Comment extraire les données de JSON en PHP
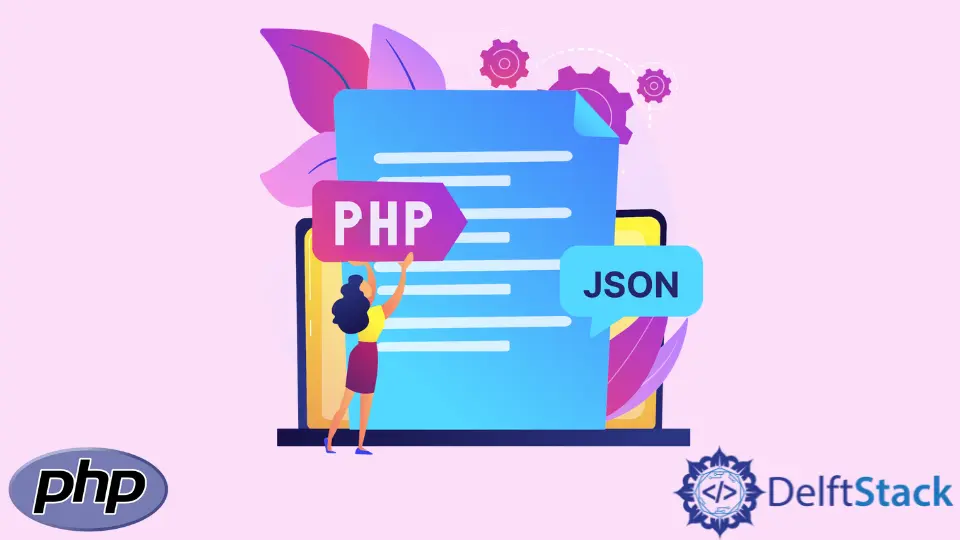
Dans cet article, nous introduirons des méthodes pour extraire des données de JSON en PHP.
- Utilisation de la fonction
json_decode()
.
Utilisez la fonction json_decode()
pour extraire des données de JSON en PHP
Nous utiliserons la fonction intégrée json_decode()
pour extraire les données de JSON. Nous allons convertir la chaîne
JSON en un objet ou un tableau
pour extraire les données. La syntaxe correcte pour utiliser cette fonction est la suivante.
json_decode($jsonString, $assoc, $depth, $options);
La fonction intégrée json_decode()
a quatre paramètres. Les détails de ses paramètres sont les suivants
Paramètres | Description | |
---|---|---|
$jsonString |
obligatoire | Il s’agit de la chaîne codée en JSON dont nous voulons extraire les données. |
$assoc |
facultatif | Il s’agit d’une variable booléenne . Si elle est VRAIE, la fonction retournera un tableau associatif. Si elle est FAUSSE, la fonction retournera l’objet. |
$depth |
facultatif | C’est un nombre entier. Il indique la profondeur spécifiée. |
$options |
facultatif | Il parle du masque de bit de JSON_BIGINT_AS_STRING **, JSON_INVALID_UTF8_IGNORE , JSON_INVALID_UTF8_SUBSTITUTE , JSON_OBJECT_AS_ARRAY , **JSON_THROW_ON_ERROR . Vous pouvez consulter leurs coordonnées ici. |
Cette fonction retourne NULL si la chaîne
de JSON n’est pas dans un format approprié. Elle retourne un tableau
ou un objet associatif en fonction du paramètre $assoc
.
Le programme ci-dessous montre comment nous pouvons utiliser la fonction json_decode()
pour extraire des données d’une chaîne
JSON.
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth":
{
"year":"1999",
"month":"06",
"day":"19"
}
}';
$data = json_decode($jsonString);
echo("The data is: \n");
var_dump($data);
?>
La fonction retournera un objet parce que nous n’avons pas passé le paramètre $assoc
.
Production:
The data is:
object(stdClass)#1 (3) {
["firstName"]=>
string(6) "Olivia"
["lastName"]=>
string(5) "Mason"
["dateOfBirth"]=>
object(stdClass)#2 (3) {
["year"]=>
string(4) "1999"
["month"]=>
string(2) "06"
["day"]=>
string(2) "19"
}
}
Si nous passons le paramètre $assoc
, la fonction retournera un tableau associatif.
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth":
{
"year":"1999",
"month":"06",
"day":"19"
}
}';
$data = json_decode($jsonString, true);
echo("The data is: \n");
var_dump($data);
?>
Production:
The data is:
array(3) {
["firstName"]=>
string(6) "Olivia"
["lastName"]=>
string(5) "Mason"
["dateOfBirth"]=>
array(3) {
["year"]=>
string(4) "1999"
["month"]=>
string(2) "06"
["day"]=>
string(2) "19"
}
}
Si la fonction a renvoyé un objet, nous pouvons accéder aux données de la manière suivante:
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth":
{
"year":"1999",
"month":"06",
"day":"19"
}
}';
$data = json_decode($jsonString);
echo("The first name is: \n");
echo $data->firstName;
?>
La fonction retournera le premier nom des données extraites.
Production:
The first name is:
Olivia
Si la fonction a renvoyé un tableau
, nous pouvons accéder directement aux données de la manière suivante:
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth":
{
"year":"1999",
"month":"06",
"day":"19"
}
}';
$data = json_decode($jsonString, true);
echo("The first name is: \n");
echo $data['firstName'];
?>
La fonction retournera le prénom des données extraites.
Production:
The first name is:
Olivia
Nous pouvons également itérer à travers notre array
.
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth": "19-09-1999"
}';
$data = json_decode($jsonString, true);
foreach ($data as $key=> $data1) {
echo $key, " : ";
echo $data1, "\n";
}
?>
La fonction renverra les données extraites.
Production:
firstName : Olivia
lastName : Mason
dateOfBirth : 19-09-1999