PHP에서 강제로 파일 다운로드
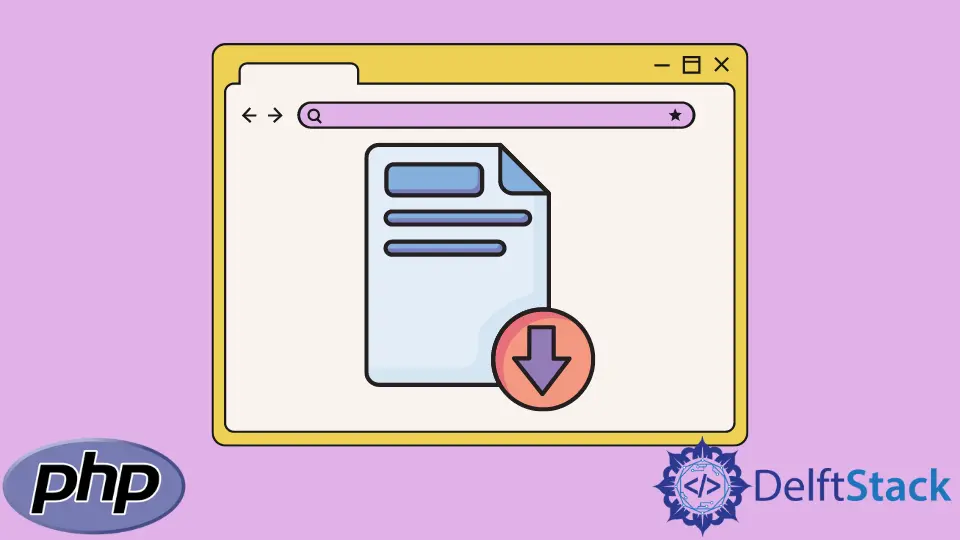
이 튜토리얼에서는 PHP에서 파일을 강제로 다운로드하는 방법을 배웁니다.
PHP에서 강제로 파일 다운로드
이 프로세스에는 두 단계가 필요합니다.
- 보호하려는 파일을 처리하기 위한 PHP 파일을 만듭니다.
- 해당 PHP 파일이 나타나는 동안 페이지의 HTML 내부에 관련성을 추가합니다.
서버에 파일을 업로드한 후 텍스트 편집기에서 PHP 문서를 생성합니다. 예를 들어 sample.pdf를 온라인에서 보는 대신 다운로드하도록 하려면 다음과 같은 스크립트를 만드십시오.
<?php
header("Content-disposition: attachment; filename=sample.pdf");
header("Content-type: application/pdf");
readfile("sample.pdf");
?>
PHP에서 콘텐츠 유형 참조는 중요합니다. 보호하는 파일의 MIME 유형입니다. 예를 들어 MP3 파일을 대신 저장했다면 application/pdf를 오디오 MPEG로 바꿔야 합니다.
PHP로 파일 다운로드
일반적으로 PHP와 같은 서버 측 스크립팅 언어를 사용하여 이미지, zip 파일, PDF 문서, exe 파일 등을 다운로드할 필요가 없습니다. 이러한 유형의 파일이 액세스 가능한 공용 폴더에 저장되어 있으면 간단히 해당 파일을 가리키는 하이퍼링크이며 사용자가 링크를 클릭할 때마다 브라우저는 자동으로 해당 파일을 다운로드합니다.
<a href="downloads/test.zip">Download Zip file</a>
<a href="downloads/masters.pdf">Download PDF file</a>
<a href="downloads/sample.jpg">Download Image file</a>
<a href="downloads/setup.exe">Download EXE file</a>
readfile()
함수
PHP readfile()
기능을 사용하여 이미지 또는 기타 파일 유형을 사용자의 하드 드라이브에 직접 다운로드하도록 할 수 있습니다. 여기에서는 사용자가 마우스를 한 번만 클릭하여 브라우저에서 이미지 파일을 다운로드할 수 있는 간단한 이미지 갤러리를 만들고 있습니다. “image-gallery.php"라는 파일을 만들고 그 안에 다음 코드를 넣어봅시다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple Image Gallery</title>
<style type="text/css">
.img-box{
display: inline-block;
text-align: center;
margin: 0 15px;
}
</style>
</head>
<body>
<?php
// Array containing sample image file names
$images = array("hello.jpg", "resampled1.jpg");
// Loop through array to create image gallery
foreach($images as $image){
echo '<div class="img-box">';
echo '<img src="/examples/images/' . $image . '" width="200" alt="' . pathinfo($image, PATHINFO_FILENAME) .'">';
echo '<p><a href="/examples/php/download.php?file=' . urlencode($image) . '">Download</a></p>';
echo '</div>';
}
?>
</body>
</html>
위의 예제 프로그램을 자세히 살펴보면 파일로 연결되는 다운로드 링크를 찾을 수 있습니다. URL에는 이미지 파일 이름도 쿼리 문자열로 포함됩니다. 또한 PHP의 urlencode()
함수를 사용하여 이미지 파일 이름을 인코딩하여 파일 이름에 안전하지 않은 URL 문자가 포함될 수 있으므로 URL 매개변수로 안전하게 전달할 수 있습니다.
다음은 이미지 다운로드를 강제하는 "download.php"
파일의 전체 코드입니다.
<?php
if(!empty($_GET['file'])){
$fileName = basename($_GET['file']);
$filePath = 'files.txt/'.$fileName;
if(!empty($fileName) && file_exists($filePath)){
// Define headers
header("Cache-Control: public");
header("Content-Description: File Transfer");
header("Content-Disposition: attachment; filename=$fileName");
header("Content-Type: application/zip");
header("Content-Transfer-Encoding: binary");
// Read the file
readfile($filePath);
exit;
}else{
echo 'The file does not exist.';
}
}
} else {
die("Invalid file name!");
}
}
?>
마찬가지로 Word Doc, PDF 파일 등과 같은 다른 파일 형식을 강제로 다운로드할 수 있습니다. 위의 예에서 정규식은 이름이 마침표(.
)로 시작하거나 끝나는 파일을 허용하지 않습니다. 예를 들어 hello.jpeg
또는 resampled1.jpeg
, myscript.min.js
와 같은 파일 이름은 허용하지만 hello.jpeg.
또는 .resampled.Jpeg.
는 허용하지 않습니다.