How to Use of the Secondary Constructors in Kotlin
- The History of Secondary Constructors in Kotlin
- Kotlin Secondary Constructors
- Create Multiple Secondary Constructors in Kotlin
- Call a Secondary Constructor From Another Existing Secondary Constructor
- Call a Secondary Constructor From Parent Class From a Secondary Constructor in the Child Class
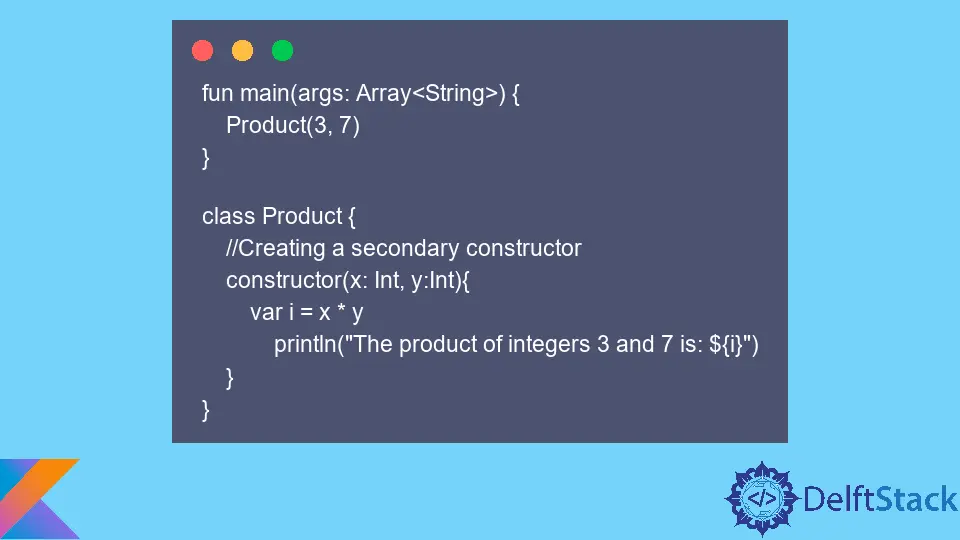
Constructors are member functions automatically called when an object is created in an object-oriented programming language.
Since Kotlin is an OOPS language, it also uses constructors for object initialization. In Kotlin, there are two constructors: Primary constructors and Secondary constructors.
This article introduces the concept of secondary constructors in Kotlin and how to use them.
The History of Secondary Constructors in Kotlin
The secondary constructors were not supported in Kotlin until 2015. Until then, developers used one of the blow techniques to solve most of the secondary constructor use cases.
- Defining a factory method next to the class.
- Defining default values for parameters.
- Using a factory method defined in another object.
While these techniques were good enough, there was a need for secondary constructors. Kotlin started supporting secondary constructors with the launch of M11 in 2015.
Kotlin Secondary Constructors
While primary constructors provide a concise way to initialize a class, the secondary constructors become necessary while extending them. Moreover, the secondary constructor also allows adding initialization logic.
While we can have only one primary constructor in a program, Kotlin enables the creation of multiple secondary constructors.
We can create and use secondary constructors in Kotlin with the constructor
keyword. A Kotlin program can have one or multiple secondary constructors.
Secondary constructors are generally avoided, but they are used to extend a class. The reason is that they help initialize a single class in multiple ways through multiple constructors.
Syntax:
class ABC {
constructor(data: String) {
// code
}
To understand the secondary constructors better, let’s look at the following example. We will create a secondary constructor for class Product
that will output the product of two integers.
Example Code:
fun main(args: Array<String>) {
Product(3, 7)
}
class Product {
//Creating a secondary constructor
constructor(x: Int, y:Int){
var i = x * y
println("The product of integers 3 and 7 is: ${i}")
}
}
Output:
Create Multiple Secondary Constructors in Kotlin
We can also have multiple constructors in Kotlin. Which of the many constructors the compiler calls is decided based on the arguments passed.
Example Code:
fun main(args: Array<String>) {
Student(11035, "David")
Student(11047,"Steve","New York")
}
class Student {
constructor (std_id : Int, std_name: String ) {
var id: Int = std_id
var name: String = std_name
print("Student's ID is: $id, ")
println("Student's Name is: $name")
println()
}
constructor (std_id : Int, std_name: String, std_city: String) {
var id: Int = std_id
var name: String = std_name
var city: String = std_city
print("Student's ID is: $id, ")
print("Student's Name is: $name, ")
println("Student's City is: $city")
}
}
Output:
Call a Secondary Constructor From Another Existing Secondary Constructor
We can call a secondary constructor in Kotlin using an existing secondary constructor. We need to use the this()
function.
Example Code:
fun main(args: Array<String>){
Product(3,7)
}
class Product {
// using this to call another secondary constructor
constructor(x: Int,y:Int) : this(x,y,5) {
var ProductOfTwo = x * y
println("The product of the two integers is: $ProductOfTwo")
}
constructor(x: Int, y: Int,z: Int) {
var ProductOfThree = x * y * z
println("The product of the three integers is is: $ProductOfThree")
}
}
Output:
Call a Secondary Constructor From Parent Class From a Secondary Constructor in the Child Class
Besides calling a secondary constructor from another, we can also call a parent class’s secondary constructor from the child class’s constructor. It is similar to inheritance.
We need to use the super
keyword for inheriting the constructor.
Example Code:
fun main(args: Array<String>) {
childClass(11035, "David")
}
open class parentClass {
constructor (std_id : Int, std_name: String, std_city: String) {
var id: Int = std_id
var name: String = std_name
var city: String = std_city
print("Student's ID is: $id, ")
print("Student's Name is: $name, ")
println("Student's City is: $city")
println()
}
}
class childClass : parentClass {
constructor (std_id : Int, std_name: String):super(std_id,std_name,"New York"){
var id: Int = std_id
var name: String = std_name
print("Student's ID is: $id, ")
println("Student's Name is: $name")
}
}
Output:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn