How to Check if a Variable Has a Value in Bash
-
Use
if...else
to Check if a Variable Has a Value in Bash - Use Double Square Brackets to Check if a Variable Has a Value in Bash
- Use the Parameter Expression to Check if a Variable Has a Value in Bash
-
Use the
-z
Conditional Expression to Check if a Variable Has a Value in Bash -
Use the
-n
Conditional Expression to Check if a Variable Has a Value in Bash
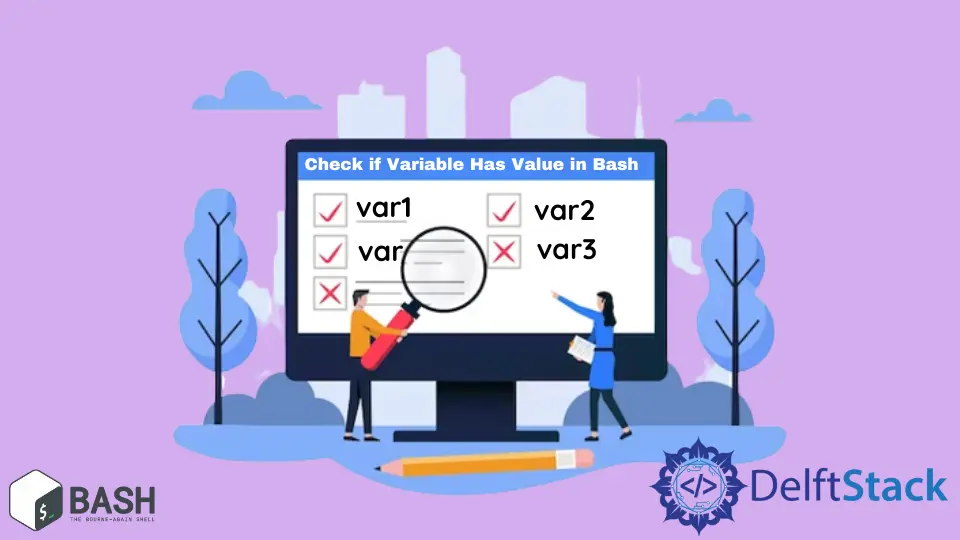
Sometimes, we need to check whether the variable contains a value or not. This is important when working on a project that contains user input to run.
In a Bash script, we can follow many ways to check whether a variable contains a value or not. This article will show how we can check a variable for value.
Also, we will see necessary examples and explanations to make the topic easier.
Use if...else
to Check if a Variable Has a Value in Bash
The first method we will use for checking a variable is the if...else
statement. We can also check whether a variable contains a value or not by using this method.
To do this, you can follow the example code shown below:
VAR=
if [ x"${VAR}" == "x" ]; then
echo "No value found for the variable"
else
echo "Value found for the variable"
fi
In the example above, through the line if [ x"${VAR}" == "x" ];
, we checked whether the variable contains a value. When you execute the code, you will get the following output:
No value found for the variable
Use Double Square Brackets to Check if a Variable Has a Value in Bash
We also can perform the same task using double square brackets [[]]
. This section will show how we can check a variable for value through double square brackets using the example code shared below.
VAR=
[[ x"${VAR}" == "x" ]] && echo "No value found for the variable" || echo "Value found for the variable"
In the example above, through the line [[ x"${VAR}" == "x" ]]
, we checked whether the variable contains a value. Now, when you execute this code, you will get an output like the following:
No value found for the variable
Use the Parameter Expression to Check if a Variable Has a Value in Bash
Another way to check whether the variable contains a value is using the Parameter Expression.
This section will use the built-in Parameter Expression to check a variable. For this purpose, you can follow the below example:
VAR=
[[ ${VAR:-"unset"} == "unset" ]] && echo "No value found for the variable" || echo "Value found for the variable"
On the example above, through the line [[ ${VAR:-"unset"} == "unset" ]]
, we checked whether the variable contains value.
Now, when you execute the code above, you will get an output like the following:
No value found for the variable
Use the -z
Conditional Expression to Check if a Variable Has a Value in Bash
In Bash scripting, there is a -z
conditional expression, which we can use to check whether a variable contains a value or not. It returns true
if the string length is 0.
To check if a variable contains a value or not through this method, you can follow the below example code:
var=
[[ -z "${var}" ]] && echo "No value was found for the variable !!!" || echo "Value found for the variable..."
In the example above, through the line [[ -z "${VAR}" ]]
, we checked whether the variable contains a value.
When you execute the code above, you will get the output below:
No value was found for the variable !!!
Use the -n
Conditional Expression to Check if a Variable Has a Value in Bash
Also, Bash has a built-in -n
conditional expression that we can use for checking the variable.
The -n
conditional expression returns true if the string length is a non-zero value. We can also use this method to check if the variable contains a value or not.
You can follow the example code shared below:
VAR=
[[ ! -n "${VAR}" ]] && echo "No value found for the variable" || echo "Value found for the variable"
In the example above, through the line [[ ! -n "${VAR}" ]]
, we checked whether the variable contains a value.
Now, when you execute this code, you will get an output like the one below:
No value found for the variable
You can select any of the above methods to check your variable based on your needs and complexity.
Please note that all the code used in this article is written in Bash. It will only be runnable in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn