Multi-Dimensional Array in Bash
- Basic Way for Declaring a Multi-Dimensional Array in Bash
- Declare 2-Dimensional Array Using an Associative Array in Bash
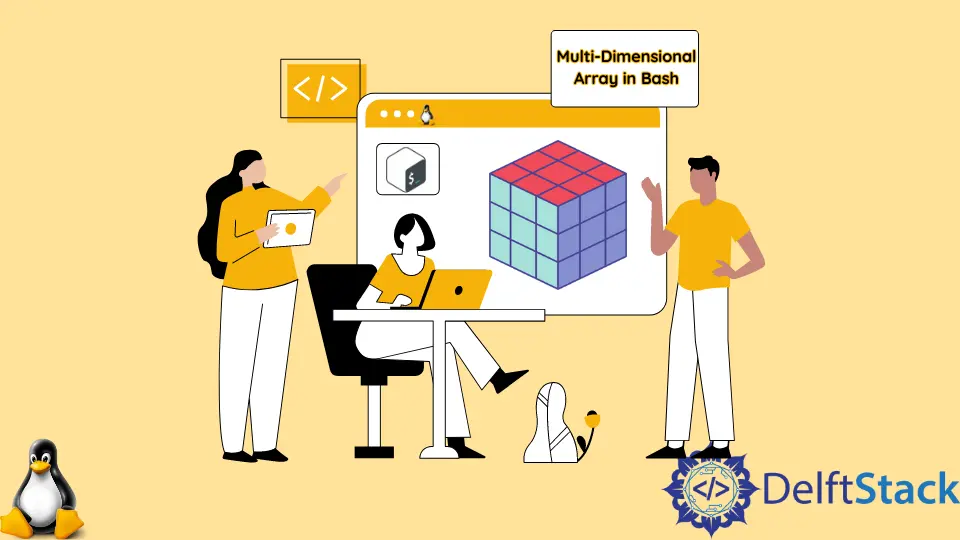
A multi-dimensional array is a very important element for any program. It is mainly used to create a table view of the data and for many other purposes.
This article demonstrates how to create a 2-dimensional array. Also, we will discuss the topic with necessary examples and explanations.
We will discuss two different methods here.
Basic Way for Declaring a Multi-Dimensional Array in Bash
This is the most basic method of creating a multi-dimensional array. In our example below, we will create a very basic two-dimensional array.
Below is the code for our example.
ArrayOfArray_1=("Alen" "24")
ArrayOfArray_2=("Walker" "31")
MainArray=(
ArrayOfArray_1[@]
ArrayOfArray_2[@]
)
ArrayLength=${#MainArray[@]}
for ((i=0; i<$ArrayLength; i++))
do
name=${!MainArray[i]:0:1}
age=${!MainArray[i]:1:1}
echo "Name : ${name}"
echo "Age : ${age}"
done
The code above shows that we declared two different arrays named ArrayOfArray_1
and ArrayOfArray_2
. After that, we declared our main array, whose element is these two arrays we recently declared.
Then we calculated the length of the array for a loop. Inside the loop, we extract the data from these two sub-array by indexing the main array.
Lastly, we echo
the final result. After executing the script, you will get an output like the one below.
Name : Alen
Age : 24
Name : Walker
Age : 31
Declare 2-Dimensional Array Using an Associative Array in Bash
This method is a bit complex. This method will discuss a special Bash script array named the associative array.
An associative array is a special array that can store string value as a key or index. It’s similar to the other programming languages.
The general syntax for declaring an associative array is declare -A ArrayName
.
Our example below will create a 2D array containing five rows and six columns. Below is the code for our example.
declare -A Array2D
RowNum=5
ColumnNum=6
for ((i=1;i<=RowNum;i++)) do
for ((j=1;j<=ColumnNum;j++)) do
Array2D[$i,$j]=$RANDOM
done
done
f1="%$((${#RowNum}+1))s"
f2=" %9s"
printf "$f1" ''
for ((i=1;i<=RowNum;i++)) do
printf "$f2" $i
done
echo
for ((j=1;j<=ColumnNum;j++)) do
printf "$f1" $j
for ((i=1;i<=RowNum;i++)) do
printf "$f2" ${Array2D[$i,$j]}
done
echo
done
In the example above, we declared an associative array named Array2D
.
After that, we created two variables and assigned them an integer value. This integer value specifies the number of rows and columns.
Then we created a nested loop that filled the array with the random number.
Now we are done with declaring and organizing the array. It’s time to see what our 2D array looks like.
We do that by using some loops, as seen in the code. When you execute the above script, you will see an output like the below.
1 2 3 4 5
1 16700 5241 2599 24330 1662
2 23264 19557 10425 13413 25606
3 17987 4199 13598 23897 26734
4 24420 27830 24855 8165 13531
5 15495 18790 13347 12947 11826
6 23458 22838 137 32454 32441
All the codes in this article are written in Bash. It will only work in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn