Kotlin 中二级构造函数的使用
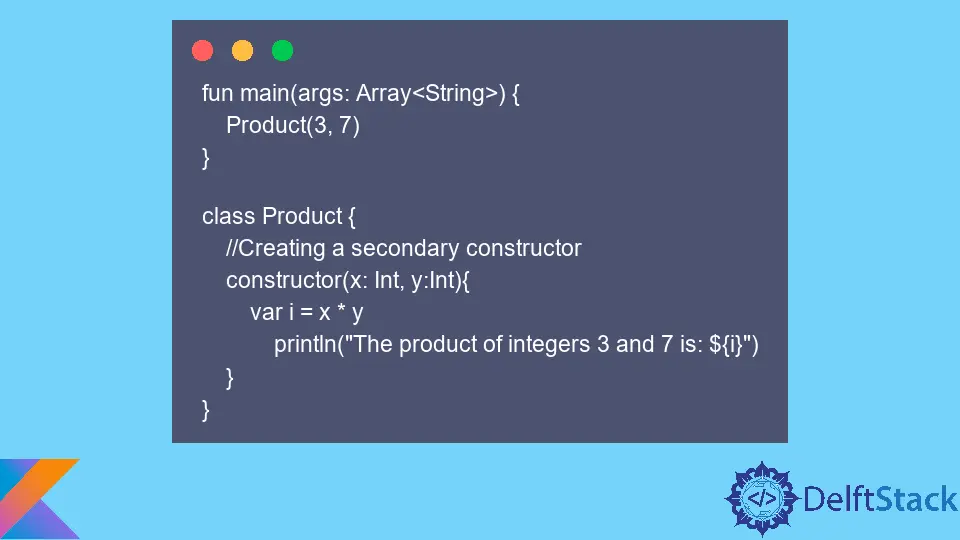
构造函数是在面向对象的编程语言中创建对象时自动调用的成员函数。
由于 Kotlin 是一种 OOPS 语言,因此它还使用构造函数进行对象初始化。在 Kotlin 中,有两个构造函数:主构造函数和辅助构造函数。
本文介绍了 Kotlin 中二级构造函数的概念以及如何使用它们。
Kotlin 二次构造函数的历史
直到 2015 年,Kotlin 才支持辅助构造函数。在此之前,开发人员使用其中一种打击技术来解决大多数辅助构造函数用例。
- 在类旁边定义一个工厂方法。
- 定义参数的默认值。
- 使用在另一个对象中定义的工厂方法。
尽管这些技术已经足够好,但仍需要辅助构造函数。随着 2015 年 M11 的推出,Kotlin 开始支持二级构造函数。
Kotlin 二级构造函数
虽然主构造函数提供了一种简洁的方式来初始化一个类,但在扩展它们时,辅助构造函数变得很有必要。此外,辅助构造函数还允许添加初始化逻辑。
虽然我们在一个程序中只能有一个主构造函数,但 Kotlin 允许创建多个辅助构造函数。
我们可以通过 constructor
关键字在 Kotlin 中创建和使用二级构造函数。一个 Kotlin 程序可以有一个或多个辅助构造函数。
通常避免使用辅助构造函数,但它们用于扩展类。原因是它们通过多个构造函数以多种方式帮助初始化单个类。
语法:
class ABC {
constructor(data: String) {
// code
}
为了更好地理解二级构造函数,让我们看下面的例子。我们将为类 Product
创建一个辅助构造函数,它将输出两个整数的乘积。
示例代码:
fun main(args: Array<String>) {
Product(3, 7)
}
class Product {
//Creating a secondary constructor
constructor(x: Int, y:Int){
var i = x * y
println("The product of integers 3 and 7 is: ${i}")
}
}
输出:
在 Kotlin 中创建多个辅助构造函数
我们还可以在 Kotlin 中拥有多个构造函数。编译器调用的许多构造函数中的哪一个是根据传递的参数决定的。
示例代码:
fun main(args: Array<String>) {
Student(11035, "David")
Student(11047,"Steve","New York")
}
class Student {
constructor (std_id : Int, std_name: String ) {
var id: Int = std_id
var name: String = std_name
print("Student's ID is: $id, ")
println("Student's Name is: $name")
println()
}
constructor (std_id : Int, std_name: String, std_city: String) {
var id: Int = std_id
var name: String = std_name
var city: String = std_city
print("Student's ID is: $id, ")
print("Student's Name is: $name, ")
println("Student's City is: $city")
}
}
输出:
从另一个现有的辅助构造函数调用辅助构造函数
我们可以使用现有的辅助构造函数在 Kotlin 中调用辅助构造函数。我们需要使用 this()
函数。
示例代码:
fun main(args: Array<String>){
Product(3,7)
}
class Product {
// using this to call another secondary constructor
constructor(x: Int,y:Int) : this(x,y,5) {
var ProductOfTwo = x * y
println("The product of the two integers is: $ProductOfTwo")
}
constructor(x: Int, y: Int,z: Int) {
var ProductOfThree = x * y * z
println("The product of the three integers is is: $ProductOfThree")
}
}
输出:
从子类中的辅助构造函数调用父类的辅助构造函数
除了从另一个调用辅助构造函数外,我们还可以从子类的构造函数调用父类的辅助构造函数。它类似于继承。
我们需要使用 super
关键字来继承构造函数。
示例代码:
fun main(args: Array<String>) {
childClass(11035, "David")
}
open class parentClass {
constructor (std_id : Int, std_name: String, std_city: String) {
var id: Int = std_id
var name: String = std_name
var city: String = std_city
print("Student's ID is: $id, ")
print("Student's Name is: $name, ")
println("Student's City is: $city")
println()
}
}
class childClass : parentClass {
constructor (std_id : Int, std_name: String):super(std_id,std_name,"New York"){
var id: Int = std_id
var name: String = std_name
print("Student's ID is: $id, ")
println("Student's Name is: $name")
}
}
输出:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn