PHPでURLから画像を保存
-
PHP で
file_get_contents()
とfile_put_contents()
を使用して URL から画像を保存する -
cURL
を使用して URL から画像を保存 -
PHP で
copy()
関数を使用して URL から画像を保存する -
PHP で
fread()
とfwrite()
を使用して URL から画像を保存する -
gzip
画像を PHP に保存する
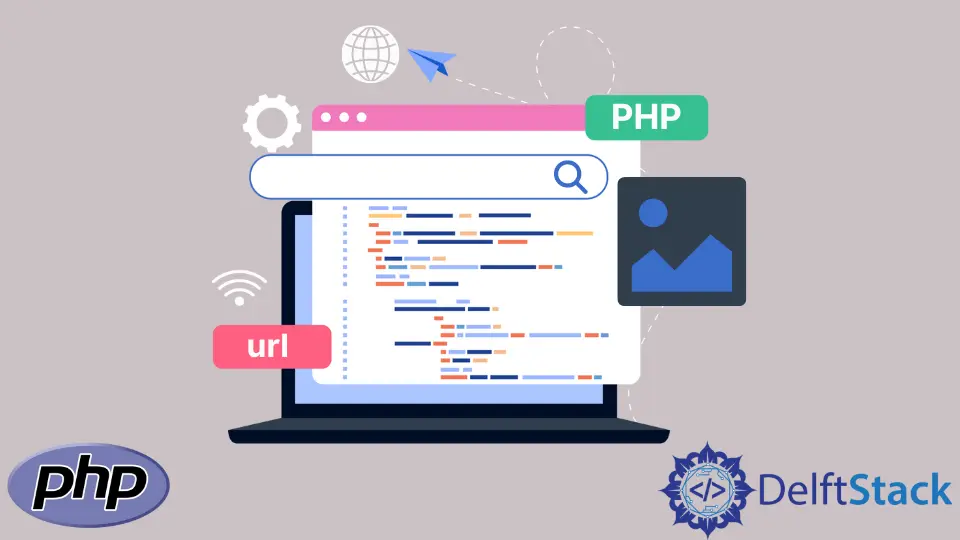
この記事では、PHP で URL から画像を保存する 5つの方法を説明します。 これらのメソッドは、file_put_contents()
、copy()
、fopen()
、fread()
、fwrite()
、gzdecode()
などの関数を使用します。
PHP で file_get_contents()
と file_put_contents()
を使用して URL から画像を保存する
PHP file_get_contents()
はファイルを文字列に読み込み、file_put_contents()
はその文字列をファイルに書き込むことができます。 両方の機能を組み合わせると、URL から画像を保存できます。
最初に、file_get_contents()
を使用して URL から画像を文字列に変換し、次に file_put_contents()
を使用してこの文字列をファイルに保存します。 結果は、URL からの画像のコピーになります。
以下では、file_get_contents()
と file_put_contents()
を使用して Imgur から画像を保存します。 また、画像の名前を 01_computer_image.png
に変更しますが、別の名前と有効な画像拡張子を使用できます。
<?php
// The image is from Unsplash, and we've uploaded
// it to Imgur for this article.
$image_url = 'https://i.imgur.com/NFyDQTj.jpeg';
// Define the image location. Here, the location
// is the saved_images folder.
$image_location = 'saved_images/01_computer_image.png';
// Use file_put_contents to grab the image from
// the URL and place it into the image location
// with its new name.
if (file_put_contents($image_location, file_get_contents($image_url))) {
echo "Image saved successfully";
} else {
echo "An error code, please check your code.";
}
?>
出力:
cURL
を使用して URL から画像を保存
cURL は、ネットワーク プロトコルを使用してデータを転送するためのコマンドライン ツールです。 イメージはサーバー上の URL に存在するため、コピーをコンピューターに保存するcURL
セッションを開始できます。
以下では、Imgur URL から画像を保存する cURL
セッションがあります。
<?php
// Initiate a cURL request to the image, and
// define its location.
$curl_handler = curl_init('https://i.imgur.com/ZgpqSGm.jpeg');
$image_location = 'saved_images/02_apples.png';
// Open the file for writing in binary mode
$open_image_in_binary = fopen($image_location, 'wb');
// Define where cURL should save the image.
curl_setopt($curl_handler, CURLOPT_FILE, $open_image_in_binary);
curl_setopt($curl_handler, CURLOPT_HEADER, 0);
// Lets you use this script when there is
// redirect on the server.
curl_setopt($curl_handler, CURLOPT_FOLLOWLOCATION, true);
// Auto detect encoding for the response | identity
// deflation and gzip
curl_setopt($curl_handler, CURLOPT_ENCODING, '');
// Execute the current cURL session.
curl_exec($curl_handler);
// Close the connection and
curl_close($curl_handler);
// Close the file pointer
fclose($open_image_in_binary);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
出力:
PHP で copy()
関数を使用して URL から画像を保存する
PHP の copy()
関数は、ある場所から別の場所にリソースをコピーできます。 URL から画像を保存するには、copy()
に URL と新しい場所を指定します。
画像があることを確認するには、file_exists()
を使用してその存在を確認します。
<?php
$image_url = 'https://i.imgur.com/CcicAAl.jpeg';
$image_location = 'saved_images/03_robot.png';
// Use the copy() function to copy the image from
// its Imgur URL to a new file name in the
// saved_images folder.
$copy_image = copy($image_url, $image_location);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
出力:
PHP で fread()
と fwrite()
を使用して URL から画像を保存する
PHP fread()
は開いているファイルを読み取り、fwrite()
は開いているファイルに書き込みます。 これを知っていれば、fopen()
を使用して画像の URL を開き、fread()
を使用して画像を読み取り、fwrite()
を使用して保存できます。
これは、見た目よりも少し複雑に聞こえます。 そのため、プロセスを簡素化する関数を作成しました。
この機能は次のように機能します。
fopen()
を使用して、読み取りバイナリ モードでイメージを開きます。fopen()
を使用して、書き込みバイナリ モードでイメージの場所を開きます。fread()
を使用して画像を読み取ります。- イメージの場所にイメージを書き込みます。
- 開いた画像のハンドルを閉じます。
- ハンドルを画像位置まで閉じます。
この関数を使用して、Mac の写真を保存しました。
<?php
// Define a custom function to grab an image
// from a URL using fopen and fread.
function save_image_from_URL($source, $destination) {
$image_source = fopen($source, "rb");
$image_location = fopen($destination, "wb");
while ($read_file = fread($image_source, 8192)) {
fwrite($image_location, $read_file, 8192);
}
fclose($image_source);
fclose($image_location);
}
// Set the image URL and its new destination on
// your system
$image_url = 'https://i.imgur.com/XGSex5B.jpeg';
$image_location = 'saved_images/04_Mac.png';
// Save the image to its new destination
$save_image = save_image_from_URL($image_url, $image_location);
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
出力:
gzip
画像を PHP に保存する
画像が gzip
圧縮されている場合、この記事で説明した方法は機能しない可能性があります。 回避策として、file_put_contents()
と file_get_contents()
を使用する最初の例を修正しました。
今回は、画像ヘッダーを取得し、gzip
エンコーディングを確認します。 true の場合、画像を保存する前にデコードします。 それ以外の場合は、PHP の copy()
関数を使用して画像をコピーします。
<?php
// Set the image URL and its new location
$image_url = 'https://i.imgur.com/PpJnfpL.jpeg';
$image_location = 'saved_images/05_Application_UI.png';
// Fetch all headers from the URL
$image_headers = get_headers($image_url);
// Check if content encoding is set
$content_encoding = isset($image_headers['Content-Encoding']) ? $image_headers['Content-Encoding'] : null;
// Set gzip decode flag
$gzip_decode = ($content_encoding === 'gzip') ? true : false;
// If the image is gzipped, decode it before
// placing it in its folder.
if ($gzip_decode) {
file_put_contents($image_location, gzdecode(file_get_contents($image_url)));
} else {
copy($image_url, $image_location);
}
// Confirm the new image exists in the saved_images
// folder.
if (file_exists($image_location)) {
echo "Image saved successfully";
} else {
echo "An error occurred. Please check your code";
}
?>
出力:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn