How to Assign One Variable to Another in Bash
- Method 1: Direct Assignment
- Method 2: Using Command Substitution
- Method 3: Using Arrays
- Conclusion
- FAQ
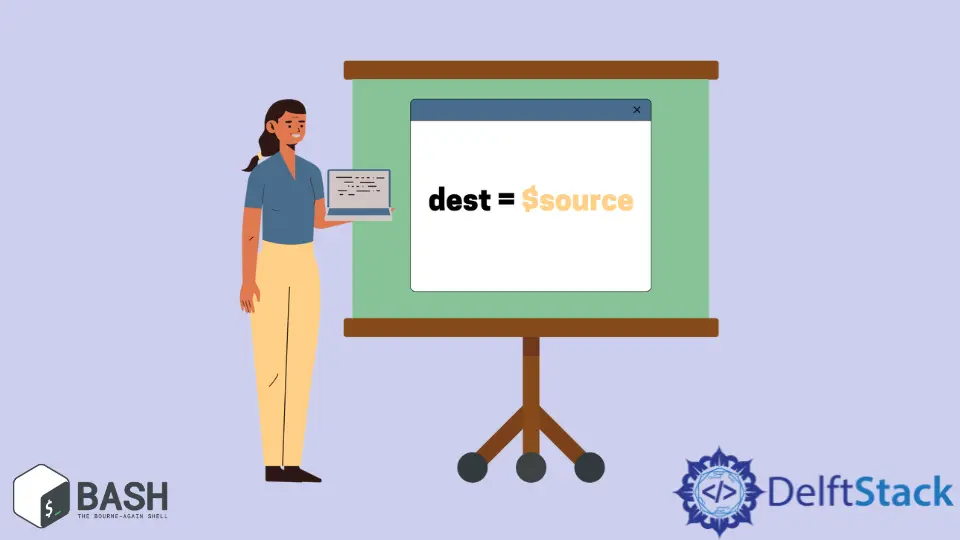
When working with Bash, understanding how to manipulate variables is crucial for writing effective scripts. One common task is assigning the value of one variable to another. This can be particularly useful when you want to create a copy of a variable or need to modify values while keeping the original intact.
In this tutorial, we will explore different methods to assign one variable to another in Bash, providing clear examples and explanations. Whether you are a beginner or looking to refine your scripting skills, this guide will help you grasp the concept with ease. Let’s dive in!
Method 1: Direct Assignment
The simplest way to assign one variable to another in Bash is through direct assignment. This method involves creating a new variable and setting it equal to the existing variable.
Here’s how it works:
original_variable="Hello, World!"
new_variable="$original_variable"
Output:
Hello, World!
In this example, we first declare a variable called original_variable
and assign it the string “Hello, World!”. Next, we create a new variable named new_variable
and assign it the value of original_variable
. The dollar sign before original_variable
indicates that we want to use its value rather than its name. When you run this code, new_variable
will contain the same string as original_variable
.
This method is straightforward and efficient for copying values. However, keep in mind that if you change the value of original_variable
later, new_variable
will remain unchanged. This is because Bash variables are assigned by value, not by reference.
Method 2: Using Command Substitution
Another way to assign one variable to another in Bash is through command substitution. This method is particularly useful when the value you want to assign is the result of a command.
Here’s an example:
original_variable=$(date)
new_variable="$original_variable"
Output:
Tue Oct 03 12:34:56 UTC 2023
In this code snippet, original_variable
is assigned the output of the date
command, which fetches the current date and time. We then assign new_variable
the value of original_variable
. The use of $(...)
for command substitution allows us to execute a command and use its output as a variable value.
Just like in the previous method, if you modify original_variable
later, new_variable
will retain its original value. This approach is particularly powerful when you need to capture and store the output of commands for later use.
Method 3: Using Arrays
Bash also supports arrays, which can be another way to assign values. When you want to copy an array, you can use a different syntax to ensure that you are copying the entire array rather than just a single value.
Here’s how to do it:
original_array=(one two three)
new_array=("${original_array[@]}")
Output:
one two three
In this example, original_array
is an array containing three elements: “one”, “two”, and “three”. To assign this array to new_array
, we use the syntax ("${original_array[@]}")
. This notation ensures that all elements of original_array
are copied to new_array
.
Arrays are particularly useful when you need to handle multiple values and want to keep them organized. Just as with scalar variables, changes to original_array
will not affect new_array
after the assignment.
Conclusion
In this tutorial, we have explored various methods to assign one variable to another in Bash. From direct assignments to command substitutions and array handling, each method serves its purpose depending on the context. Understanding these techniques can significantly enhance your scripting capabilities, enabling you to write cleaner and more efficient Bash scripts. Remember, the choice of method often depends on your specific needs and the nature of the data you are working with. Happy scripting!
FAQ
-
How do I assign a variable in Bash?
You can assign a variable in Bash using the syntaxvariable_name=value
. No spaces are allowed around the equal sign. -
Can I assign multiple variables in one line?
Yes, you can assign multiple variables in one line by separating them with spaces, like this:var1=value1 var2=value2
. -
What happens if I change the original variable after assignment?
The new variable will retain its original value, as Bash assigns variables by value, not by reference. -
How can I check the value of a variable in Bash?
You can check the value of a variable by using theecho
command, like this:echo $variable_name
.
- Are there any special characters I should avoid in variable names?
Yes, it’s best to avoid spaces and special characters (except underscores) in variable names. Stick to alphanumeric characters and underscores for best practices.