Kotlin에서 보조 생성자 사용
- Kotlin의 보조 생성자의 역사
- Kotlin 보조 생성자
- Kotlin에서 여러 보조 생성자 만들기
- 다른 기존 보조 생성자에서 보조 생성자 호출
- 자식 클래스의 보조 생성자에서 부모 클래스의 보조 생성자 호출
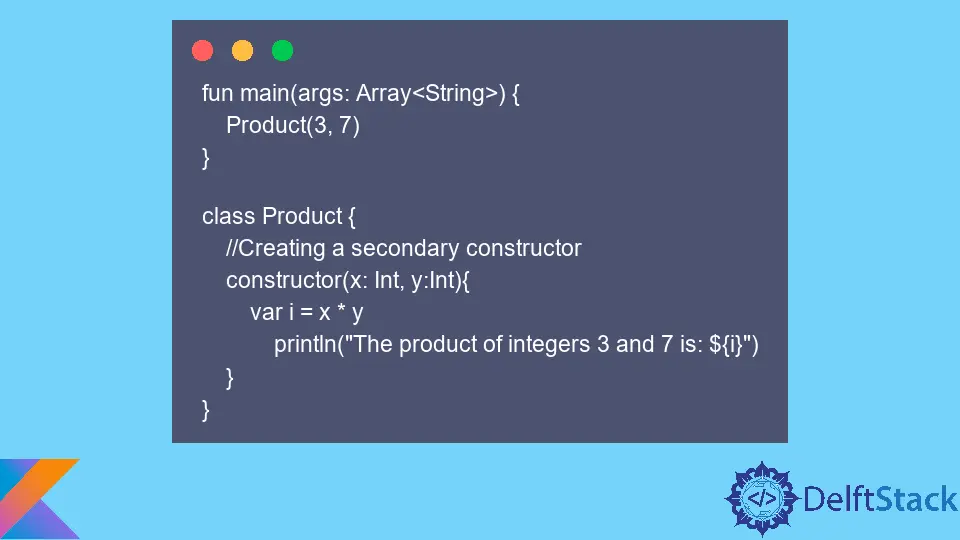
생성자는 객체 지향 프로그래밍 언어로 객체를 생성할 때 자동으로 호출되는 멤버 함수입니다.
Kotlin은 OOPS 언어이므로 객체 초기화에도 생성자를 사용합니다. Kotlin에는 기본 생성자와 보조 생성자의 두 가지 생성자가 있습니다.
이 문서에서는 Kotlin의 보조 생성자의 개념과 사용 방법을 소개합니다.
Kotlin의 보조 생성자의 역사
보조 생성자는 2015년까지 Kotlin에서 지원되지 않았습니다. 그때까지 개발자는 타격 기술 중 하나를 사용하여 대부분의 보조 생성자 사용 사례를 해결했습니다.
- 클래스 옆에 팩토리 메소드 정의.
- 매개변수의 기본값 정의.
- 다른 객체에 정의된 팩토리 메소드 사용.
이러한 기술은 충분했지만 보조 생성자가 필요했습니다. Kotlin은 2015년 M11 출시와 함께 보조 생성자를 지원하기 시작했습니다.
Kotlin 보조 생성자
기본 생성자는 클래스를 초기화하는 간결한 방법을 제공하지만 보조 생성자는 클래스를 확장하는 동안 필요하게 됩니다. 또한 보조 생성자는 초기화 논리를 추가할 수도 있습니다.
프로그램에는 기본 생성자가 하나만 있을 수 있지만 Kotlin에서는 여러 개의 보조 생성자를 만들 수 있습니다.
constructor
키워드를 사용하여 Kotlin에서 보조 생성자를 만들고 사용할 수 있습니다. Kotlin 프로그램은 하나 이상의 보조 생성자를 가질 수 있습니다.
보조 생성자는 일반적으로 피하지만 클래스를 확장하는 데 사용됩니다. 그 이유는 여러 생성자를 통해 단일 클래스를 여러 방법으로 초기화하는 데 도움이 되기 때문입니다.
통사론:
class ABC {
constructor(data: String) {
// code
}
보조 생성자를 더 잘 이해하기 위해 다음 예제를 살펴보겠습니다. 두 정수의 곱을 출력하는 Product
클래스에 대한 보조 생성자를 생성합니다.
예제 코드:
fun main(args: Array<String>) {
Product(3, 7)
}
class Product {
//Creating a secondary constructor
constructor(x: Int, y:Int){
var i = x * y
println("The product of integers 3 and 7 is: ${i}")
}
}
출력:
Kotlin에서 여러 보조 생성자 만들기
Kotlin에는 여러 생성자가 있을 수도 있습니다. 많은 생성자 중 컴파일러가 호출하는 생성자는 전달된 인수에 따라 결정됩니다.
예제 코드:
fun main(args: Array<String>) {
Student(11035, "David")
Student(11047,"Steve","New York")
}
class Student {
constructor (std_id : Int, std_name: String ) {
var id: Int = std_id
var name: String = std_name
print("Student's ID is: $id, ")
println("Student's Name is: $name")
println()
}
constructor (std_id : Int, std_name: String, std_city: String) {
var id: Int = std_id
var name: String = std_name
var city: String = std_city
print("Student's ID is: $id, ")
print("Student's Name is: $name, ")
println("Student's City is: $city")
}
}
출력:
다른 기존 보조 생성자에서 보조 생성자 호출
기존 보조 생성자를 사용하여 Kotlin에서 보조 생성자를 호출할 수 있습니다. this()
함수를 사용해야 합니다.
예제 코드:
fun main(args: Array<String>){
Product(3,7)
}
class Product {
// using this to call another secondary constructor
constructor(x: Int,y:Int) : this(x,y,5) {
var ProductOfTwo = x * y
println("The product of the two integers is: $ProductOfTwo")
}
constructor(x: Int, y: Int,z: Int) {
var ProductOfThree = x * y * z
println("The product of the three integers is is: $ProductOfThree")
}
}
출력:
자식 클래스의 보조 생성자에서 부모 클래스의 보조 생성자 호출
다른 생성자에서 보조 생성자를 호출하는 것 외에도 자식 클래스의 생성자에서 부모 클래스의 보조 생성자를 호출할 수도 있습니다. 상속과 비슷합니다.
생성자를 상속받기 위해서는 super
키워드를 사용해야 합니다.
예제 코드:
fun main(args: Array<String>) {
childClass(11035, "David")
}
open class parentClass {
constructor (std_id : Int, std_name: String, std_city: String) {
var id: Int = std_id
var name: String = std_name
var city: String = std_city
print("Student's ID is: $id, ")
print("Student's Name is: $name, ")
println("Student's City is: $city")
println()
}
}
class childClass : parentClass {
constructor (std_id : Int, std_name: String):super(std_id,std_name,"New York"){
var id: Int = std_id
var name: String = std_name
print("Student's ID is: $id, ")
println("Student's Name is: $name")
}
}
출력:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn