Difference Between the == and the === Comparison Operators in PHP
-
Compare an Integer Value and a Floating Point Value With the Double (
==
) and Triple (===
) Equals Comparision Operator in PHP -
Compare an Integer Value and a Boolean Value With the Double (
==
) and Triple (===
) Equals Comparision Operator in PHP - Compare an Integer Value and a String Value With the Double and Triple Equals Comparision Operator
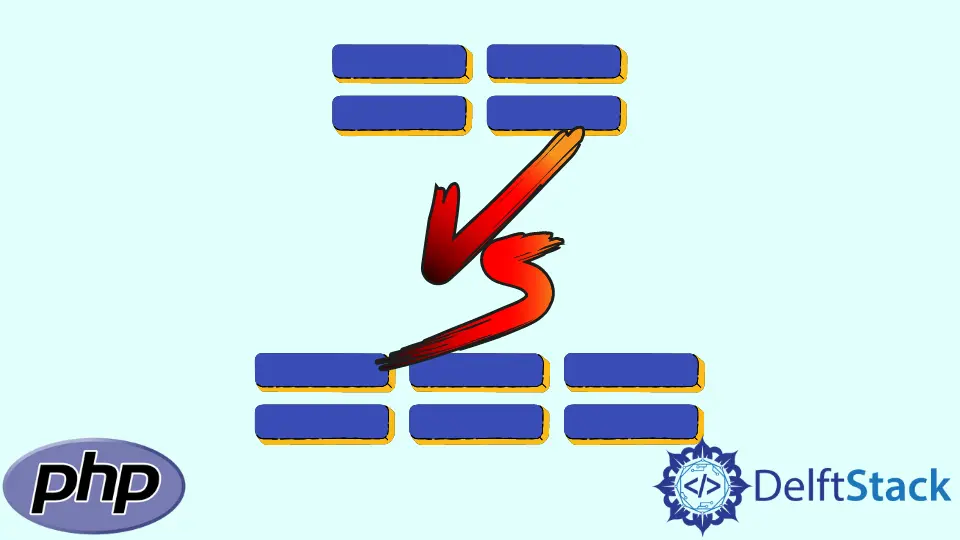
This article will introduce the difference between the double equals ==
and the triple equals ===
comparison operators in PHP. The significant difference is the double equals comparison operator compares the value of the two operands. In contrast, the triple equals Comparision operator compares the value and the type of the operands.
We will introduce a method to differentiate the double equals and the triple equals comparison operator by comparing an integer and a floating-point value. We will use the ternary operator as the conditional statement.
The second method compares the double equals and the triple equals comparison operator with an integer and a boolean value. We will also use the ternary operator in this method.
We will also demonstrate the comparison of an integer value and a string using double equals and the triple equals comparison operator. We will use the var_dump()
function to dump the comparison in this method.
Compare an Integer Value and a Floating Point Value With the Double (==
) and Triple (===
) Equals Comparision Operator in PHP
We can compare the two same values of different types using the comparison operators. This method compares an integer and a floating point that have the same value. We can use the ternary operator to compare the operands and the var_dump()
function to dump their boolean values.
For example, create a variable $a
and store the integer value 5
in it. Store a floating-point value 5.0
in another variable $b
. Compare the two variables with the triple comparison operator using the ternary operator. The truthy value returns true
, and the falsy value returns false
in the ternary operator. Store the operation in the $c
variable. Similarly, compare the two variables with double equals operator with the ternary operator and store the operation in the $d
variable. Use the var_dump()
functions in both the $c
and $d
variables.
The output of the code example returns the false
value for the triple equals operator and the true
value for the double equals operator. The value 5
is of integer type, and the value 5.0
is of float type, although the value is the same. Therefore, the triple equals operator returns a false value, and the double operator returns a truthy value.
Example Code:
#php 7.x
<?php
$a = 5;
$b = 5.0;
$c = $a===$b ? true : false;
$d = $a==$b ? true : false;
var_dump($c);
var_dump($d);
?>
Output:
bool(false) bool(true)
Compare an Integer Value and a Boolean Value With the Double (==
) and Triple (===
) Equals Comparision Operator in PHP
This method compares an integer value 1
and the boolean value true
. The integer value 1
denotes the truth value. We can use the ternary operator as in the first method. For example, store the integer value 1
in a variable $a
. Create another variable $b
and store a boolean value true
in it. Use the ternary operator to compare these two variables as in the first method. Compare the variables with both double equals and triple equals comparison operator. Store the results in the variables $c
and $d
and use the var_dump()
functions to dump these variables.
The $c
variable returns the falsy value, and the $d
value returns the truthy value. As the triple equals operator compares the type of the operands, it is obvious that the type of 1
and true
does not match. But, the value 1
is the truthy value, so its value is the same as the true
boolean value.
Example Code:
#php 7.x
<?php
$a = 1;
$b = true;
$c = $a===$b ? true : false;
$d = $a==$b ? true : false;
var_dump($c);
var_dump($d);
?>
Output:
bool(false) bool(true)
Compare an Integer Value and a String Value With the Double and Triple Equals Comparision Operator
We can compare the operands directly in the var_dump()
function to see the result of the comparison. This method is the short-hand method of the methods using the ternary operators. The illustration below compares an integer value and a string value using the triple equals and the double equals comparison operator.
For example, create a variable $a
and store the integer value 5
in it. Store a string value '5'
in another variable $b
. Compare these two variables first using the triple equals comparison operator and then using the double equals operator. Wrap these comparisons inside the var_dump()
functions.
The first comparison results in a false value. The second comparison returns the truth value. The type of the two variables does not match, so it results in a false value. The string value 5
gets typecasted to an integer value, and the value for both variables will be the same. However, the type of the variables does not change.
Example Code:
#php 7.x
<?php
$a = 5;
$b = '5';
var_dump($a===$b);
var_dump($a==$b);
?>
Output:
bool(false) bool(true)
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn