Convertir un tableau numérique en image PIL en Python
- Convertir un tableau NumPy en image PIL en Python
- Convertir un tableau NumPy en image PIL Python avec la fonction Matplotlib Colormap
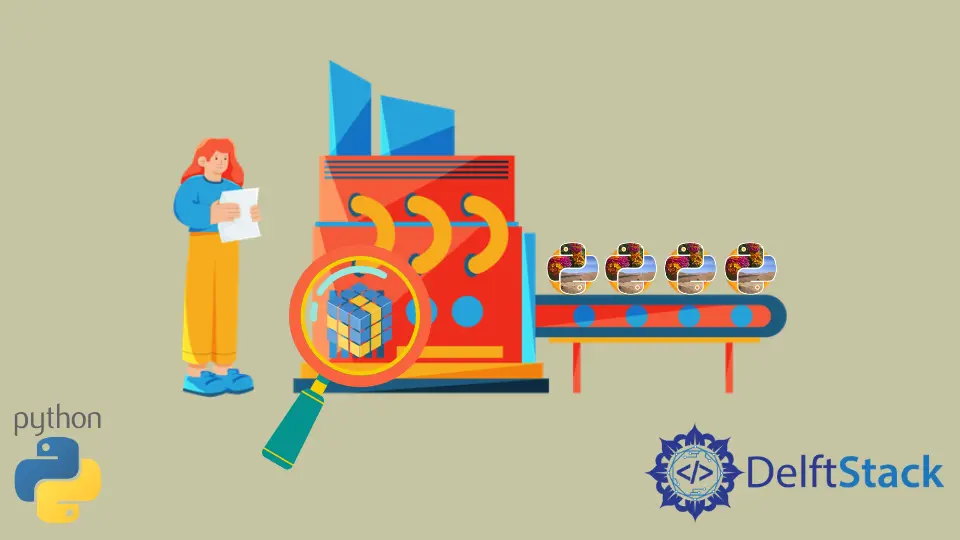
Ce tutoriel explique comment nous pouvons convertir le tableau NumPy en une image PIL en utilisant l’option Image.fromarray()
du paquet PIL
. La Python Imaging Library (PIL
) est une bibliothèque en Python avec diverses fonctions de traitement d’images.
La fonction Image.fromarray()
prend l’objet tableau comme entrée et renvoie l’objet image créé à partir de l’objet tableau.
Convertir un tableau NumPy en image PIL en Python
import numpy as np
from PIL import Image
image = Image.open("lena.png")
np_array = np.array(image)
pil_image = Image.fromarray(np_array)
pil_image.show()
Production :
Il lira l’image lena.png
dans le répertoire de travail courant en utilisant la méthode open()
de Image
et retournera un objet image.
Nous convertissons ensuite cet objet image en un tableau NumPy en utilisant la méthode numpy.array()
.
Nous utilisons la fonction Image.fromarray()
pour reconvertir le tableau en objet image PIL
et finalement afficher l’objet image en utilisant la méthode show()
.
import numpy as np
from PIL import Image
array = np.random.randint(255, size=(400, 400), dtype=np.uint8)
image = Image.fromarray(array)
image.show()
Production :
Ici, nous créons un tableau NumPy de taille 400x400
avec des nombres aléatoires allant de 0
à 255
puis nous convertissons le tableau en un objet Image
en utilisant la fonction Image.fromarray()
et nous affichons image
en utilisant la méthode show()
.
Convertir un tableau NumPy en image PIL Python avec la fonction Matplotlib Colormap
import numpy as np
from PIL import Image
import matplotlib.pyplot as plt
from matplotlib import cm
image_array = plt.imread("lena.jpg")
image_array = image_array / 255
image = Image.fromarray(np.uint8(cm.plasma(image_array) * 255))
image.show()
Production :
Il applique la carte de couleurs plasma
du paquet Matplotlib
. Pour appliquer une carte de couleurs à une image, nous normalisons d’abord le tableau avec une valeur maximale de 1
. La valeur maximale de l’élément dans image_array
est de 255
dans l’exemple ci-dessus. Donc, nous divisons le image_array
par 255 pour la normalisation.
Nous appliquons ensuite la carte de couleurs à image_array
et nous la multiplions par 255 à nouveau. Ensuite, nous convertissons les éléments au format int
en utilisant la méthode np.uint8()
. Enfin, nous convertissons le tableau en image en utilisant la fonction Image.fromarray()
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn