Kotlin 中二級建構函式的使用
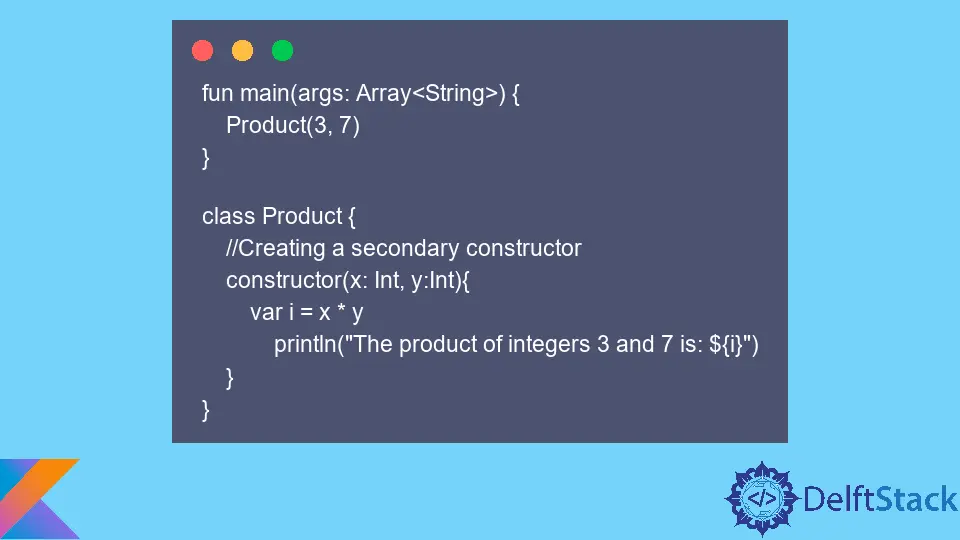
建構函式是在物件導向的程式語言中建立物件時自動呼叫的成員函式。
由於 Kotlin 是一種 OOPS 語言,因此它還使用建構函式進行物件初始化。在 Kotlin 中,有兩個建構函式:主建構函式和輔助建構函式。
本文介紹了 Kotlin 中二級建構函式的概念以及如何使用它們。
Kotlin 二次建構函式的歷史
直到 2015 年,Kotlin 才支援輔助建構函式。在此之前,開發人員使用其中一種打擊技術來解決大多數輔助建構函式用例。
- 在類旁邊定義一個工廠方法。
- 定義引數的預設值。
- 使用在另一個物件中定義的工廠方法。
儘管這些技術已經足夠好,但仍需要輔助建構函式。隨著 2015 年 M11 的推出,Kotlin 開始支援二級建構函式。
Kotlin 二級建構函式
雖然主建構函式提供了一種簡潔的方式來初始化一個類,但在擴充套件它們時,輔助建構函式變得很有必要。此外,輔助建構函式還允許新增初始化邏輯。
雖然我們在一個程式中只能有一個主建構函式,但 Kotlin 允許建立多個輔助建構函式。
我們可以通過 constructor
關鍵字在 Kotlin 中建立和使用二級建構函式。一個 Kotlin 程式可以有一個或多個輔助建構函式。
通常避免使用輔助建構函式,但它們用於擴充套件類。原因是它們通過多個建構函式以多種方式幫助初始化單個類。
語法:
class ABC {
constructor(data: String) {
// code
}
為了更好地理解二級建構函式,讓我們看下面的例子。我們將為類 Product
建立一個輔助建構函式,它將輸出兩個整數的乘積。
示例程式碼:
fun main(args: Array<String>) {
Product(3, 7)
}
class Product {
//Creating a secondary constructor
constructor(x: Int, y:Int){
var i = x * y
println("The product of integers 3 and 7 is: ${i}")
}
}
輸出:
在 Kotlin 中建立多個輔助建構函式
我們還可以在 Kotlin 中擁有多個建構函式。編譯器呼叫的許多建構函式中的哪一個是根據傳遞的引數決定的。
示例程式碼:
fun main(args: Array<String>) {
Student(11035, "David")
Student(11047,"Steve","New York")
}
class Student {
constructor (std_id : Int, std_name: String ) {
var id: Int = std_id
var name: String = std_name
print("Student's ID is: $id, ")
println("Student's Name is: $name")
println()
}
constructor (std_id : Int, std_name: String, std_city: String) {
var id: Int = std_id
var name: String = std_name
var city: String = std_city
print("Student's ID is: $id, ")
print("Student's Name is: $name, ")
println("Student's City is: $city")
}
}
輸出:
從另一個現有的輔助建構函式呼叫輔助建構函式
我們可以使用現有的輔助建構函式在 Kotlin 中呼叫輔助建構函式。我們需要使用 this()
函式。
示例程式碼:
fun main(args: Array<String>){
Product(3,7)
}
class Product {
// using this to call another secondary constructor
constructor(x: Int,y:Int) : this(x,y,5) {
var ProductOfTwo = x * y
println("The product of the two integers is: $ProductOfTwo")
}
constructor(x: Int, y: Int,z: Int) {
var ProductOfThree = x * y * z
println("The product of the three integers is is: $ProductOfThree")
}
}
輸出:
從子類中的輔助建構函式呼叫父類的輔助建構函式
除了從另一個呼叫輔助建構函式外,我們還可以從子類的建構函式呼叫父類的輔助建構函式。它類似於繼承。
我們需要使用 super
關鍵字來繼承建構函式。
示例程式碼:
fun main(args: Array<String>) {
childClass(11035, "David")
}
open class parentClass {
constructor (std_id : Int, std_name: String, std_city: String) {
var id: Int = std_id
var name: String = std_name
var city: String = std_city
print("Student's ID is: $id, ")
print("Student's Name is: $name, ")
println("Student's City is: $city")
println()
}
}
class childClass : parentClass {
constructor (std_id : Int, std_name: String):super(std_id,std_name,"New York"){
var id: Int = std_id
var name: String = std_name
print("Student's ID is: $id, ")
println("Student's Name is: $name")
}
}
輸出:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn