How to Pass Request Headers in jQuery AJAX
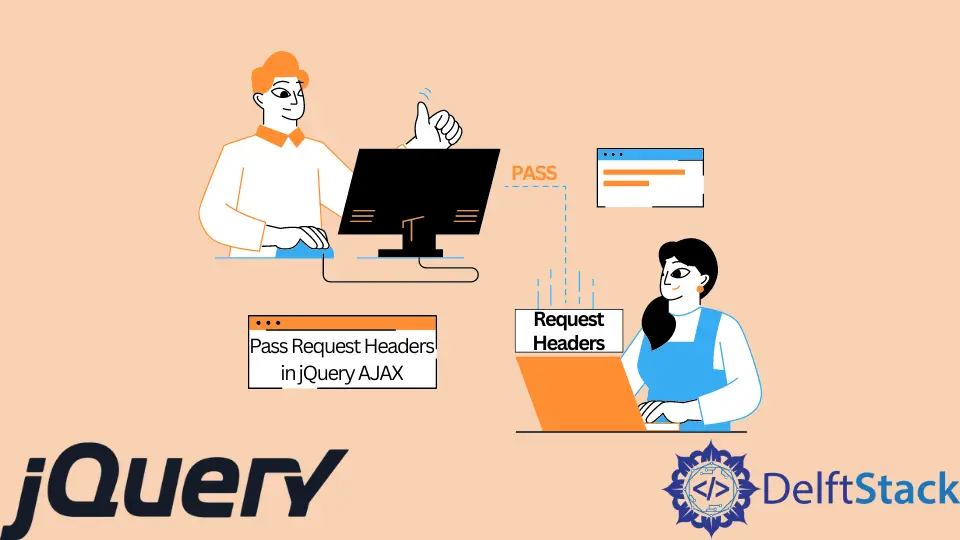
The headers specify the kind of response accepted from the server in jQuery AJAX. This tutorial demonstrates how to use the headers in jQuery AJAX.
Pass Request Headers in jQuery AJAX
As mentioned above, the headers can specify the kind of response accepted from the server in jQuery AJAX. A header is a built-in option passed to the ajax()
method in jQuery.
The headers are key-value pairs sent in the AJAX request using the XMLHttpRequest
object. An asynchronous HTTP request and a header will inform the server what kind of response to accept.
A callback function can also be used to set the header properties.
The syntax for the jQuery AJAX header is:
$.ajax({ headers : { key : value }})
The syntax for the beforeSend
callback function to overwrite header properties is:
$.ajax({beforeSend: function (jqXHR, settings) { jqXHR.setRequestHeader(key, value) );}})
Where headers : { key : value }
is an optional option. It will specify the type of response accepted from the server while sending the request to the server.
The default value for headers is {}
, which is a plain object type. The keys for headers are Accept
, Accept-Encoding
, Accept-Language
, Connection
, Cookie
, User-Agent
, Host
, and Order-Number
, etc.
And beforeSend
is an optional function that will set or overwrite to specify the type of response accepted from the server. It will accept jqXHR
, and the settings
parameters will modify the jqXHR
object and add a custom header using the setRequestHeader
method.
Let’s try a simple example with AJAX headers to get the data from the server:
<!doctype html>
<html lang = "en">
<head>
<meta charset = "utf-8">
<script type = "text/javascript" src = "https://ajax.googleapis.com/ajax/libs/jquery/1.11.2/jquery.min.js"></script>
<title> jQuery Ajax headers </title>
</head>
<body>
<h3> jQuery ajax headers : </h3>
<button id = "Send_Request" > Send the ajax request with headers <button/>
<br>
<p id = "para1" style = "color : green"> </p>
<script type = "text/javascript">
$(document).ready( function () {
$('#Send_Request').click( function(){
var Ajax_Request = $.ajax( { url : 'http://localhost/',
contentType : 'application/json',
dataType : 'json',
headers: {"Accepts": "text/plain; charset=utf-8"}
});
ajxReq.success( function ( Request_Data, Request_Status, jqXHR ) {
$( '#para1' ).append( '<h2> The JSON data: </h2>');
$( '#para1' ).append( '<p> The Request Date : ' + Request_Data.date + '</p>');
$( '#para1' ).append ('<p> The Request Time: ' + Request_Data.time + '</p>');
$( '#para1' ).append( '<p> The Request Status : ' + Request_Status + '</p>');
});
Ajax_Request.error( function ( jqXHR, Text_Status, Error_Message ) {
$( "p" ).append( "The status is :" +Text_Status);
});
});
});
</script>
</body>
</html>
The code above will try to send an AJAX request with headers options "Accepts": "text/plain; charset=utf-8"
. See the output:
As shown in the output, it throws an error because, in the header, we request plain text from the server, but the received data is JSON, so it throws an error. To successfully process the request, we must set the header value as JSON.
Let’s try to correct the example:
<!doctype html>
<html lang = "en">
<head>
<meta charset = "utf-8">
<script type = "text/javascript" src = "https://ajax.googleapis.com/ajax/libs/jquery/1.11.2/jquery.min.js"></script>
<title> jQuery Ajax headers </title>
</head>
<body>
<h3> jQuery ajax headers : </h3>
<button id = "Send_Request" > Send the ajax request with headers <button/>
<br>
<p id = "para1" style = "color : green"> </p>
<script type = "text/javascript">
$(document).ready( function () {
$('#Send_Request').click( function(){
// url from where we want to get the data
var ajxReq = $.ajax( { url : 'http://time.jsontest.com',
contentType : 'application/json',
dataType : 'json',
headers: {"Accept": "application/json"}
});
ajxReq.success( function ( Request_Data, Request_Status, jqXHR ) {
$( '#para1' ).append( '<h2> The JSON data: </h2>');
$( '#para1' ).append( '<p> The Request Date : ' + Request_Data.date + '</p>');
$( '#para1' ).append ('<p> The Request Time: ' + Request_Data.time + '</p>');
$( '#para1' ).append( '<p> The Request Status : ' + Request_Status + '</p>');
});
ajxReq.error( function ( jqXHR, Text_Status, Error_Message ) {
$( "p" ).append( "The status is : " + Text_Status);
});
});
});
</script>
</body>
</html>
The code above will send an AJAX request with headers value JSON, and the code will get the JSON data back from the server. See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook