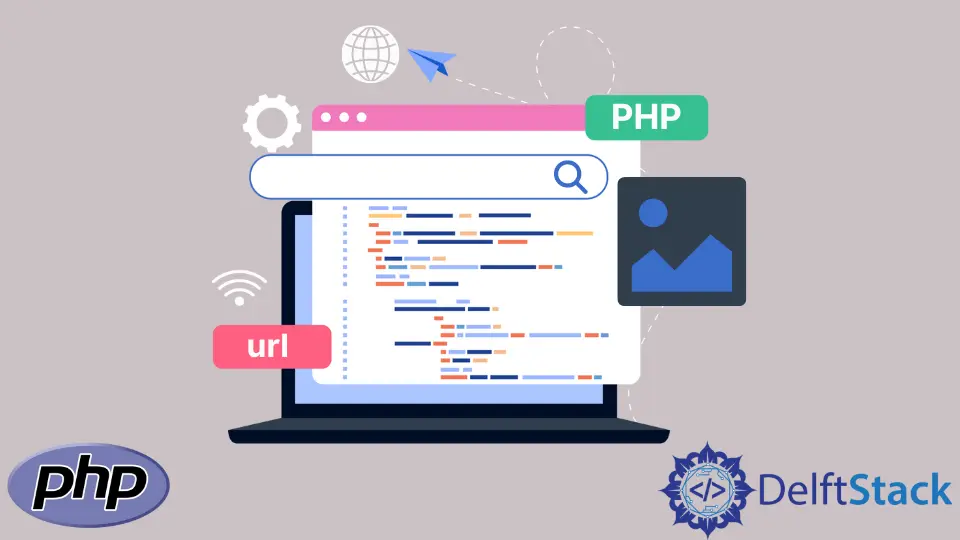
이 기사에서는 PHP의 URL에서 이미지를 저장하는 다섯 가지 방법을 설명합니다. 이러한 메서드는 file_put_contents()
, copy()
, fopen()
, fread()
, fwrite()
및 gzdecode()
와 같은 함수를 사용합니다.
PHP에서 file_get_contents()
및 file_put_contents()
를 사용하여 URL에서 이미지 저장
PHP file_get_contents()
는 파일을 문자열로 읽고 file_put_contents()
는 해당 문자열을 파일에 쓸 수 있습니다. 두 기능을 결합하면 URL에서 이미지를 저장할 수 있습니다.
먼저 file_get_contents()
를 사용하여 URL의 이미지를 문자열로 변환한 다음 file_put_contents()
를 사용하여 이 문자열을 파일에 저장합니다. 결과는 URL의 이미지 사본입니다.
다음에서는 file_get_contents()
및 file_put_contents()
를 사용하여 Imgur에서 이미지를 저장합니다. 또한 이미지의 이름을 01_computer_image.png
로 바꾸지만 다른 이름과 유효한 이미지 확장자를 사용할 수 있습니다.
<?php
// The image is from Unsplash, and we've uploaded
// it to Imgur for this article.
$image_url = 'https://i.imgur.com/NFyDQTj.jpeg';
// Define the image location. Here, the location
// is the saved_images folder.
$image_location = 'saved_images/01_computer_image.png';
// Use file_put_contents to grab the image from
// the URL and place it into the image location
// with its new name.
if (file_put_contents($image_location, file_get_contents($image_url))) {
echo "Image saved successfully";
} else {
echo "An error code, please check your code.";
}
?>
출력:
;
$image_location = 'saved_images/02_apples.png';
// Open the file for writing in binary mode
$open_image_in_binary = fopen($image_location, 'wb');
// Define where cURL should save the image.
curl_setopt($curl_handler, CURLOPT_FILE, $open_image_in_binary);
curl_setopt($curl_handler, CURLOPT_HEADER, 0);
// Lets you use this script when there is
// redirect on the server.
curl_setopt($curl_handler, CURLOPT_FOLLOWLOCATION, true);
// Auto detect encoding for the response | identity
// deflation and gzip
curl_setopt($curl_handler, CURLOPT_ENCODING, '');
// Execute the current cURL session.
curl_exec($curl_handler);
// Close the connection and
curl_close($curl_handler);
// Close the file pointer
fclose($open_image_in_binary);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
출력:
PHP에서 copy()
기능을 사용하여 URL에서 이미지 저장
PHP copy()
기능은 리소스를 한 위치에서 다른 위치로 복사할 수 있습니다. URL에서 이미지를 저장하려면 copy()
에 URL과 새 위치를 제공하십시오.
이미지가 있는지 확인하려면 file_exists()
를 사용하여 이미지가 있는지 확인하십시오.
<?php
$image_url = 'https://i.imgur.com/CcicAAl.jpeg';
$image_location = 'saved_images/03_robot.png';
// Use the copy() function to copy the image from
// its Imgur URL to a new file name in the
// saved_images folder.
$copy_image = copy($image_url, $image_location);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
출력:
로 이미지 저장
PHP에서 fread()
및 fwrite()
를 사용하여 URL에서 이미지 저장
PHP fread()
는 열린 파일을 읽고 fwrite()
는 열린 파일에 씁니다. 이를 알면 fopen()
을 사용하여 이미지 URL을 열고 fread()
를 사용하여 이미지를 읽은 다음 fwrite()
를 사용하여 저장할 수 있습니다.
이것은 보이는 것보다 조금 더 복잡하게 들립니다. 그래서 프로세스를 단순화하는 기능을 만들었습니다.
이 기능은 다음과 같은 방식으로 작동합니다.
fopen()
을 사용하여 바이너리 읽기 모드로 이미지를 엽니다.fopen()
을 사용하여 바이너리 쓰기 모드에서 이미지 위치를 엽니다.fread()
를 사용하여 이미지를 읽습니다.- 이미지 위치에 이미지를 씁니다.
- 열린 이미지의 핸들을 닫습니다.
- 핸들을 이미지 위치로 닫습니다.
이 기능을 사용하여 Mac 사진을 저장했습니다.
<?php
// Define a custom function to grab an image
// from a URL using fopen and fread.
function save_image_from_URL($source, $destination) {
$image_source = fopen($source, "rb");
$image_location = fopen($destination, "wb");
while ($read_file = fread($image_source, 8192)) {
fwrite($image_location, $read_file, 8192);
}
fclose($image_source);
fclose($image_location);
}
// Set the image URL and its new destination on
// your system
$image_url = 'https://i.imgur.com/XGSex5B.jpeg';
$image_location = 'saved_images/04_Mac.png';
// Save the image to its new destination
$save_image = save_image_from_URL($image_url, $image_location);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
출력:

및 file_get_contents()
를 사용하는 첫 번째 예제를 수정했습니다.
이번에는 이미지 헤더를 잡고 gzip
인코딩을 확인합니다. 참이면 이미지를 저장하기 전에 디코딩합니다. 그렇지 않으면 PHP copy()
기능을 사용하여 이미지를 복사합니다.
<?php
// Set the image URL and its new location
$image_url = 'https://i.imgur.com/PpJnfpL.jpeg';
$image_location = 'saved_images/05_Application_UI.png';
// Fetch all headers from the URL
$image_headers = get_headers($image_url);
// Check if content encoding is set
$content_encoding = isset($image_headers['Content-Encoding']) ? $image_headers['Content-Encoding'] : null;
// Set gzip decode flag
$gzip_decode = ($content_encoding === 'gzip') ? true : false;
// If the image is gzipped, decode it before
// placing it in its folder.
if ($gzip_decode) {
file_put_contents($image_location, gzdecode(file_get_contents($image_url)));
} else {
copy($image_url, $image_location);
}
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
출력:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn