How to Download File Forcely in PHP
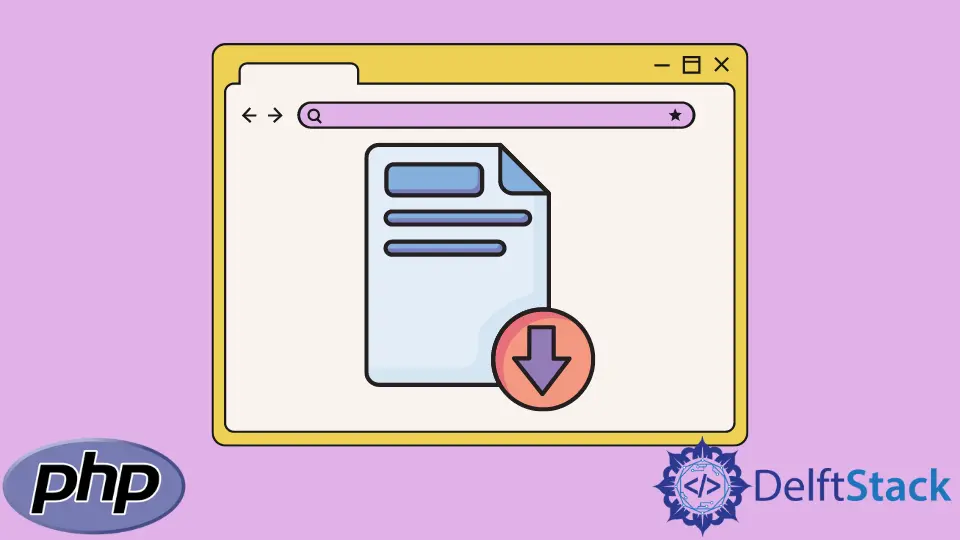
In this tutorial, we learn how to force download files in PHP.
Force a File Download in PHP
This process requires two steps:
- Create the PHP file for handling that file which you want to protect.
- Add a relevance that PHP file inside the HTML of the page during which it appears.
After uploading a file to the server, create a PHP document in a text editor. For example, if you want to force sample.pdf to download instead of viewing it online, create a script like this.
<?php
header("Content-disposition: attachment; filename=sample.pdf");
header("Content-type: application/pdf");
readfile("sample.pdf");
?>
The content-type reference in PHP is important: it is the MIME type of the file that you are protecting. For example, if you saved an MP3 file instead, you would need to replace the application/pdf with an audio MPEG.
Download Files With PHP
Usually, you don’t need to use a server-side scripting language like PHP to download images, zip files, PDF documents, exe files, etc. If this type of file is stored in an accessible public folder, you can simply create a hyperlink pointing to that file, and each time a user clicks the link, the browser will automatically download that file.
<a href="downloads/test.zip">Download Zip file</a>
<a href="downloads/masters.pdf">Download PDF file</a>
<a href="downloads/sample.jpg">Download Image file</a>
<a href="downloads/setup.exe">Download EXE file</a>
the readfile()
Function
You can force images or other file types to be downloaded directly to the user’s hard drive by using PHP readfile()
function. Here we are creating a simple image gallery that will allow users to download the image files from the browser with a single click of the mouse. Let’s create a file called “image-gallery.php” and put the following code in it.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple Image Gallery</title>
<style type="text/css">
.img-box{
display: inline-block;
text-align: center;
margin: 0 15px;
}
</style>
</head>
<body>
<?php
// Array containing sample image file names
$images = array("hello.jpg", "resampled1.jpg");
// Loop through array to create image gallery
foreach($images as $image){
echo '<div class="img-box">';
echo '<img src="/examples/images/' . $image . '" width="200" alt="' . pathinfo($image, PATHINFO_FILENAME) .'">';
echo '<p><a href="/examples/php/download.php?file=' . urlencode($image) . '">Download</a></p>';
echo '</div>';
}
?>
</body>
</html>
If you look closely at the example program above, you can find the download link that leads to a file; the URL also includes the image file name as a query string. We also used PHP’s urlencode()
function to encode the image filenames so that they can be safely passed as URL parameters, as the filenames can contain unsafe URL characters.
Here’s the complete code of "download.php"
file, which forces image download.
<?php
if(!empty($_GET['file'])){
$fileName = basename($_GET['file']);
$filePath = 'files.txt/'.$fileName;
if(!empty($fileName) && file_exists($filePath)){
// Define headers
header("Cache-Control: public");
header("Content-Description: File Transfer");
header("Content-Disposition: attachment; filename=$fileName");
header("Content-Type: application/zip");
header("Content-Transfer-Encoding: binary");
// Read the file
readfile($filePath);
exit;
}else{
echo 'The file does not exist.';
}
}
} else {
die("Invalid file name!");
}
}
?>
Likewise, you can force download other file formats like Word Doc, PDF files, etc. The regular expression in the example above simply does not allow files with names beginning or ending with a period (.
). For example, it allows filenames like hello.jpeg
or resampled1.jpeg
, myscript.min.js
, but does not allow hello.jpeg.
or .resampled.Jpeg.
.