How to Increment Variable Value by One in Shell Programming
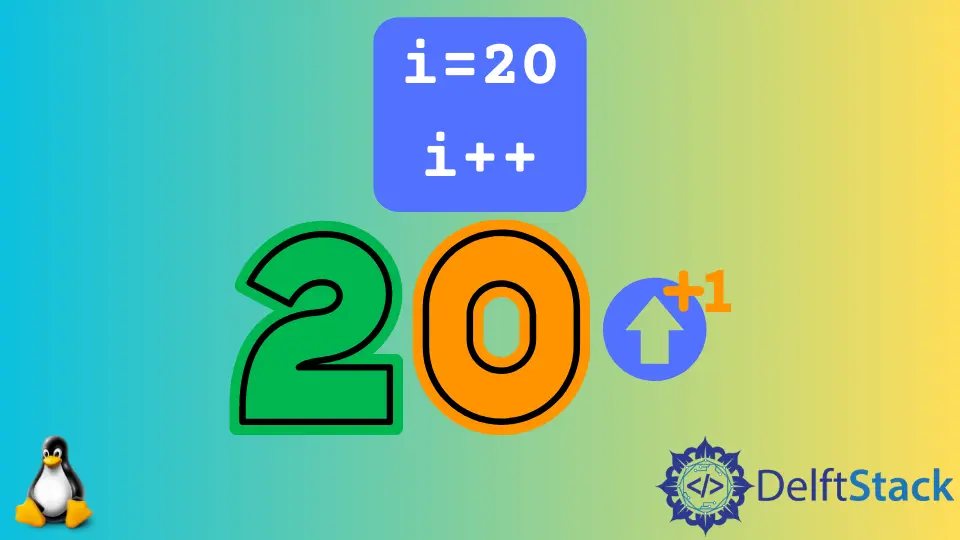
Today, we will learn how to increment a variable in bash. We will also learn different types of increment operators used in bash scripts in Linux.
Let’s begin with shell programming in Linux and understand how to increment a variable in bash.
Increment a Variable by One in Bash Using Linux
The Linux Bash Shell is a full-featured shell with programming capabilities. Variables and arithmetic operations, such as sum, divide, subtract, multiply, and more, are available in the bash.
This incrementation procedure can be performed in various ways in the bash shell. So, let’s explore them below.
Types of Increment Operators Used in Bash
There are many ways to increment a variable’s value in bash programming, which includes
+
operator++
operator+=
operator- Loop
Let’s discuss each of the above methods with code examples.
Use the +
Operator to Increment a Variable’s Value by One
In Bash Programming, we use the +
operator with the $
sign to do the increment. It is the simplest way to increment a variable.
Using the +
operator, we can increment the value by 1
. Let’s try it with an example:
Example Code:
# declear a variable ad assign it value 0
i=0
# print it to check the value of the variable
echo $i
The output of the above code:
0
Now we’ll use the +
operator to increment the value by 1
.
# use the (``+` and `$` ) sign to increment the value of the variable by one
i=$((i+1))
# print the variable to check the new value
echo $i
The output after the increment:
1
Use the ++
Operator to Increment the Variable’s Value by One
The most practical way to increment a bash variable with a single statement is to use the ++
operator. The increment value and other details don’t need to be specified.
The variable and the ++
operator can be used together directly. There are two methods for using the ++
operator.
Prefix
- The++i
version is known as the prefix, and the variable value is increased by one before use.Postfix
- Thei++
version is called postfix, and the variable value is incremented by one after use.
Example to Demonstrate Prefix
Let’s look at examples to better grasp the prefix
and postfix
operators. Starting with the prefix
increment operator by declaring a variable and assigning it a value.
i=2
Now, we will use the ++
operator for increment and print the variable in one line of code.
Example code using the ++
operator:
echo $((++i))
And the output of the code is:
3
Example to Demonstrate Postfix
The following code example explains how we can use the postfix
increment operator. First, declare a variable and assign it a value.
i=12
Now use the postfix
operators for increment and print it.
echo $((i++))
The above code produces the following output:
12
Look at the below example code to understand the prefix
and postfix
operators.
Example Code:
i=20
echo $i
echo $((i++))
echo $i
echo $((++i))
echo $i
echo $((i++))
echo $i
The output of the code:
20
20
21
22
22
22
23
Use the +=
Operator to Increment the Variable’s Value by One
The +=
operator is another popular operator we can use to increase a bash variable. While using this operator, we write one line of code to assign both the first operand and the result variable.
First, we will create a variable and assign it a value, then we will use +=
for increment, and after this, we will print the variable to check the new value.
Example code using the +=
operator:
i=55
((i+=1))
echo $i
The output of the code:
56
Use a for
Loop to Increment the Variable’s Value by One Repeatedly
One of the most important tasks when using loops in any programming language is incrementing the value of a counter or an iterator.
Doing so assists us in reaching the loop’s termination condition, without which our loop will run indefinitely.
Today, we will look at the many techniques for incrementing a variable in bash. But, first, let’s try some increment examples using a loop.
Example code using the for
loop:
for ((j = 0 ; j < 5 ; j++ )); do echo "$j"; done
The output of the code:
0
1
2
3
4
5
6
7
8
9
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn