Difference Between Kotlin Init and Constructors
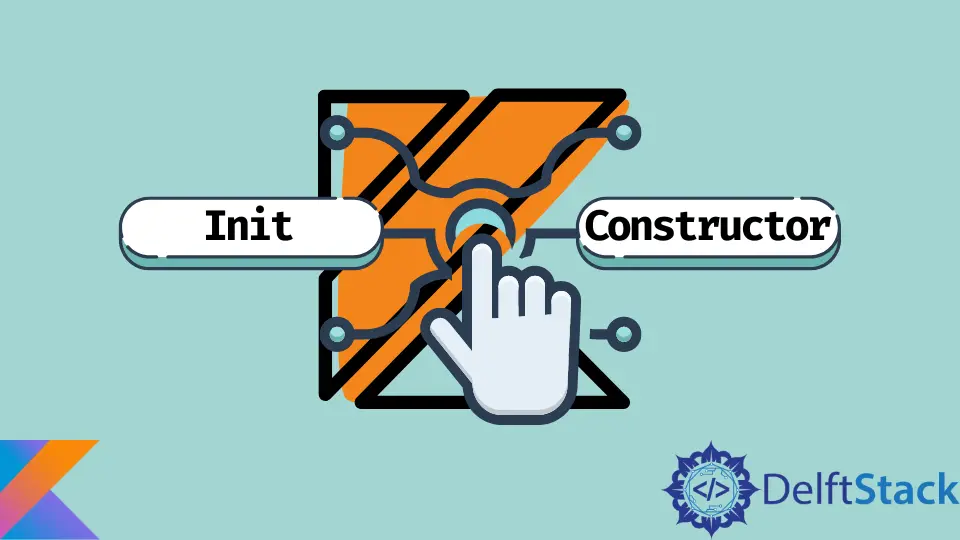
Kotlin is an object-oriented programming (OOP) language. Constructors and init
blocks are vital components of this Android programming language.
This article will look at the difference between these two components.
Kotlin Constructors
As the name gives out, Kotlin constructors help in constructing classes. Classes are the base of an OOP language.
In Kotlin, there are two types of constructors, primary and secondary. Each class can have one primary and any number of secondary constructors defined using the constructor
keyword.
Kotlin Primary Constructors
The primary constructor in Kotlin is always a part of the class header. It can have optional parameters.
class exampleClass public constructor(str: String, i: Int){
//functions
}
You might wonder, if every class header has a primary constructor, why are many classes declared without using the constructor
keyword.
You can omit the keyword if you don’t want to use access modifiers or annotations with the primary constructor.
class exampleClass (str: String, i: Int){
//functions
}
Since the primary constructor is a part of the class header, it cannot have any code. We can put the code in the initializer blocks using the init
keyword, more about that later in this article.
While writing a code is not allowed, we can declare and initialize primary constructor properties using these syntaxes:
class exampleClass (val str: String, var i: Int){
//functions
}
OR
class exampleClass (
val str: String,
var i: Int,
){
//functions
}
We can also declare the default value of a property in the primary constructor:
class exampleClass (val str: String, var i: Int = 15){
//functions
}
Kotlin Secondary Constructors
Apart from the primary constructor, we can also declare one or multiple secondary constructors using the constructor
keyword.
All the secondary constructors of a class should delegate to the primary one using the this
keyword. Here’s an example of using the secondary constructor in Kotlin.
fun main(args: Array<String>){
var Stu = Student("Zack",17, "Kotlin")
Stu.printStudentDetails()
}
// This is the primary constructor
class Student constructor(var firstName: String, var age: Int){
var expert: String = "Nothing"
// Creating a secondary constructor
constructor (firstName: String, age: Int, expert: String): this(firstName,age){
this.expert = expert
}
fun printStudentDetails(){
println("$firstName, a $age years old student, is an expert in $expert.")
}
}
Output:
Kotlin init
The Kotlin init
keyword is used to write initializer blocks. These blocks help write codes for the primary constructor.
We cannot write code in the primary constructor as it is a part of the class header. Hence, we can use a secondary constructor or initializer blocks for that.
We can also use the primary constructor’s parameters and properties in the Kotlin init
blocks.
Let’s look at an example where we check if the given number is greater than 50 or not using the Kotlin init
block.
class Numbers(firstName: String, num2: Int) {
var res = false
val n1 = 50
init {
res = num2 > n1
}
}
fun main(){
var numbers = Numbers("This number is greater than 50", 63)
println(numbers.res)
}
Output:
Since the codes in Kotlin init
blocks are written as a part of the primary constructor, they become a part of it.
And since the secondary constructor’s delegation with the primary constructor is the first thing done during the former’s execution, the init
blocks are executed before the secondary constructors’ functions.
Here’s an example code. In this code, we have written the secondary constructor code above Kotlin init
; however, the init
code will execute first.
class Person {
constructor(i: Int) {
println("Person $i")
}
init {
println("This part will execute before the secondary constructor's code.")
}
}
fun main() {
Person(1)
}
Output:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn