How to Save Figures Identical to the Displayed Figures in Matplotlib
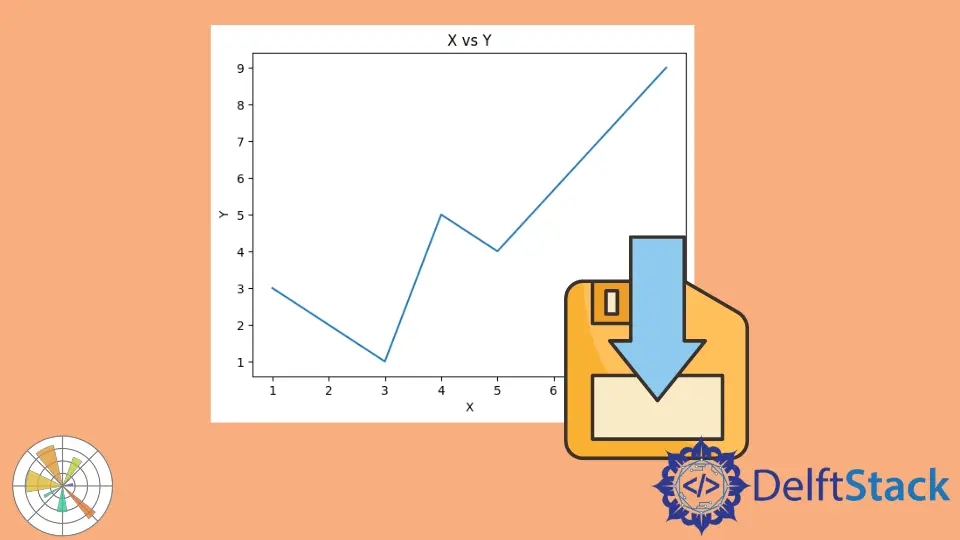
While saving the figure using matplotlib.pyplot.savefig()
, we have a parameter named dpi
, which specifies the relative size of text and the width of stroke on the lines. By default, the value of dpi
for matplotlib.pyplot.show()
is 80, while the default value of dpi
for matplotlib.pyplot.savefig()
is 100.
To make sure the figures look identical for the show()
and savefig()
method, we must use dpi=fig.dpi
in the savefig()
method. We can also set the figsize
parameter in the matplotlib.pyplot.figure()
method to adjust the plot’s absolute dimensions so that both figures are identical.
Set dpi=fig.dpi
in the savefig()
Method to Save Figures Identical to the Displayed Figures in Matplotlib
import matplotlib.pyplot as plt
x = [1, 3, 4, 5, 8]
y = [3, 1, 5, 4, 9]
fig = plt.figure()
plt.plot(x, y)
plt.xlabel("X")
plt.ylabel("Y")
plt.title("X vs Y")
fig.savefig("plot.png", dpi=fig.dpi)
plt.show()
Output:
Saved Figure:
This process saves the figure as plot.png
, which is identical to the one displayed.
Sometimes, we may get large borders on created figures. To solve this value, we can either use the matplotlib.pyplot.tight_layout()
method or set bbox_inches='tight'
in the savefig()
method.
import matplotlib.pyplot as plt
x = [1, 3, 4, 5, 8]
y = [3, 1, 5, 4, 9]
fig = plt.figure()
plt.plot(x, y)
plt.xlabel("X")
plt.ylabel("Y")
plt.title("X vs Y")
fig.savefig("plot.png", dpi=fig.dpi, bbox_inches="tight")
plt.show()
Output:
Saved Figure:
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn