AJAX POST Request in jQuery
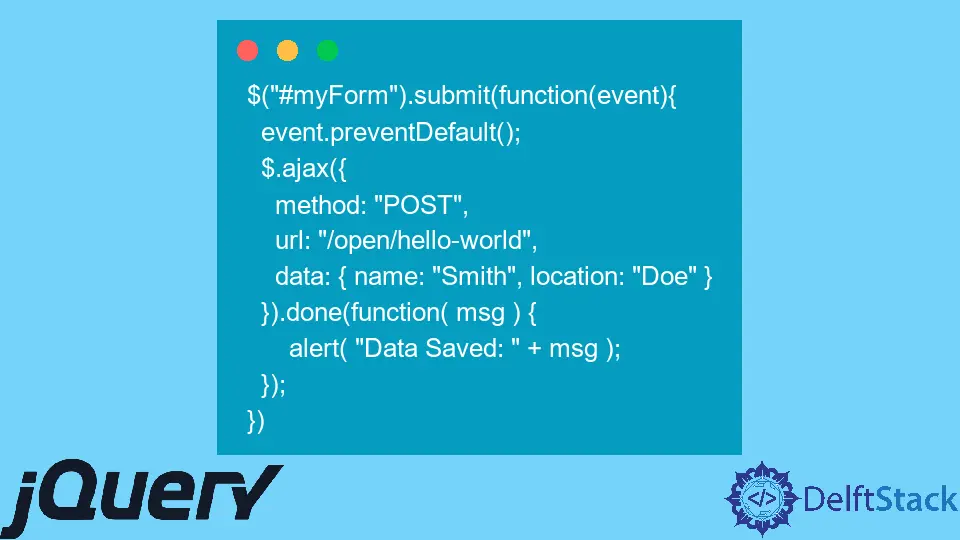
Today’s post will teach about AJAX POST
requests in jQuery.
AJAX POST
Request in jQuery
jQuery’s AJAX POST
request perform an asynchronous HTTP (AJAX) request.
Syntax:
jQuery.ajax(url[, settings])
jQuery.ajax([settings])
URL
is the string containing the URL to which the request will be sent.settings
are the key/value pair objects that configure the AJAX request. All settings are optional. A default value can be set for each option with$.ajaxSetup()
.
The $.ajax()
function underlies all AJAX requests dispatched by way of jQuery. It is often useless to call this characteristic at once, as numerous higher-level options like $.Get()
and .load()
are available and simpler to apply.
Commonplace options are required if much less, although $.ajax()
may be used more flexibly.
The jQuery XMLHttpRequest
(jqXHR
) object returned via $.ajax()
as of jQuery 1.5 is a superset of the browser-local XMLHttpRequest
element. For instance, it contains responseText
and responseXML
houses, as well as a getResponseHeader()
technique.
While the delivery mechanism is something apart from XMLHttpRequest
(for instance, a script tag for a JSONP
request), the jqXHR
item simulates native XHR capability where feasible.
As of jQuery 1.5.1, the jqXHR
object additionally carries the overrideMimeType()
method (it changed in jQuery 1.4.X, as nicely, but become briefly eliminated in jQuery 1.5). For example, the .overrideMimeType()
technique can be used inside the beforeSend()
callback function to change the response’s Content-Type header.
Distinct responses to $.Ajax()
calls are subjected to specific types of pre-processing earlier than being handed to the success handler. The available information kinds are text
, HTML
, XML
, JSON
, JSONP
, and script
.
The data option can either send the data in a query string of the form key1=value1&key2=value2
or an object of the form {key1: 'value1', key2: 'value2'}
.
Due to browser safety restrictions, most AJAX requests are subject to the same-origin coverage; the request can’t efficaciously retrieve facts from a different domain, subdomain, port, or protocol.
Find more information in the .ajax()
documentation.
Let’s understand it with the following example. The following codes cannot be run as-is and have no output.
It must be added to the existing code to see the outputs.
<form id="myForm">
<label for="name">Name</label>
<input id="name" name="name" type="text" value="Smith" />
<input type="submit" value="Send" />
</form>
$('#myForm').submit(function(event) {
event.preventDefault();
$.ajax({
method: 'POST',
url: '/open/hello-world',
data: {name: 'Smith', location: 'Doe'}
}).done(function(msg) {
alert('Data Saved: ' + msg);
});
})
Once the user submits the form in the example above, an AJAX call is sent to the server with the specified URL and parameters. When the server returns with a successful message, you can print the message on the console or notify the user with the appropriate message.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn