How to Display an Image in Grayscale in Matplotlib
-
matplotlib.pyplot.imshow()
to Display an Image in Grayscale in Matplotlib - Examples: Matplotlib Display Image in Grayscale
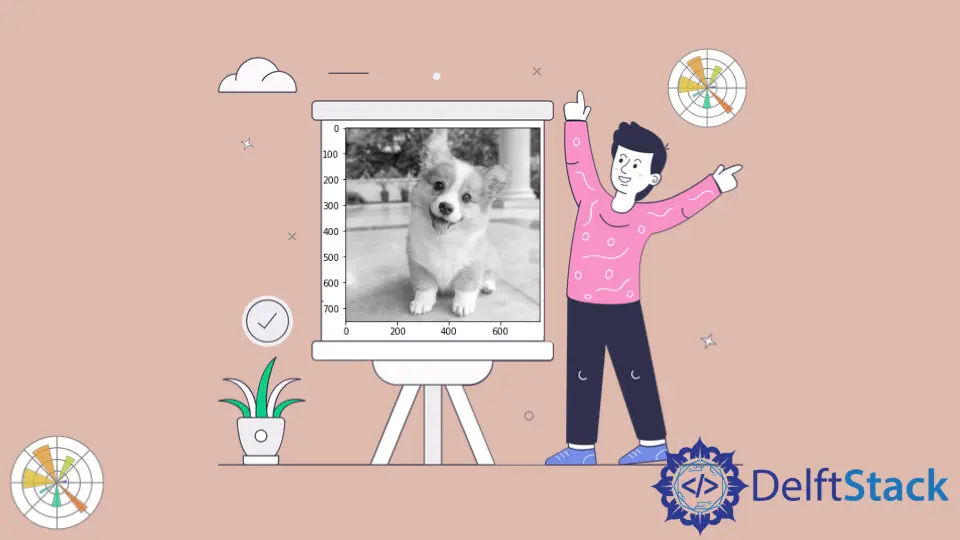
To display a grayscale image in Matplotlib, we use the matplotlib.pyplot.imshow()
with parameters cmap
set to 'gray'
, vmin
set to 0
and vmax
set to 255
.By default, the value of cmap
, vmin
and vmax
is set to None
.
matplotlib.pyplot.imshow()
to Display an Image in Grayscale in Matplotlib
matplotlib.pyplot.imshow(X,
cmap=None,
norm=None,
aspect=None,
interpolation=None,
alpha=None,
vmin=None,
vmax=None,
origin=None,
extent=None,
shape= < deprecated parameter > ,
filternorm=1,
filterrad=4.0,
imlim= < deprecated parameter > ,
resample=None,
url=None,
*,
data=None,
**kwargs)
Examples: Matplotlib Display Image in Grayscale
import matplotlib.pyplot as plt
import matplotlib.image as img
image = img.imread("lena.jpg")
plt.imshow(image[:, :, 1], cmap="gray", vmin=0, vmax=255, interpolation="none")
plt.show()
Output:
This method reads the image lena.jpg
, which is an RGB image using the imread()
function from the matplotlib.image
module. To display the image as grayscale, we only need one color channel. So for the next step, only take a single color channel and display the image using the plt.imshow()
method with cmap
set to 'gray'
, vmin
set to 0
, and vmax
set to 255
.
Finally, we use the show()
method to show a window displaying the image in grayscale. To run the script above, we must have lena.jpg
in our current working directory.
Taking only one color channel is not an appropriate way to convert RGB images to grayscale images because we have specific algorithms to make the conversion more efficient. These algorithms are implemented in almost all popular image processing libraries available. Below, we demonstrate an example using the PIL
.
import numpy as np
import matplotlib.pyplot as plt
from PIL import Image
image = Image.open("lena.jpg")
gray_image = image.convert("L")
gray_image_array = np.asarray(gray_image)
plt.imshow(gray_image_array, cmap="gray", vmin=0, vmax=255)
plt.show()
Output:
Here, we read the image lena.jpg
in our current working directory using the open()
function and then convert the image to grayscale using the convert()
method with 'L'
as an argument. After that, we convert the grayscale image into a NumPy array and display the image using the imshow()
method.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn