How to Execute Commands in a Variable in Bash Script
- Bash Script Variable
-
Use the
Eval
Command to Execute Commands in a Variable in Bash Script -
Use
bash
With the-c
Flag to Execute Commands in a Variable in Bash Script -
Use Command Substitution
$()
to Execute Commands in a Variable in Bash Script
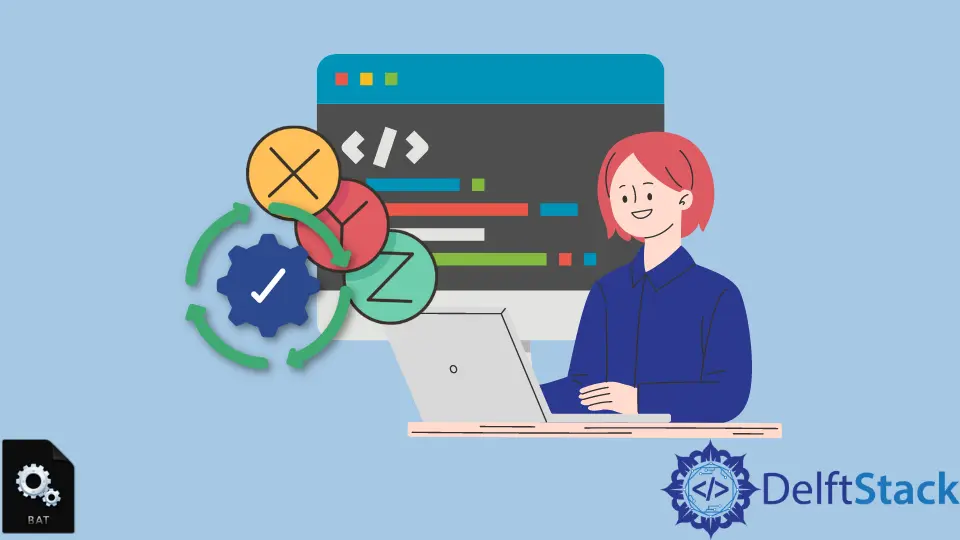
This guide is about storing a Bash command in a variable and later executing it directly from this variable. First, we will discuss multiple methods to execute commands contained in a variable, followed by several script examples.
Let’s get started.
Bash Script Variable
Variables are an essential feature powered by Bash. Whether you use them in an if
control structure, a case
structure, or simply within scripts, they not only neaten up your scripts but also make understanding them a whole lot easier.
You can also run them as scripts, making life especially easier when you are piping many commands and the commands are very long. This will reduce the length of your script, which might be a hundred words long to one, which might not even be a dozen words long.
Imagine debugging a script that is a hundred words long versus one which is not even a dozen words long; contemplate the time you can save.
Sometimes, we need to write Bash wrappers that take commands from the user, store them into variables, and conditionally run some of them after applying some business logic (maybe a series of if-else
decisions). Now, in these scenarios, we need to look at the methods that can help us in parsing and interpreting the command stored in variables to run it as a Bash command.
Here we will discuss a few of the most efficient ways to run commands contained in a variable.
Use the Eval
Command to Execute Commands in a Variable in Bash Script
Some Bash scripts require you to build a string using input values or variables (for instance) and run it as a command at the end. The eval
command is the answer in these situations.
To evaluate parameters like a shell command, use the Bash eval
command.
The shell command receives a string of arguments and uses that string to execute the command. Then the command is executed in the current shell by eval
.
This command is useful when executing a command that uses a specific operator or reserved terms.
Syntax:
eval [arguments...]
Arguments are passed to this command, combined as a string literal, and then sent to Bash for execution. This command returns the exit status after the execution of the command.
If no arguments are passed to this command or null is passed, it returns 0 as the exit status.
Example 1: Count the Number of Words in a File.
Suppose we have a file mysample.txt
containing some lines of text. If we have to count the number of words in that file, we can use the wc -w
command.
We will perform this task using the eval
command. The Bash script will be:
#!/bin/bash
wordcount="wc -w mysample.txt"
eval $wordcount
Let’s look at its output:
Example 2: Use Multiple Variables to Build the Command.
Suppose we need to print a statement Welcome to Eval Command
. We can do this using the eval
command:
#!/bin/bash
var1="Welcome to Eval Command"
comm1="echo"
eval $comm2 $var1
In this script, we have created two variables, one containing the message to be printed and the other containing the echo
command. We will pass these two variables to the eval
command, and it will construct the whole command accordingly.
After execution of this script, we will get the following output:
Example 3: Print the Values of Variables.
In the following example, we will print the value of a variable that contains another variable.
#!/bin/bash
str1="my script"
str2= str1
comm="echo "
eval $comm '$str2'
The output of the above script will be:
Example 4: Print the Sum of the Numbers.
In the following example, we will print the sum of the numbers ranging from 1 to 4 using the for
loop in the script. Then we will print that sum using the eval
command.
The script for this problem is:
#!/bin/bash
sum=0
for n in {1..4}
do
sum=$(($sum+$n))
done
comm="echo 'The result of sum from 1-4 is: '"
eval $comm $sum
The output of the above script will be:
Use bash
With the -c
Flag to Execute Commands in a Variable in Bash Script
By default, the bash
command interpreter reads commands from the standard input or a file and executes them. However, if the -c
flag is set, the bash
interpreter reads commands from the first string parameter (and it should be a non-option argument).
Therefore, we can use it to execute commands stored in a Bash string variable.
For example, the following script first assigns the ls
command string to the COMMAND
variable. Then, it uses the bash
interpreter with the -c
command to use the COMMAND
variable as an input.
#!/bin/bash
COMMAND="ls"
bash -c $COMMAND
The command above will list all the directories and files in the current working directory of the script file.
Use Command Substitution $()
to Execute Commands in a Variable in Bash Script
We can use the command substitution method to run the command stored in a variable as:
#!/bin/bash
COMMAND="ls"
$(echo $COMMAND)