在 PHP 中強制下載檔案
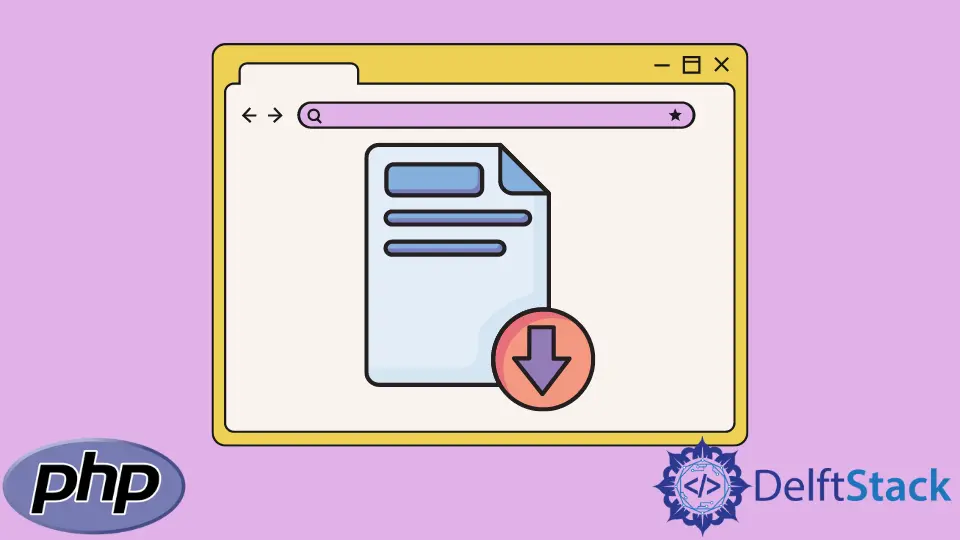
在本教程中,我們將學習如何在 PHP 中強制下載檔案。
在 PHP 中強制下載檔案
這個過程需要兩個步驟:
- 建立用於處理要保護的檔案的 PHP 檔案。
- 在它出現的頁面的 HTML 中新增一個相關的 PHP 檔案。
將檔案上傳到伺服器後,在文字編輯器中建立一個 PHP 文件。例如,如果你想強制下載 sample.pdf 而不是線上檢視,請建立如下指令碼。
<?php
header("Content-disposition: attachment; filename=sample.pdf");
header("Content-type: application/pdf");
readfile("sample.pdf");
?>
PHP 中的內容型別引用很重要:它是你要保護的檔案的 MIME 型別。例如,如果你儲存的是 MP3 檔案,則需要用音訊 MPEG 替換應用程式/pdf。
使用 PHP 下載檔案
通常,你不需要使用 PHP 等伺服器端指令碼語言來下載影象、zip 檔案、PDF 文件、exe 檔案等。如果此類檔案儲存在可訪問的公共資料夾中,你只需建立一個指向該檔案的超連結,每次使用者單擊該連結時,瀏覽器都會自動下載該檔案。
<a href="downloads/test.zip">Download Zip file</a>
<a href="downloads/masters.pdf">Download PDF file</a>
<a href="downloads/sample.jpg">Download Image file</a>
<a href="downloads/setup.exe">Download EXE file</a>
readfile()
函式
你可以使用 PHP readfile()
函式強制將影象或其他檔案型別直接下載到使用者的硬碟驅動器。在這裡,我們建立了一個簡單的圖片庫,允許使用者通過單擊滑鼠從瀏覽器下載影象檔案。讓我們建立一個名為 image-gallery.php
的檔案並將以下程式碼放入其中。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple Image Gallery</title>
<style type="text/css">
.img-box{
display: inline-block;
text-align: center;
margin: 0 15px;
}
</style>
</head>
<body>
<?php
// Array containing sample image file names
$images = array("hello.jpg", "resampled1.jpg");
// Loop through array to create image gallery
foreach($images as $image){
echo '<div class="img-box">';
echo '<img src="/examples/images/' . $image . '" width="200" alt="' . pathinfo($image, PATHINFO_FILENAME) .'">';
echo '<p><a href="/examples/php/download.php?file=' . urlencode($image) . '">Download</a></p>';
echo '</div>';
}
?>
</body>
</html>
如果你仔細檢視上面的示例程式,你可以找到指向檔案的下載連結; URL 還包括影象檔名作為查詢字串。我們還使用 PHP 的 urlencode()
函式對影象檔名進行編碼,以便它們可以安全地作為 URL 引數傳遞,因為檔名可能包含不安全的 URL 字元。
這是"download.php"
檔案的完整程式碼,它強制下載影象。
<?php
if(!empty($_GET['file'])){
$fileName = basename($_GET['file']);
$filePath = 'files.txt/'.$fileName;
if(!empty($fileName) && file_exists($filePath)){
// Define headers
header("Cache-Control: public");
header("Content-Description: File Transfer");
header("Content-Disposition: attachment; filename=$fileName");
header("Content-Type: application/zip");
header("Content-Transfer-Encoding: binary");
// Read the file
readfile($filePath);
exit;
}else{
echo 'The file does not exist.';
}
}
} else {
die("Invalid file name!");
}
}
?>
同樣,你可以強制下載其他檔案格式,如 Word Doc、PDF 檔案等。上例中的正規表示式不允許名稱以句點 .
開頭或結尾的檔案。例如,它允許像 hello.jpeg
或 resampled1.jpeg
、myscript.min.js
這樣的檔名,但不允許 hello.jpeg.
或 .resampled.Jpeg.
。