How to Log Errors in PHP
-
Use
ini_set()
to Enable PHP Error Logging -
Use
error_log()
to Log Errors in PHP -
Use
error_reporting()
to Log Errors in PHP in the Browser
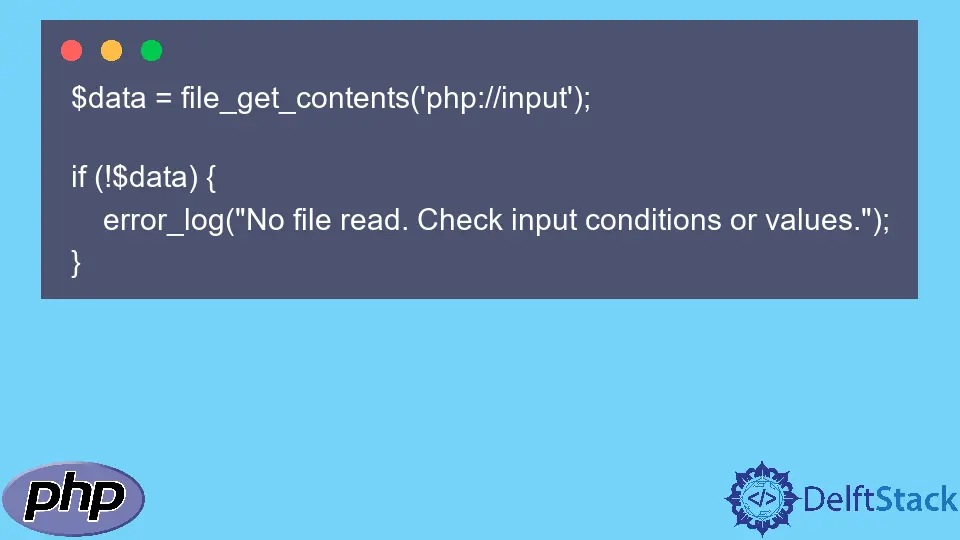
Logging errors are essential when we develop any application, including PHP applications. It is, therefore, important to know how to log errors in PHP and not rely on print_r()
and var_dump()
to know when something has gone wrong.
This article will discuss all the functions we need to use to enable and work with error logging in PHP.
Use ini_set()
to Enable PHP Error Logging
Before anything, open the php.ini
file and check if display_errors
is set to On
, just like in the image below.
Additionally, within the php.ini
file, you need to check for error_reporting
conditions. If your version is PHP 8.1, you might see error_reporting = E_ALL & ~E_DEPRECATED & ~E_STRICT
in your php.ini
file.
However, to be safe, add the following lines.
error_reporting = E_ALL & ~E_NOTICE
error_reporting = E_ALL & ~E_NOTICE | E_STRICT
error_reporting = E_COMPILE_ERROR|E_RECOVERABLE_ERROR|E_ER… _ERROR
error_reporting = E_ALL & ~E_NOTICE
To allow you to log errors properly, you need to make use of the ini_set()
function to enable error logging. This function allows us to set the value of a configuration option, such as error logging options.
We can configure PHP error logging within our codebase with an option and a value. The code snippet below shows us the options and the values needed.
ini_set("log_errors", 1);
ini_set("error_log", "/tmp/php-error.log");
If we need to log errors within our PHP scripts, we will need to include them within that script. To maintain the DRY
principle, we should create a PHP file that holds the above code and require it in every PHP we need.
require_once('error.php');
Use error_log()
to Log Errors in PHP
Now that we have configured our PHP environment to enable PHP error logging, we can use the all-important error_log()
function.
With this function, we reduce using the print_r()
and var_dump()
function for debugging (especially in the production environment) and create special error templates to let us know something is not working right.
To show how error_log()
works, we can use the function to tell us a read file input operation has failed. In the code snippet, we read the PHP input using the file_get_contents
function, and if $data
is empty (which will set true
on a NOT
condition), the No file read.
error statement will be logged.
$data = file_get_contents('php://input');
if (!$data) {
error_log("No file read. Check input conditions or values.");
}
The output of the code, if there is no input for the file_get_contents()
function, is below.
No file read. Check input conditions or values.
As you can see the error_log()
function takes a message
argument. Aside from that argument, it takes a message_type
, destination
and additional_headers
argument.
Different log types define the integer value you will parse to the error_log
function; you can check the PHP documentation for more details about that.
For example, if you want to send the error message to the PHP system logger, you use 0
as the message type.
Also, you can save to a file destination using the message type 3
, as in the code snippet below.
error_log("No file read. Check input conditions or values.", 3, "/my-errors.log");
Use error_reporting()
to Log Errors in PHP in the Browser
Aside from the ini_set()
function, we can set which PHP errors are reported. The error_reporting()
function helps us with that.
Remember the error PHP file we created to be required by every PHP file that needs it; we can add this function and its parameters there.
-
To turn off all error reporting within your PHP code space.
error_reporting(0);
-
To report simple running errors (fatal runtime errors, runtime warnings, and compile-time parse errors).
error_reporting(E_ERROR | E_WARNING | E_PARSE);
-
To report uninitialized variables or catch variable name misspellings (runtime notices).
```php
error_reporting(E_ERROR | E_WARNING | E_PARSE | E_NOTICE);
```
-
To report all errors except runtime notices.
error_reporting(E_ALL & ~E_NOTICE);
-
To report all types of PHP errors.
error_reporting(E_ALL); error_reporting(-1); ini_set('error_reporting', E_ALL);
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn