How to Label Lines in Matplotlib
-
Label Lines With the
label
Parameter in Matplotlib -
Set Label/Legend Positions With the
loc
Parameter in Matplotlib
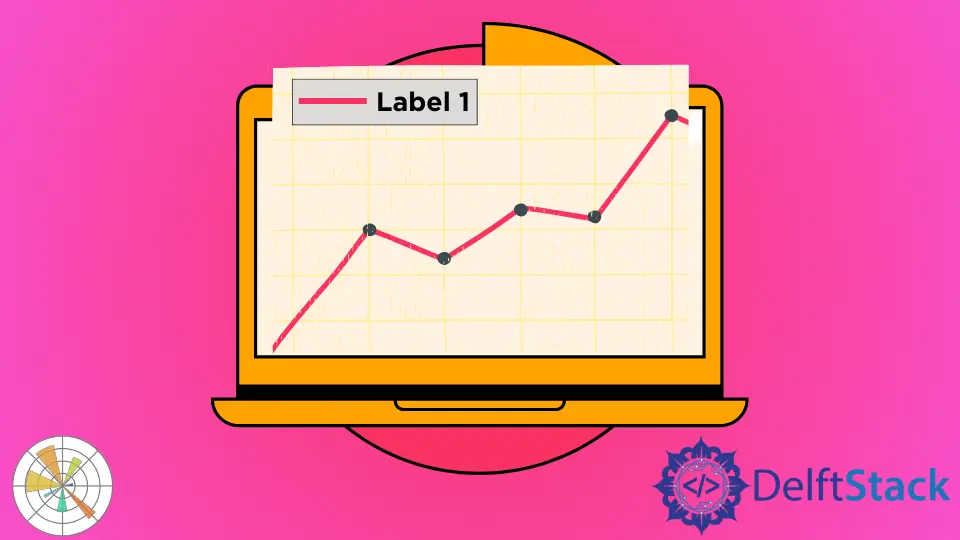
This tutorial will demonstrate how to label lines in Matplotlib and place them inside the plot.
Before working with plots, we need to set up our script to work with the library. So we start by importing matplotlib.
Furthermore, we load the randrange
function from the random
module to quickly generate some data. Remember that it will look different for you.
import matplotlib.pyplot as plt
from random import randrange
data_1 = [randrange(0, 10) for _ in range(0, 10)]
Label Lines With the label
Parameter in Matplotlib
We label lines or other kinds of plots with the label
parameter, which we provide with a string. But for the label to show, we also have to call the legend
function.
plt.plot(data_1, label="Random Data")
plt.legend()
plt.show()
Output:
Set Label/Legend Positions With the loc
Parameter in Matplotlib
It is also possible to set the position of the legend. We do this with the loc
parameter of the legend method.
plt.legend(loc="lower left")
Output:
The following positions are valid arguments:
upper right
upper left
lower left
lower right
right
center left
center right
lower center
upper center
center
Complete code:
import matplotlib.pyplot as plt
from random import randrange
data_1 = [randrange(0, 10) for _ in range(0, 10)]
data_2 = [randrange(0, 10) for _ in range(0, 10)]
plt.plot(data_1, label="Random Data")
plt.legend(loc="lower left")
plt.show()
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub