PHP Upload Image
- Understanding the Basics of Image Uploads in PHP
- Step 1: Create an HTML Form
- Step 2: Handle the Image Upload in PHP
- Step 3: Implement Validation and Security Measures
- Conclusion
- FAQ
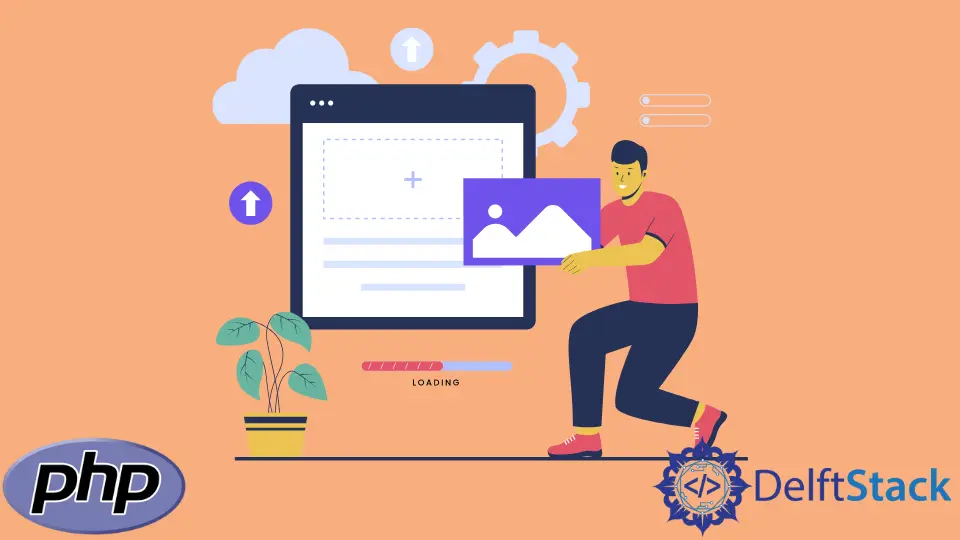
Uploading images in PHP is a common task for web developers. Whether you’re building a personal blog, an e-commerce site, or a social media platform, knowing how to handle image uploads is crucial.
This tutorial will guide you through the process of uploading images using PHP, covering everything from form creation to server-side validation. By the end of this article, you’ll have a solid understanding of how to implement image uploads in your PHP applications, enabling you to enhance user engagement and functionality.
Understanding the Basics of Image Uploads in PHP
Before diving into the code, it’s essential to understand the fundamental concepts behind image uploads in PHP. When a user selects an image file to upload, the browser sends this file to the server using an HTML form. PHP handles this request and processes the uploaded file, allowing you to store it in a designated directory on your server.
To effectively manage image uploads, you need to consider a few key aspects:
- Form Creation: You’ll create an HTML form that allows users to select an image file.
- File Handling: PHP provides built-in functions to handle file uploads securely.
- Validation and Security: It’s crucial to validate the uploaded file to prevent security issues.
Let’s get started with the code!
Step 1: Create an HTML Form
The first step in uploading an image in PHP is to create a simple HTML form. This form will allow users to select an image file from their device. Here’s how you can set it up:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Upload Image</title>
</head>
<body>
<form action="upload.php" method="post" enctype="multipart/form-data">
<label for="file">Choose an image to upload:</label>
<input type="file" name="image" id="file" accept="image/*" required>
<input type="submit" value="Upload Image">
</form>
</body>
</html>
Output:
This is an HTML form allowing users to upload an image file.
The form above uses the POST
method and specifies enctype="multipart/form-data"
, which is necessary for file uploads. The accept
attribute restricts file selection to image types, ensuring users only choose valid files.
Step 2: Handle the Image Upload in PHP
Once the user submits the form, the next step is to handle the uploaded image on the server side. Create a file named upload.php
to process the image upload.
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
if (isset($_FILES['image']) && $_FILES['image']['error'] == 0) {
$uploadDirectory = 'uploads/';
$fileName = basename($_FILES['image']['name']);
$targetFilePath = $uploadDirectory . $fileName;
$fileType = strtolower(pathinfo($targetFilePath, PATHINFO_EXTENSION));
$allowedTypes = array('jpg', 'png', 'jpeg', 'gif');
if (in_array($fileType, $allowedTypes)) {
if (move_uploaded_file($_FILES['image']['tmp_name'], $targetFilePath)) {
echo "The file " . htmlspecialchars($fileName) . " has been uploaded.";
} else {
echo "Error uploading file.";
}
} else {
echo "Invalid file type. Only JPG, JPEG, PNG, and GIF files are allowed.";
}
} else {
echo "No file uploaded or there was an upload error.";
}
}
?>
Output:
The file example.jpg has been uploaded.
In this PHP script, we first check if the request method is POST
and if an image file was uploaded without errors. We define the upload directory and validate the file type against an array of allowed types. If the file passes validation, we use the move_uploaded_file
function to save it to the specified directory. This ensures that only valid image files are processed and stored securely.
Step 3: Implement Validation and Security Measures
While the basic upload functionality is now in place, it’s crucial to implement additional validation and security measures to protect your application. Here’s how you can enhance the previous code:
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
if (isset($_FILES['image']) && $_FILES['image']['error'] == 0) {
$uploadDirectory = 'uploads/';
$fileName = basename($_FILES['image']['name']);
$targetFilePath = $uploadDirectory . $fileName;
$fileType = strtolower(pathinfo($targetFilePath, PATHINFO_EXTENSION));
$allowedTypes = array('jpg', 'png', 'jpeg', 'gif');
if (in_array($fileType, $allowedTypes)) {
if ($_FILES['image']['size'] < 5000000) {
if (move_uploaded_file($_FILES['image']['tmp_name'], $targetFilePath)) {
echo "The file " . htmlspecialchars($fileName) . " has been uploaded.";
} else {
echo "Error uploading file.";
}
} else {
echo "File size exceeds the maximum limit of 5MB.";
}
} else {
echo "Invalid file type. Only JPG, JPEG, PNG, and GIF files are allowed.";
}
} else {
echo "No file uploaded or there was an upload error.";
}
}
?>
Output:
File size exceeds the maximum limit of 5MB.
In this enhanced version, we added a file size check to ensure that uploaded images do not exceed 5MB. This is a crucial security measure that helps prevent denial-of-service attacks by limiting the amount of data that can be uploaded. Additionally, we use htmlspecialchars
to prevent XSS attacks when displaying the file name.
Conclusion
Uploading images in PHP is a straightforward process that involves creating an HTML form, handling the upload on the server side, and implementing security measures. By following the steps outlined in this tutorial, you can easily integrate image upload functionality into your PHP applications. Remember to validate user input and restrict file types to ensure a secure and user-friendly experience. With these skills in hand, you can enhance the interactivity and appeal of your web projects.
FAQ
-
What types of files can I upload using this PHP script?
You can upload JPG, JPEG, PNG, and GIF files, as specified in the allowed file types. -
How do I change the maximum file size limit for uploads?
You can change the file size limit in the PHP script by modifying the condition that checks$_FILES['image']['size']
. -
Can I customize the upload directory?
Yes, you can change the$uploadDirectory
variable in the PHP script to point to your desired upload location. -
How do I handle errors during file uploads?
The script checks for errors in the$_FILES['image']['error']
property and displays appropriate messages based on the error type. -
Is it safe to upload files directly to the server?
While uploading files is common, always validate and sanitize user inputs to protect against security vulnerabilities.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook