How to Handle $.ajax Failure in jQuery
- Understanding AJAX Failures
- Using the .fail() Method
- Implementing Error Handling with .always()
- Customizing Error Responses
- Conclusion
- FAQ
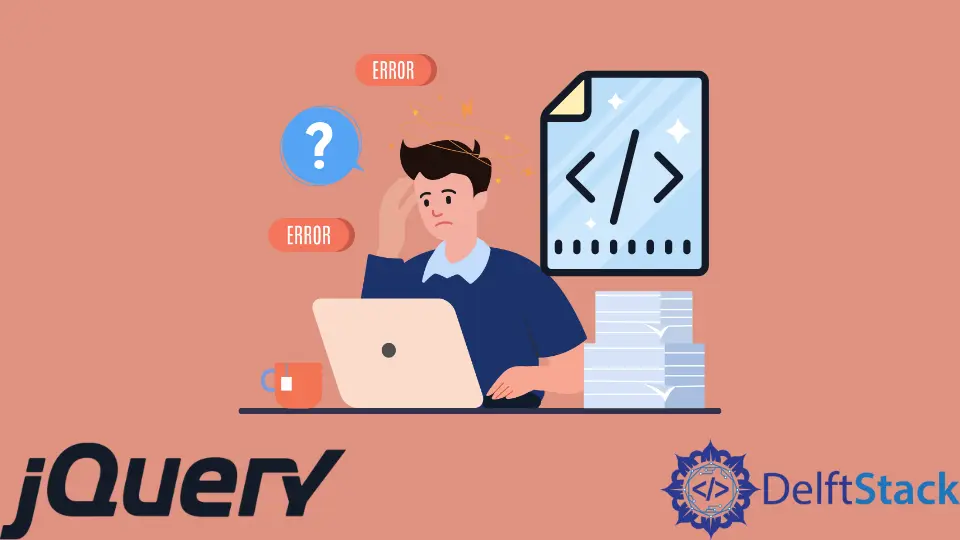
In today’s post, we’ll learn about handling failure requests in AJAX in jQuery. AJAX (Asynchronous JavaScript and XML) is a powerful tool that allows web applications to communicate with servers asynchronously, enhancing user experience by loading data in the background. However, not every request goes smoothly, and handling failures is crucial for maintaining a seamless user experience.
In this article, we’ll explore the various ways to effectively manage AJAX failures using jQuery. We’ll discuss common failure scenarios, how to implement error handling, and provide practical code examples to help you grasp these concepts easily. Whether you’re a beginner or looking to refine your skills, this guide will equip you with the knowledge to handle AJAX failures gracefully.
Understanding AJAX Failures
Before diving into the solutions, it’s essential to understand what can cause AJAX failures. Various factors can lead to unsuccessful requests, including:
- Network issues
- Server errors (like 404 or 500)
- Cross-origin resource sharing (CORS) problems
- Incorrect URL or request parameters
Understanding these causes will help you anticipate potential issues and implement effective error handling strategies.
Using the .fail() Method
One of the simplest ways to handle AJAX failures in jQuery is by using the .fail()
method. This method is specifically designed to catch errors that occur during the AJAX request. Here’s how you can use it:
$.ajax({
url: 'https://api.example.com/data',
method: 'GET'
})
.done(function(data) {
console.log('Success:', data);
})
.fail(function(jqXHR, textStatus, errorThrown) {
console.error('Request failed:', textStatus, errorThrown);
});
In this example, we initiate an AJAX GET request to a specified URL. If the request is successful, the .done()
method is executed, logging the returned data. However, if the request fails, the .fail()
method is triggered. It captures three parameters: jqXHR
, which contains the response data, textStatus
, which describes the type of error, and errorThrown
, which provides the error message.
Output:
Request failed: errorType errorMessage
Using the .fail()
method allows you to handle errors efficiently and provides feedback to the user. You can customize the error message or even redirect users based on the type of error encountered.
Implementing Error Handling with .always()
Another effective way to manage AJAX requests is through the .always()
method. This method runs after both the .done()
and .fail()
methods, regardless of the request’s outcome. It’s useful for executing code that should run after the request, like hiding loading indicators or resetting form fields.
$.ajax({
url: 'https://api.example.com/data',
method: 'GET'
})
.done(function(data) {
console.log('Data received:', data);
})
.fail(function(jqXHR, textStatus, errorThrown) {
console.error('Error:', textStatus, errorThrown);
})
.always(function() {
console.log('Request completed.');
});
In this example, the .always()
method is added to the AJAX call. No matter if the request succeeds or fails, the message “Request completed.” will be logged. This is particularly useful for cleanup tasks, ensuring that your application remains in a consistent state after the request.
Output:
Request completed.
By using the .always()
method, you can ensure that certain actions are taken regardless of the AJAX request’s success or failure. This enhances the reliability of your application and provides a smoother user experience.
Customizing Error Responses
Sometimes, you may want to provide users with more informative error messages based on the type of failure encountered. You can achieve this by examining the jqXHR
object returned in the .fail()
method. Here’s how:
$.ajax({
url: 'https://api.example.com/data',
method: 'GET'
})
.fail(function(jqXHR) {
let errorMessage;
switch (jqXHR.status) {
case 404:
errorMessage = 'Requested resource not found.';
break;
case 500:
errorMessage = 'Internal server error. Please try again later.';
break;
default:
errorMessage = 'An unexpected error occurred.';
}
console.error('Error:', errorMessage);
});
In this example, we check the status
property of the jqXHR
object to determine the type of error. Depending on the status code, we set a custom error message that provides more context to the user. This approach enhances user experience by guiding them on what to do next.
Output:
Error: Requested resource not found.
Customizing error responses not only informs users about the issue but can also help them troubleshoot problems effectively. By providing specific messages, you can reduce frustration and enhance user satisfaction.
Conclusion
Handling AJAX failures in jQuery is vital for creating robust web applications. By using methods like .fail()
, .always()
, and customizing error responses, you can effectively manage errors and maintain a smooth user experience. Remember to test your error handling thoroughly to ensure that your application can gracefully handle various failure scenarios. With these strategies in your toolkit, you’ll be well-prepared to tackle AJAX failures head-on.
FAQ
-
What is AJAX?
AJAX stands for Asynchronous JavaScript and XML, and it allows web applications to send and receive data asynchronously without refreshing the entire page. -
How can I check if an AJAX request failed?
You can use the.fail()
method in jQuery to capture errors and check the response status. -
What is the difference between .done() and .fail() in jQuery AJAX?
The.done()
method is executed when the AJAX request is successful, while the.fail()
method is executed when the request fails. -
Can I provide custom error messages in AJAX requests?
Yes, you can customize error messages based on the status code returned in the.fail()
method. -
What should I do if an AJAX request fails?
You should handle the failure gracefully by informing the user and possibly providing options to retry the request.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn