Kotlin Private Constructor
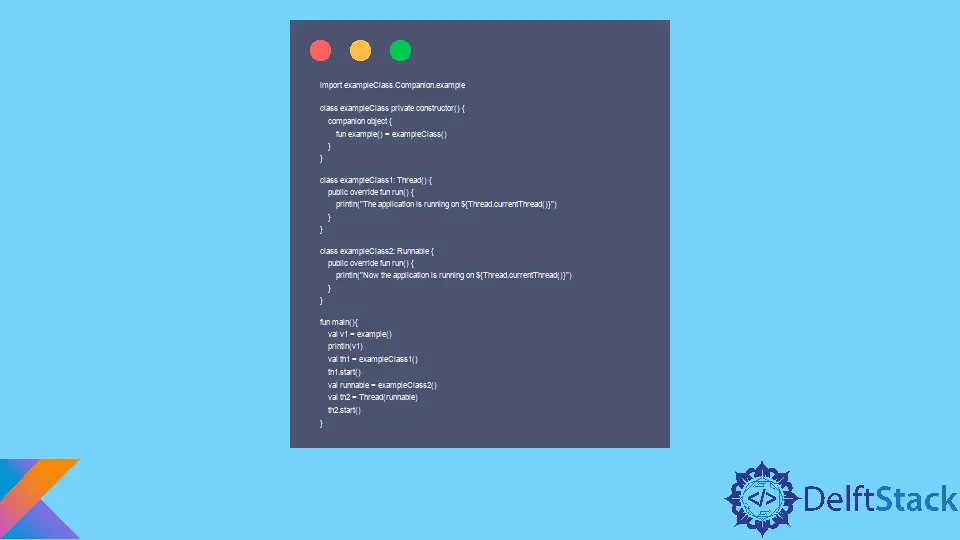
We can define a primary constructor in Java programming as private
. We can then access the constructor using a public
static
method. However, is that possible in Kotlin?
Kotlin, too, allows the creation of private
constructors. How? That we will see in this article.
Kotlin private
Constructor
Constructors are special member functions of a Kotlin class
. They are invoked when we create and initiate an object.
Kotlin private
constructors are helpful to enable custom visibility into the class
; it will also hide the primary constructor of the Kotlin class
. For example, suppose we want to prevent an unwanted object creation for memory efficiency. We can use private
constructors to achieve that.
We can define a Kotlin constructor as private
using the access modifier keyword private
and a companion object. Class members can access companion objects’ private
members.
The syntax of declaring a constructor as private is:
class class_Name private constructor()
Working of private
Constructors
private
constructors work by making the primary constructor of your class private
. It prevents other classes from creating objects using the private
constructor.
When creating a private
constructor, it is best to use a static
method. The reason is that static
methods can run without object initialization.
Now, the private
constructors will allow creating and initializing objects from the same class. However, when you try to initialize objects from other classes, it will throw an error.
Pros and Cons of private
Constructors
Let’s start with exploring the advantages of private
constructors in Kotlin.
The first benefit of using a private
constructor is security. Since no other classes can access the data of the private
class, it becomes secure.
Memory efficiency is also an advantage of using private
constructors means that only a single instance is created. Moreover, it can also prevent the automatic generation of a default constructor.
Coming to the disadvantages (cons), private
constructors do not allow inheritance. Additionally, if we want to initialize a private
constructor class, we need to use a nested class or a static
method.
Demonstration of a Kotlin private
Constructor
Here’s an example where we use the above syntax to create a class with a private
constructor.
import exampleClass.Companion.example
class exampleClass private constructor() {
companion object {
fun example() = exampleClass()
}
}
class exampleClass1: Thread() {
public override fun run() {
println("The application is running on ${Thread.currentThread()}")
}
}
class exampleClass2: Runnable {
public override fun run() {
println("Now the application is running on ${Thread.currentThread()}")
}
}
fun main(){
val v1 = example()
println(v1)
val th1 = exampleClass1()
th1.start()
val runnable = exampleClass2()
val th2 = Thread(runnable)
th2.start()
}
Output:
exampleClass@77afea7d
The application is running on Thread[Thread-0,5, main]
Now the application is running on Thread[Thread-1,5, main]
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn