How to Extract Data From JSON in PHP
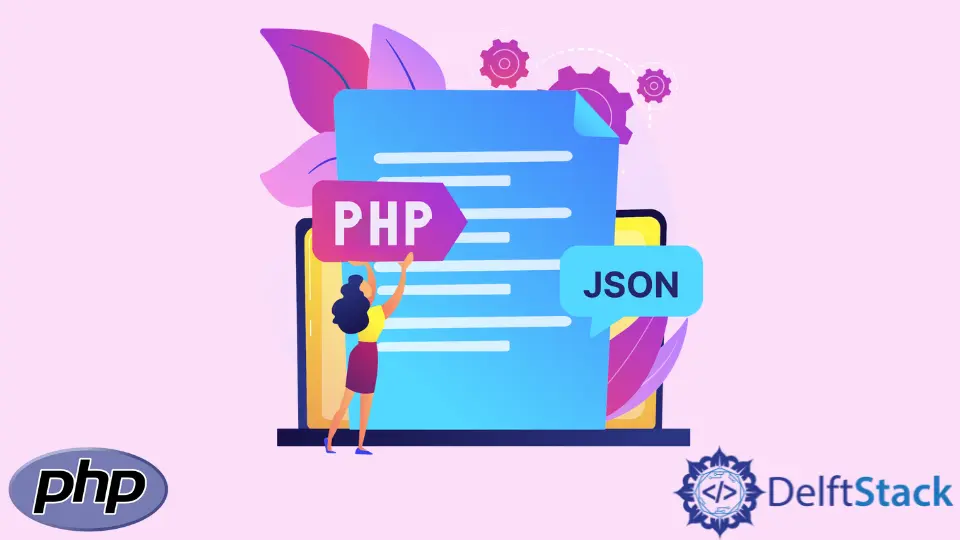
In this article, we will introduce methods to extract data from JSON in PHP.
- Using
json_decode()
function
Use json_decode()
Function to Extract Data From JSON in PHP
We will use the built-in function json_decode()
to extract data from JSON. We will convert the JSON string to an object or an array to extract the data. The correct syntax to use this function is as follows.
json_decode($jsonString, $assoc, $depth, $options);
The built-in function json_decode()
has four parameters. The details of its parameters are as follows
Parameters | Description | |
---|---|---|
$jsonString |
mandatory | It is the JSON encoded string from which we want to extract the data. |
$assoc |
optional | It is a Boolean variable. If it is TRUE, the function will return an associative array. If FALSE, the function will return the object. |
$depth |
optional | It is an integer. It specifies the specified depth. |
$options |
optional | It specifies the bitmask of JSON_BIGINT_AS_STRING , JSON_INVALID_UTF8_IGNORE , JSON_INVALID_UTF8_SUBSTITUTE , JSON_OBJECT_AS_ARRAY , JSON_THROW_ON_ERROR . You can check their details here. |
This function returns NULL if the JSON string is not in a proper format. It returns an associative array or object depending upon the $assoc
parameter.
The program below shows how we can use the json_decode()
function to extract data from a JSON string.
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth":
{
"year":"1999",
"month":"06",
"day":"19"
}
}';
$data = json_decode($jsonString);
echo("The data is: \n");
var_dump($data);
?>
The function will return an object because we have not passed the $assoc
parameter.
Output:
The data is:
object(stdClass)#1 (3) {
["firstName"]=>
string(6) "Olivia"
["lastName"]=>
string(5) "Mason"
["dateOfBirth"]=>
object(stdClass)#2 (3) {
["year"]=>
string(4) "1999"
["month"]=>
string(2) "06"
["day"]=>
string(2) "19"
}
}
If we pass the $assoc
parameter, the function will return an associative array.
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth":
{
"year":"1999",
"month":"06",
"day":"19"
}
}';
$data = json_decode($jsonString, true);
echo("The data is: \n");
var_dump($data);
?>
Output:
The data is:
array(3) {
["firstName"]=>
string(6) "Olivia"
["lastName"]=>
string(5) "Mason"
["dateOfBirth"]=>
array(3) {
["year"]=>
string(4) "1999"
["month"]=>
string(2) "06"
["day"]=>
string(2) "19"
}
}
If the function has returned an object, we can access the data in the following way:
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth":
{
"year":"1999",
"month":"06",
"day":"19"
}
}';
$data = json_decode($jsonString);
echo("The first name is: \n");
echo $data->firstName;
?>
The function will return the first name from the data extracted.
Output:
The first name is:
Olivia
If the function has returned an array, we can directly access the data in the following way:
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth":
{
"year":"1999",
"month":"06",
"day":"19"
}
}';
$data = json_decode($jsonString, true);
echo("The first name is: \n");
echo $data['firstName'];
?>
The function will return the first name from the data extracted.
Output:
The first name is:
Olivia
We can also iterate through our array.
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth": "19-09-1999"
}';
$data = json_decode($jsonString, true);
foreach ($data as $key=> $data1) {
echo $key, " : ";
echo $data1, "\n";
}
?>
The function will return the extracted data.
Output:
firstName : Olivia
lastName : Mason
dateOfBirth : 19-09-1999