How to Get the Errors to Display in PHP
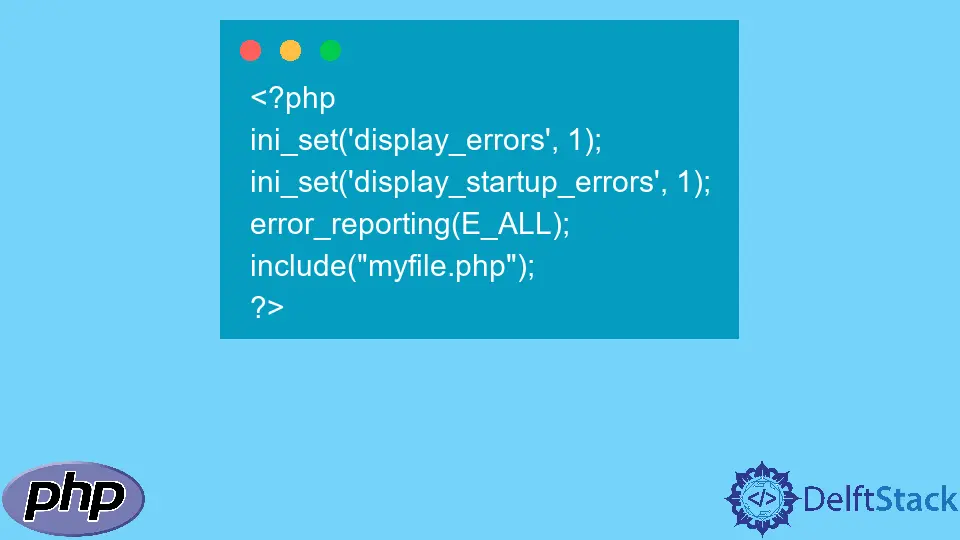
In this article, we will introduce a method to get the errors to display in PHP.
- Using
ini_set()
anderror_reporting()
functions
Use ini_set()
and error_reporting()
Function to Get the Errors to Display in PHP
To get the errors to display in PHP we can use ini_set()
and error_reporting
functions. These functions display the errors contained in a PHP file. The correct syntax to use these two functions is as follows.
ini_set($configurationName, $value);
The built-in function ini_set()
sets a new value for the given configuration. The configuration configures the PHP setup. There are several configurations available for this function. This function accepts two parameters. The details of its parameters are as follows.
Parameters | Description | |
---|---|---|
$configurationName |
mandatory | It specifies the configuration whose value we wish to update. It should be a string. The list of the configurations for this function is available here. |
$value |
mandatory | It is the value for the chosen configuration. |
It returns the previous value on success and false otherwise.
The built-in function error_reporting()
selects which error or errors will be reported.
error_reporting($errorLevel);
The error_reporting()
function accepts only one parameter. It returns the previous error if no new error is passed. The detail of its parameter is as follows.
Parameters | Description | |
---|---|---|
$errorLevel |
mandatory | It is the name of the error we wish to report. It can be a number or a named constant. A named constant makes reporting more clear for the user rather than using a number. You can check these here. |
The program below specifies how to use these two functions in displaying errors.
<?php
ini_set('display_errors', 1);
ini_set('display_startup_errors', 1);
error_reporting(E_ALL);
include("myfile.php");
?>
The display_errors
configuration decides whether the errors will be displayed to the user or not. The display_startup_errors
configuration is used to look for the errors during PHP’s startup. And E_ALL
is a named constant used to specify the error level. It means that all the errors and warnings are included.
Output:
The above code does not display the parse errors. To display the parse errors, you will have to modify php.ini using the following line.
display_errors = on
For example, for the code given below, if display_errors
is on in php.ini
then the output will be an error because there is a semi-colon missing after $j <= 5
.
<?php
for($j = 0; $j <= 5 $j++)
{
echo $j;
}
?>
Output: