How to Read Images using imread in Python Matplotlib
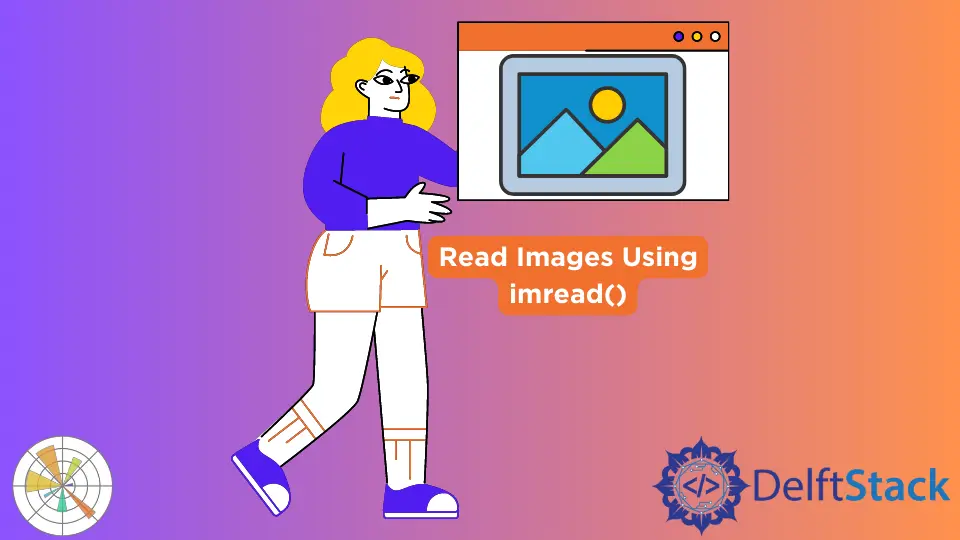
The article explains how we can read an image file into an array using the imread()
method from the Matplotlib package.
matplotlib.pyplot.imread()
The matplotlib.pyplot.imread()
reads an image from a file into an array.
Syntax
matplotlib.pyplot.imread(fname, format=None)
Here, fname
represents the name of the image file to be read, and format
represents the image file format. If format=None
the function will extract the format from the filename.
The function returns an array with the shape MxN
for grayscale images, MxNx3
for RGB images, and MxNx4
for RGBA
images, where M
is the width and N
is the height of the image.
Example: Read Images Using the matplotlib.pyplot.imread()
Function
import numpy as np
import matplotlib.pyplot as plt
img_array = plt.imread("lena.png")
plt.imshow(img_array)
plt.title("Display Image read using imread()")
plt.axis("off")
plt.show()
Output:
It reads the image lena.png
in the current working directory into an array using the imread()
method and then displays the image using the imshow()
method.
By default, it has X-axis
and Y-axis
with ticks in the displayed image. To remove the axes and ticks, we use the statement plt.axis('off')
. Finally, we use the matplotlib.pyplot.show()
function to show the image.
We can view the shape of the image array using the shape
attribute.
import matplotlib.pyplot as plt
img_array = plt.imread("lena.png")
print(img_array.shape)
Output:
(330, 330, 3)
It prints the shape of the image - (330, 330, 3)
, representing a 3-dimensional image array of width as 330, height as 330, and 3 channels.
Example: Clip Images Using the matplotlib.pyplot.imread()
Function
After matplotlib.pyplot.imread()
reads an image into a NumPy array, we can clip the image by indexing the array using the :
operator.
import matplotlib.pyplot as plt
img_array = plt.imread("lena.png")[50:300, 30:300]
plt.imshow(img_array)
plt.axis("off")
plt.title("Clipped Image")
plt.show()
Output:
Here, the imread()
method reads the full image into an array, and we only select the elements from position 50 to 300 in width and elements from position 30 to 300 in height and stores the indexed array in img_array
. We then display the indexed array using the imshow()
function.
import matplotlib.pyplot as plt
import matplotlib.patches as patches
img_array = plt.imread("lena.png")
fig, ax = plt.subplots()
im = ax.imshow(img_array)
patch = patches.Circle((160, 160), radius=150, transform=ax.transData)
im.set_clip_path(patch)
ax.axis("off")
plt.show()
Output:
It displays the image clipped using a circular patch. Here, we clip the image using a circular patch with the center at (160, 160)
and radius as 150.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn