Bild von URL in PHP speichern
-
Bild von URL speichern mit
file_get_contents()
undfile_put_contents()
in PHP -
Bild von URL mit
cURL
speichern -
Bild von URL speichern mit der
copy()
-Funktion in PHP -
Bild von URL speichern mit
fread()
undfwrite()
in PHP -
Speichern Sie ein
gzip
-Bild in PHP
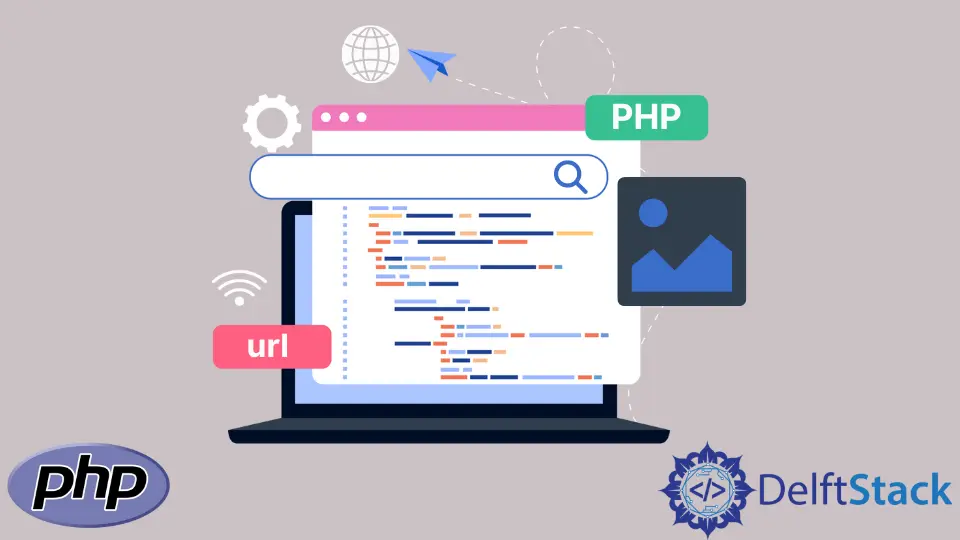
Dieser Artikel lehrt fünf Methoden, um ein Bild von einer URL in PHP zu speichern. Diese Methoden verwenden Funktionen wie file_put_contents()
, copy()
, fopen()
, fread()
, fwrite()
und gzdecode()
.
Bild von URL speichern mit file_get_contents()
und file_put_contents()
in PHP
PHP file_get_contents()
liest eine Datei in einen String, während file_put_contents()
diesen String in eine Datei schreiben kann. Wenn Sie beide Funktionen kombinieren, können Sie ein Bild von einer URL speichern.
Zuerst wandelst du das Bild aus der URL mit file_get_contents()
in einen String um und dann speicherst du diesen String mit file_put_contents()
in eine Datei. Das Ergebnis ist Ihre Kopie des Bildes aus der URL.
Im Folgenden speichern wir ein Bild aus Imgur mit file_get_contents()
und file_put_contents()
. Außerdem benennen wir das Bild in 01_computer_image.png
um, aber Sie können einen anderen Namen und eine gültige Bilderweiterung verwenden.
<?php
// The image is from Unsplash, and we've uploaded
// it to Imgur for this article.
$image_url = 'https://i.imgur.com/NFyDQTj.jpeg';
// Define the image location. Here, the location
// is the saved_images folder.
$image_location = 'saved_images/01_computer_image.png';
// Use file_put_contents to grab the image from
// the URL and place it into the image location
// with its new name.
if (file_put_contents($image_location, file_get_contents($image_url))) {
echo "Image saved successfully";
} else {
echo "An error code, please check your code.";
}
?>
Ausgang:
Bild von URL mit cURL
speichern
cURL
ist ein Befehlszeilentool zum Übertragen von Daten mithilfe von Netzwerkprotokollen. Da das Bild in der URL auf einem Server vorhanden ist, können Sie die cURL
-Sitzung starten, die eine Kopie auf Ihrem Computer speichert.
Im Folgenden haben wir eine cURL
-Sitzung, die ein Bild von einer Imgur-URL speichert.
<?php
// Initiate a cURL request to the image, and
// define its location.
$curl_handler = curl_init('https://i.imgur.com/ZgpqSGm.jpeg');
$image_location = 'saved_images/02_apples.png';
// Open the file for writing in binary mode
$open_image_in_binary = fopen($image_location, 'wb');
// Define where cURL should save the image.
curl_setopt($curl_handler, CURLOPT_FILE, $open_image_in_binary);
curl_setopt($curl_handler, CURLOPT_HEADER, 0);
// Lets you use this script when there is
// redirect on the server.
curl_setopt($curl_handler, CURLOPT_FOLLOWLOCATION, true);
// Auto detect encoding for the response | identity
// deflation and gzip
curl_setopt($curl_handler, CURLOPT_ENCODING, '');
// Execute the current cURL session.
curl_exec($curl_handler);
// Close the connection and
curl_close($curl_handler);
// Close the file pointer
fclose($open_image_in_binary);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
Ausgang:
Bild von URL speichern mit der copy()
-Funktion in PHP
Die PHP-Funktion copy()
kann eine Ressource von einem Ort an einen anderen kopieren. Um das Bild von der URL zu speichern, geben Sie copy()
mit der URL und einem neuen Speicherort an.
Um sicherzustellen, dass Sie das Bild haben, überprüfen Sie es mit file_exists()
.
<?php
$image_url = 'https://i.imgur.com/CcicAAl.jpeg';
$image_location = 'saved_images/03_robot.png';
// Use the copy() function to copy the image from
// its Imgur URL to a new file name in the
// saved_images folder.
$copy_image = copy($image_url, $image_location);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
Ausgang:
Bild von URL speichern mit fread()
und fwrite()
in PHP
PHP fread()
liest eine offene Datei, während fwrite()
in eine offene Datei schreibt. Wenn Sie dies wissen, können Sie die Bild-URL mit fopen()
öffnen, das Bild mit fread()
lesen und dann mit fwrite()
speichern.
Das hört sich etwas komplexer an, als es aussieht. Aus diesem Grund haben wir eine Funktion erstellt, um den Prozess zu vereinfachen.
Die Funktion funktioniert wie folgt:
- Öffnen Sie das Bild im Read-Binary-Modus mit
fopen()
. - Öffnen Sie den Bildspeicherort im Write-Binary-Modus mit
fopen()
. - Lesen Sie das Bild mit
fread()
. - Schreiben Sie das Bild an den Bildspeicherort.
- Schließen Sie das Handle zum geöffneten Bild.
- Schließen Sie das Handle zum Bildspeicherort.
Wir haben diese Funktion verwendet, um ein Bild von einem Mac zu speichern.
<?php
// Define a custom function to grab an image
// from a URL using fopen and fread.
function save_image_from_URL($source, $destination) {
$image_source = fopen($source, "rb");
$image_location = fopen($destination, "wb");
while ($read_file = fread($image_source, 8192)) {
fwrite($image_location, $read_file, 8192);
}
fclose($image_source);
fclose($image_location);
}
// Set the image URL and its new destination on
// your system
$image_url = 'https://i.imgur.com/XGSex5B.jpeg';
$image_location = 'saved_images/04_Mac.png';
// Save the image to its new destination
$save_image = save_image_from_URL($image_url, $image_location);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
Ausgang:
Speichern Sie ein gzip
-Bild in PHP
Wenn ein Bild eine gzip
-Komprimierung hat, funktionieren die in diesem Artikel beschriebenen Methoden möglicherweise nicht. Als Problemumgehung haben wir das erste Beispiel modifiziert, das file_put_contents()
und file_get_contents()
verwendet.
Dieses Mal schnappen wir uns die Bild-Header und prüfen auf die gzip
-Kodierung. Wenn wahr, decodieren wir das Bild, bevor wir es speichern; Andernfalls kopieren wir das Bild mit der PHP-Funktion copy()
.
<?php
// Set the image URL and its new location
$image_url = 'https://i.imgur.com/PpJnfpL.jpeg';
$image_location = 'saved_images/05_Application_UI.png';
// Fetch all headers from the URL
$image_headers = get_headers($image_url);
// Check if content encoding is set
$content_encoding = isset($image_headers['Content-Encoding']) ? $image_headers['Content-Encoding'] : null;
// Set gzip decode flag
$gzip_decode = ($content_encoding === 'gzip') ? true : false;
// If the image is gzipped, decode it before
// placing it in its folder.
if ($gzip_decode) {
file_put_contents($image_location, gzdecode(file_get_contents($image_url)));
} else {
copy($image_url, $image_location);
}
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
Ausgang:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn