Guardar imagen desde URL en PHP
-
Guardar imagen desde URL usando
file_get_contents()
yfile_put_contents()
en PHP -
Guardar imagen desde URL usando
cURL
-
Guardar imagen desde URL usando la función
copy()
en PHP -
Guardar imagen desde URL usando
fread()
yfwrite()
en PHP -
Guardar una imagen
gzip
en PHP
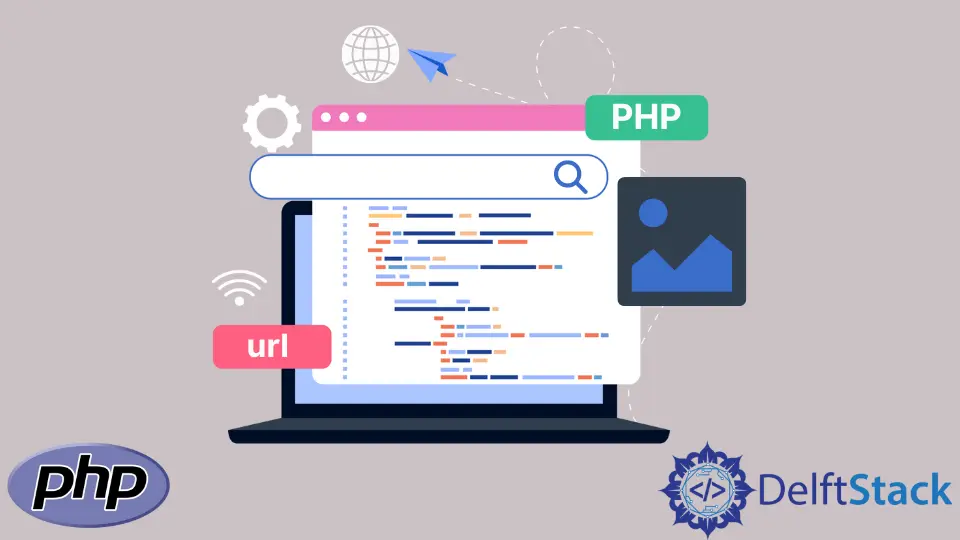
Este artículo enseña cinco métodos para guardar una imagen desde una URL en PHP. Estos métodos utilizarán funciones como file_put_contents()
, copy()
, fopen()
, fread()
, fwrite()
y gzdecode()
.
Guardar imagen desde URL usando file_get_contents()
y file_put_contents()
en PHP
PHP file_get_contents()
leerá un archivo en una cadena mientras que file_put_contents()
puede escribir esa cadena en un archivo. La combinación de ambas funciones le permitirá guardar una imagen desde una URL.
Primero, convierte la imagen de la URL en una cadena usando file_get_contents()
, y luego usa file_put_contents()
para guardar esta cadena en un archivo. El resultado será su copia de la imagen de la URL.
A continuación, guardamos una imagen de Imgur usando file_get_contents()
y file_put_contents()
. Además, cambiamos el nombre de la imagen a 01_computer_image.png
, pero puede usar otro nombre y una extensión de imagen válida.
<?php
// The image is from Unsplash, and we've uploaded
// it to Imgur for this article.
$image_url = 'https://i.imgur.com/NFyDQTj.jpeg';
// Define the image location. Here, the location
// is the saved_images folder.
$image_location = 'saved_images/01_computer_image.png';
// Use file_put_contents to grab the image from
// the URL and place it into the image location
// with its new name.
if (file_put_contents($image_location, file_get_contents($image_url))) {
echo "Image saved successfully";
} else {
echo "An error code, please check your code.";
}
?>
Producción:
Guardar imagen desde URL usando cURL
cURL
es una herramienta de línea de comandos para transferir datos usando protocolos de red. Dado que la imagen existe en la URL en un servidor, puede iniciar la sesión cURL
que guardará una copia en su computadora.
A continuación, tenemos una sesión cURL
que guardará una imagen de una URL de Imgur.
<?php
// Initiate a cURL request to the image, and
// define its location.
$curl_handler = curl_init('https://i.imgur.com/ZgpqSGm.jpeg');
$image_location = 'saved_images/02_apples.png';
// Open the file for writing in binary mode
$open_image_in_binary = fopen($image_location, 'wb');
// Define where cURL should save the image.
curl_setopt($curl_handler, CURLOPT_FILE, $open_image_in_binary);
curl_setopt($curl_handler, CURLOPT_HEADER, 0);
// Lets you use this script when there is
// redirect on the server.
curl_setopt($curl_handler, CURLOPT_FOLLOWLOCATION, true);
// Auto detect encoding for the response | identity
// deflation and gzip
curl_setopt($curl_handler, CURLOPT_ENCODING, '');
// Execute the current cURL session.
curl_exec($curl_handler);
// Close the connection and
curl_close($curl_handler);
// Close the file pointer
fclose($open_image_in_binary);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
Producción:
Guardar imagen desde URL usando la función copy()
en PHP
La función copy()
de PHP puede copiar un recurso de una ubicación a otra. Para guardar la imagen de la URL, proporcione copia()
con la URL y una nueva ubicación.
Para asegurarse de tener la imagen, verifique su existencia usando file_exists()
.
<?php
$image_url = 'https://i.imgur.com/CcicAAl.jpeg';
$image_location = 'saved_images/03_robot.png';
// Use the copy() function to copy the image from
// its Imgur URL to a new file name in the
// saved_images folder.
$copy_image = copy($image_url, $image_location);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
Producción:
Guardar imagen desde URL usando fread()
y fwrite()
en PHP
PHP fread()
leerá un archivo abierto, mientras que fwrite()
escribirá en un archivo abierto. Sabiendo esto, puede abrir la URL de la imagen usando fopen()
, leer la imagen usando fread()
, luego puede guardarla usando fwrite()
.
Esto suena un poco más complejo de lo que parece. Es por eso que hemos creado una función para simplificar el proceso.
La función funciona de las siguientes maneras:
- Abra la imagen en modo de lectura binaria usando
fopen()
. - Abra la ubicación de la imagen en modo binario de escritura usando
fopen()
. - Lee la imagen usando
fread()
. - Escriba la imagen en la ubicación de la imagen.
- Cierre el asa de la imagen abierta.
- Cierre el asa en la ubicación de la imagen.
Hemos usado esta función para guardar una imagen de una Mac.
<?php
// Define a custom function to grab an image
// from a URL using fopen and fread.
function save_image_from_URL($source, $destination) {
$image_source = fopen($source, "rb");
$image_location = fopen($destination, "wb");
while ($read_file = fread($image_source, 8192)) {
fwrite($image_location, $read_file, 8192);
}
fclose($image_source);
fclose($image_location);
}
// Set the image URL and its new destination on
// your system
$image_url = 'https://i.imgur.com/XGSex5B.jpeg';
$image_location = 'saved_images/04_Mac.png';
// Save the image to its new destination
$save_image = save_image_from_URL($image_url, $image_location);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
Producción:
Guardar una imagen gzip
en PHP
Si una imagen tiene compresión gzip
, es posible que los métodos discutidos en este artículo no funcionen. Como solución temporal, hemos modificado el primer ejemplo que usa file_put_contents()
y file_get_contents()
.
Esta vez, tomamos los encabezados de las imágenes y verificamos la codificación gzip
. Si es verdadero, decodificamos la imagen antes de guardarla; de lo contrario, copiamos la imagen usando la función copy()
de PHP.
<?php
// Set the image URL and its new location
$image_url = 'https://i.imgur.com/PpJnfpL.jpeg';
$image_location = 'saved_images/05_Application_UI.png';
// Fetch all headers from the URL
$image_headers = get_headers($image_url);
// Check if content encoding is set
$content_encoding = isset($image_headers['Content-Encoding']) ? $image_headers['Content-Encoding'] : null;
// Set gzip decode flag
$gzip_decode = ($content_encoding === 'gzip') ? true : false;
// If the image is gzipped, decode it before
// placing it in its folder.
if ($gzip_decode) {
file_put_contents($image_location, gzdecode(file_get_contents($image_url)));
} else {
copy($image_url, $image_location);
}
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
Producción:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn