Variable Interpolation in Bash Script
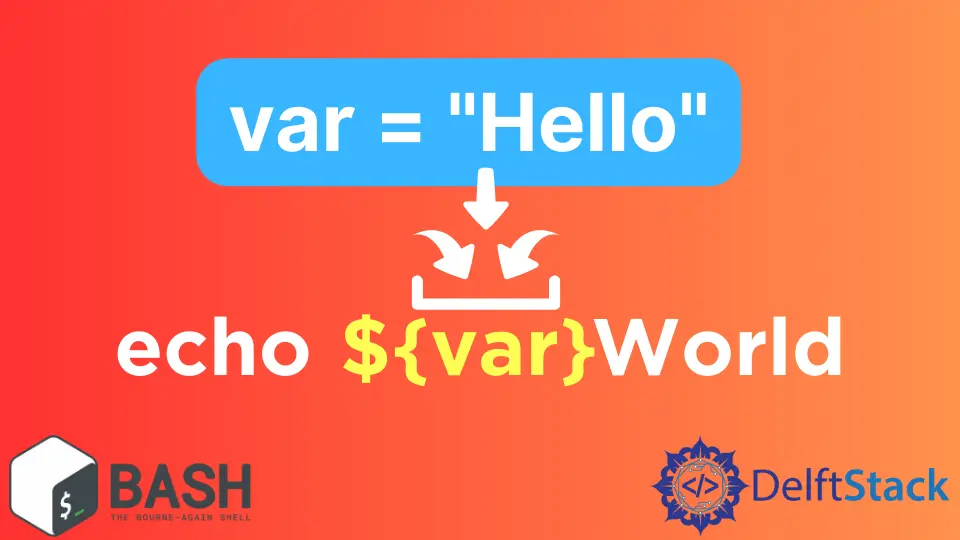
This article is about using variables in a Bash script and how to interpolate these variables in a Bash script.
Variables in Bash Script
Every programming language has variables that have specific data types. Similarly, Bash scripting also allows us to use variables to store our data values.
Unlike the other programming languages, Bash does not restrict us from having a data type of the variables. Bash variables can contain strings, numbers, characters, or anything you want.
Moreover, we don’t need to declare a variable to use it. Just assigning it a value before referencing it is enough.
Let’s look at the simple example of creating and using a variable:
#!/bin/bash
var="Hello World"
echo $var
This script creates and stores the var
variable as a string value. Later, in the following line, we printed the value of that variable.
Note that for referencing a variable, we use a $
sign to replace the value when the script is executed.
Variable Interpolation in Bash Script
You often need to use the variable value and concatenate it with another text or number. For that, we need braces to reference a variable.
The question arises of where to use curly braces {}
and where to use round braces ()
. Let us look at the difference between the two.
Use of Curly Braces {}
Curly braces are known as Parameter Expansion. Curly braces are used when we need to print a character other than space after the variable’s value.
Then, we put our variable inside the curly braces {}
like this:
#!/bin/bash
var="Hello"
echo ${var}World
Note that in the above script, we created a variable var
and stored Hello
. In the previous example, we referenced it without the curly braces because we didn’t need to add another word with it.
If we do not add curly braces now, it will search for a variable named varWorld
, which is not found. So, to tell the script the exact variable name, we have enclosed it in the curly braces.
The output of it is as follows:
Use of Round Braces ()
Round Braces are known as Command Expansion. Command substitution allows the command’s output to substitute the command itself.
After removing trailing newlines, Bash executes the command and replaces the command substitution with the command’s standard output. Embedded newlines are not erased; however, they may be removed during word splitting.
Command substitution occurs when an order is enclosed, as follows:
#!/bin/bash
day = $(date)
echo "Today is ${day}"
In the above script, the date
is a command that tells the current system date and time. So, upon executing the script, the date
is replaced with the command’s output and assigned to the variable day
.
The echo
command prints the following line: