How to Convert a NumPy Array to PIL Image in Python
- Convert a NumPy Array to PIL Image in Python
- Convert a NumPy Array to PIL Image Python With the Matplotlib Colormap
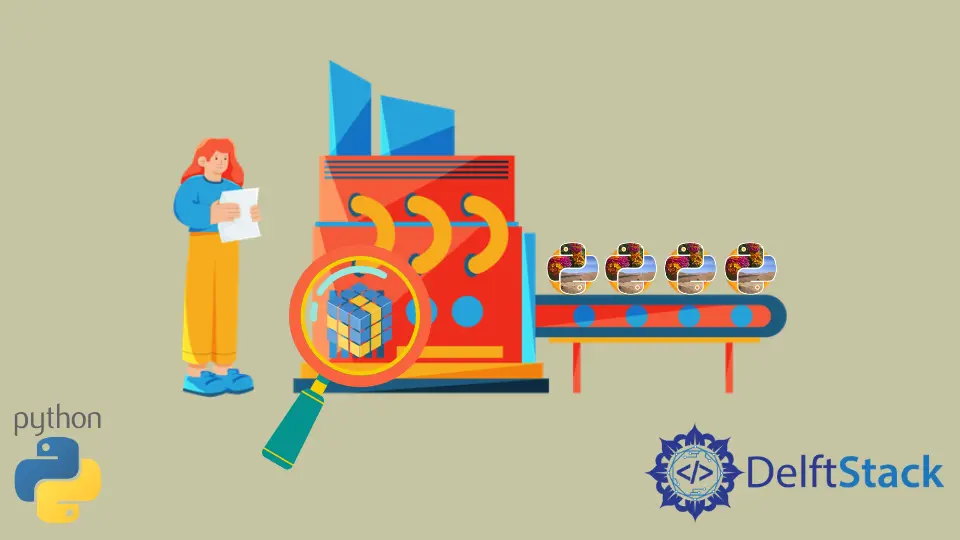
This tutorial explains how we can convert the NumPy array to a PIL
image using the Image.fromarray()
from the PIL
package. The Python Imaging Library (PIL
) is a library in Python with various image processing functions.
The Image.fromarray()
function takes the array object as the input and returns the image object made from the array object.
Convert a NumPy Array to PIL Image in Python
import numpy as np
from PIL import Image
image = Image.open("lena.png")
np_array = np.array(image)
pil_image = Image.fromarray(np_array)
pil_image.show()
Output:
It will read the image lena.png
in the current working directory using the open()
method from the Image
and return an image object.
We then convert this image object to a NumPy array using the numpy.array()
method.
We use the Image.fromarray()
function to convert the array back to the PIL
image object and finally display the image object using the show()
method.
import numpy as np
from PIL import Image
array = np.random.randint(255, size=(400, 400), dtype=np.uint8)
image = Image.fromarray(array)
image.show()
Output:
Here, we create a NumPy array of size 400x400
with random numbers ranging from 0
to 255
and then convert the array to an Image
object using the Image.fromarray()
function and display the image
using show()
method.
Convert a NumPy Array to PIL Image Python With the Matplotlib Colormap
import numpy as np
from PIL import Image
import matplotlib.pyplot as plt
from matplotlib import cm
image_array = plt.imread("lena.jpg")
image_array = image_array / 255
image = Image.fromarray(np.uint8(cm.plasma(image_array) * 255))
image.show()
Output:
It applies the plasma
colormap from the Matplotlib
package. To apply a colormap to an image, we first normalize the array with a max value of 1
. The maximum value of the element in image_array
is 255
in the above example. So, we divide the image_array
by 255 for normalization.
We then apply the colormap to the image_array
and multiply it by 255
again. Then, we convert the elements to the int
format using the np.uint8()
method. Finally, we convert the array into the image using the Image.fromarray()
function.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn