Bash Variable Scope
- Bash Variable Scope in Linux
- How to Declare a Variable in Bash
- the Scope of Variables in Bash
- Types of Variables
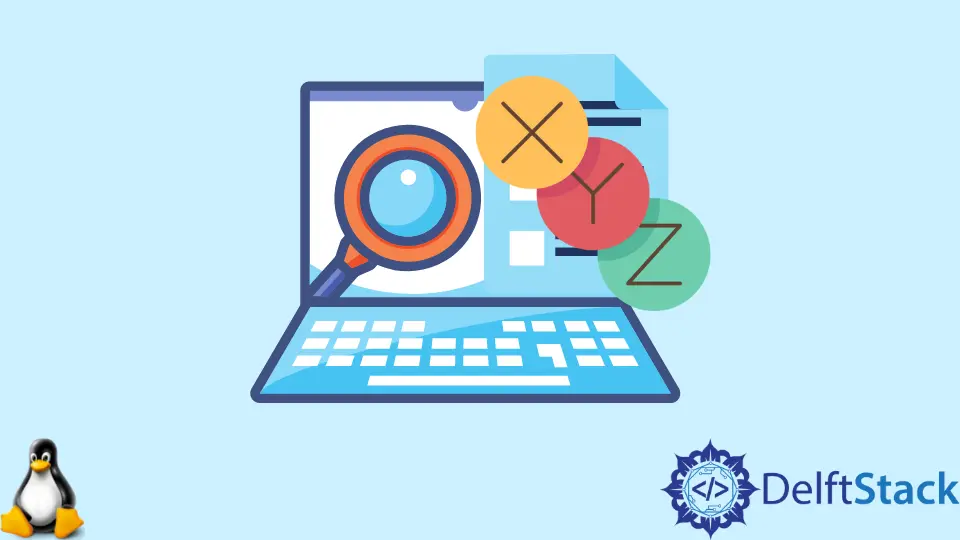
In the article, we will learn about bash variable scope
in Linux. We also learn how to declare a variable in the bash script in Linux operating system.
Further, we will see different types of variables and how to access local and global variables in bash script using Linux OS. Let’s begin with the concept of variables used in Linux.
Bash Variable Scope in Linux
Variables are temporary storage spaces for various data types to be handled in any programming language. A variable is frequently correlated to two separate entities: a data type and its value.
The data type describes the data kept in a variable, whereas the value represents the data itself. When declaring a variable in Bash programming, we do not need to specify the data type explicitly.
This is because the programming language detects the data type itself. In bash, for example, if we assign a number to a variable, it is treated as an integer right away.
How to Declare a Variable in Bash
In bash, we can declare a variable with any name we want using an equal sign '='
, and with the value, we want to allocate it. Here are some rules we can follow to declare variables in bash:
- Upper and lowercase letters, numerals, underscores, and decimals are all acceptable characters for variable names.
- Uppercase letter use for variable names in Bash scripts is recommended.
- Do not give space around the equal sign
'='
. - All pre-defined keywords are restricted, for instance,
if
,else
,local
, etc.
Below is the example of declaring a String variable in bash. We open the terminal and declare the variable. Don’t forget that we are not allowed to put any space around the equal ('='
) sign while declaring variables.
$ _name=abid
We use echo
to get the output of the variable.
$ echo $_name
Output:
abid
Declaring an Integer variable in bash.
$ _age=24
Likewise, with echo
, we can get the output of this variable too.
$ echo $_age
Output:
24
the Scope of Variables in Bash
The scope of a Bash variable can be either local or global, just like in any other programming language. It doesn’t matter where they are declared in our Bash script.
All the variables in bash have a global scope by default. This indicates that a variable can be utilized inside any function in a bash script, even if it is defined in the middle of the script.
To put it another way, we may argue that in bash, we do not always have to declare a variable at the start of a script to make its scope global.
Furthermore, we must explicitly use the local
keyword when declaring a variable in bash if we want the scope of that variable to be limited to a particular function, meaning that no other function in that script or anyplace else should be able to access it.
This will ensure that the variable’s scope is restricted to the function in which it was defined.
Types of Variables
There are mainly three types of variable scope that we need to know.
- Local Variables
- Global Variables
- Environment Variables
Accessing Local Variable in Bash
We can access Local variables only inside the code block in which we have declared them. For instance, if we declare a variable within our Bash script, we won’t be able to access it outside the code.
Example Code:
#!/bin/bash
echo "Learning the scope of local and global variables."
function_localVar(){
echo "Within the function function_localVar"
echo "Assign a variable with local keyword to a variable name: varLocal"
local varLocal=27
echo "Assign a variable without local keyword to a variable name: varLocal_wo"
varLocal_wo=9
echo "Printing within the function."
echo "Value assigned to one with local keyword varLocal = $varLocal and without local keyword varLocal_wo = $varLocal_wo"
echo "Exiting function_localVar"
}
glob_var=91
echo "Start of the code!"
echo "Global Variable = $glob_var"
echo "Local variable before calling function to one with local keyword varLocal = ** $varLocal ** and without local keyword varLocal_wo = ** $varLocal_wo **"
echo "Calling the function."
function_localVar
echo "Printing outside the function."
echo "Value assigned to one with local keyword varLocal = $varLocal and without local keyword varLocal_wo = $varLocal_wo"
Output:
Learning the scope of local and global variables.
Start of the code!
Global Variable = 91
Local variable before calling function to one with local keyword varLocal = ** ** and without local keyword varLocal_wo = ** **
Calling the function.
Within the function function_localVar
Assign a variable with local keyword to a variable name: varLocal
Assign a variable without local keyword to a variable name: varLocal_wo
Printing within the function.
Value assigned to one with local keyword varLocal = 27 and without local keyword varLocal_wo = 9
Exiting function_localVar
Printing outside the function.
Value assigned to one with local keyword varLocal = and without local keyword varLocal_wo = 9
It is clear from the following code that perhaps the local keyword sets the scope local
, and even when the variable is read outside of the function where it has been defined as local
, it returns empty.
Accessing Global Scope Variables in Bash
We can access Global variables all across the bash script. This example below help us understand how Global variables scope functions/works in bash.
Example Code:
var1='Abid'
var2='Ali'
my_function () {
local var1='Micheal'
var2='Den'
echo "Inside function: var1: $var1, var2: $var2"
}
echo "Before executing the function: var1: $var1, var2: $var2"
my_function
echo "After executing the function: var1: $var1, var2: $var2"
Output:
Before executing the function: var1: Abid, var2: Ali
Inside function: var1: Micheal, var2: Den
After executing the function: var1: Abid, var2: Den
The results above piece of code lead us to the following conclusion:
- Whenever a local variable with the same name as an existing global variable is set within the scope of the function, the local variable will take priority.
- Changes to global variables can indeed be made inside the function.
Environment Variable
Environment variables (ENVs
) essentially specify how the environment works. In bash, an environment variable can have either global or local scope.
We can access anywhere inside the environment of a terminal a globally scoped ENV
generated in that terminal. Because it operates in the context bounded by that terminal, it may be used in any script, program, or process.
Any application or process executing in the terminal cannot access a locally scoped ENV
specified there. Only the terminal we have defined the variable has access to it.
Setting Environment Variables
Environment Variable as Global Scope:
$ export COUNTRY=USA
or
$ set NAME=Abid
Environment Variable as Local Scope:
$ NAME=Abid
Now, the point is how to display environment variables. We can display Environment Variable just like normal variables using echo
.
$ echo $COUNTRY
Output:
USA
The output of this command will be the value we have assigned to this variable COUNTRY
, which is USA
.
Display All the Environment Variables
We can display all the ENVs
using three different commands.
-
// for displaying all the global Environment Variables only $ printenv
-
// We use this command to display local and global Environment Variables $ set
-
// for displaying all global Environment Variables $ env
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn