How to Submit Form With AJAX in jQuery
- Submit Form With AJAX in jQuery: Direct Approach
- Use jQuery Form Plugin to Submit Form With AJAX in jQuery
-
Submit Form With AJAX in jQuery: The
FormData
Approach
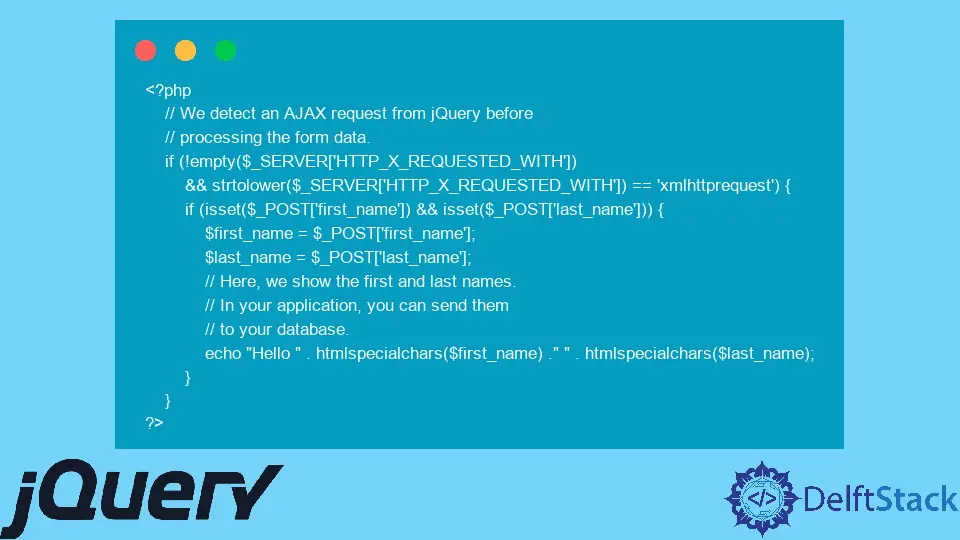
This article teaches you three methods that will submit a form using AJAX-in-jQuery. The first is the direct use of AJAX-in-jQuery, while the second employs the AJAX Form Plugin
.
In the third method, we use the FormData
object to grab the form data before sending it using AJAX.
Submit Form With AJAX in jQuery: Direct Approach
We call this a “direct approach” because there is no external library or anything special. First, you set up your HTML form and ensure you have the correct name attributes.
Then use jQuery to do the following.
- Use jQuery to listen for the form submission.
- Prevent the default submit behavior.
- Use the
ajax()
method in jQuery to start an AJAX connection. - Ensure the
type
property inajax()
isPOST
. - Ensure the value of the
url
property is the value of the HTMLaction
attribute. - Ensure the value of the
data
property is a serialized format of all form inputs. - Process the return data using a function as the value of the
success
property. - Process any error using the function as the value of the
error
property.
You’ll find the code implementation of these steps in the following code. Take note that the value of the HTML form attribute is a PHP script that “processes” the form.
This time, we only show the submitted data in the console. But, you can submit the form data to a database; you’ll find the PHP script at the end of this article.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery AJAX Submit form</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<style>
body { display: grid; justify-content: center; align-items: center; height: 100vh; }
form { border: 3px solid #1560bd; font-size: 1.2em; padding: 1.2em; }
</style>
</head>
<body>
<main>
<form id="html_form" action="process-jquery-form.php" method="POST">
<label for="first_name">First name</label>
<input id="first_name" type="text" name="first_name" required>
<label for="last_name">Last name</label>
<input id="last_name" type="text" name="last_name" required>
<input type="submit" name="submit" value="Submit form">
</form>
</main>
<script>
$("#html_form").submit(function(event) {
// Prevent the default submit behavior
event.preventDefault();
// "this" refers to the current HTML form
let current_html_form = $(this);
let action_url = current_html_form.attr('action');
$.ajax({
type: "POST",
url: action_url,
data: current_html_form.serialize(), // Turn the form elements into a usable string
success: function (data) {
console.log(data);
},
error: function (data) {
alert("An error occurred during form submission");
},
});
});
</script>
</body>
</html>
Output:
Use jQuery Form Plugin to Submit Form With AJAX in jQuery
This method results in submitting the form using AJAX in jQuery but differently. We’ll use the ajaxForm
method in the jQuery Form Plugin this time.
It has the same approach to submitting the form using AJAX with the following exception.
- Import the jQuery Form Plugin into your script.
- Use the
ajaxForm
method of the plugin. Behind the scenes, this takes care of the form submission. - The value of the
url
property is the script’s name that’ll process the submitted data. - You don’t write
$.ajax()
, instead you write$(form_selector).ajaxForm()
. Whereform_selector
is your HTML form’s class or ID name.
We’ve implemented these steps in the following code. You can check the output in the next image.
As a reminder, you’ll find the PHP script at the end of the article.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-AJAX-with-AJAX-Form.html</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery.form/4.3.0/jquery.form.min.js" integrity="sha512-YUkaLm+KJ5lQXDBdqBqk7EVhJAdxRnVdT2vtCzwPHSweCzyMgYV/tgGF4/dCyqtCC2eCphz0lRQgatGVdfR0ww==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
body { display: grid; justify-content: center; align-items: center; height: 100vh; }
form { border: 3px dotted #0a9; font-size: 1.2em; padding: 1.2em; }
</style>
</head>
<body>
<main>
<form id="html_form" action="process-jquery-form.php" method="POST">
<label for="first_name">First name</label>
<input id="first_name" type="text" name="first_name" required>
<label for="last_name">Last name</label>
<input id="last_name" type="text" name="last_name" required>
<input type="submit" name="submit" value="Submit form">
</form>
</main>
<script>
$(function(){
$('#html_form').ajaxForm({
type: "POST",
url:'process-jquery-form.php',
success: function (data) {
console.log(data);
},
error: function (data) {
alert("An error occurred during form submission");
},
})
});
</script>
</body>
</html>
Output:
Submit Form With AJAX in jQuery: The FormData
Approach
This method is the same as the direct approach because no external library is involved. But this time, we grab the form data using the FormData
object.
The remaining steps in the ajax()
method remain the same with the following additions.
- Add the
processData
property and set its value tofalse
. - Add the
contentType
property and set its value tofalse
. - The function in the
error
property should havejQXHR
,textStatus
, anderrorMessage
as arguments. Then you show an error message using theerrorMessage
argument.
The following code shows you how to do this. You’ll find the PHP script in the next section.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>03-jQuery-AJAX-with-FormData.html</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<style>
body { display: grid; justify-content: center; align-items: center; height: 100vh;}
form { border: 3px dashed #1975c7; font-size: 1.2em; padding: 1.2em; }
</style>
</head>
<body>
<main>
<form id="html_form" action="process-jquery-form.php" method="POST">
<label for="first_name">First name</label>
<input id="first_name" type="text" name="first_name" required>
<label for="last_name">Last name</label>
<input id="last_name" type="text" name="last_name" required>
<input type="submit" name="submit" value="Submit form">
</form>
</main>
<script>
$("#html_form").submit(function(event) {
// Prevent the default submit behavior
event.preventDefault();
let current_html_form = $(this);
let action_url = current_html_form.attr('action');
// Grab the form data using the FormData Object
// Later, we'll use the data in the ajax request.
let form_data = new FormData(document.getElementById('html_form'));
$.ajax({
type: "POST",
url: action_url,
data: form_data,
processData: false,
contentType: false,
success: function (data) {
console.log(data);
},
error: function (jQXHR, textStatus, errorMessage) {
console.log(errorMessage);
},
});
});
</script>
</body>
</html>
Output:
PHP Script for the HTML Form
The following is the PHP script used to process the HTML forms in this example. You can save it in your working directory as process-jquery-form.php
.
<?php
// We detect an AJAX request from jQuery before
// processing the form data.
if (!empty($_SERVER['HTTP_X_REQUESTED_WITH'])
&& strtolower($_SERVER['HTTP_X_REQUESTED_WITH']) == 'xmlhttprequest') {
if (isset($_POST['first_name']) && isset($_POST['last_name'])) {
$first_name = $_POST['first_name'];
$last_name = $_POST['last_name'];
// Here, we show the first and last names.
// In your application, you can send them
// to your database.
echo "Hello " . htmlspecialchars($first_name) ." " . htmlspecialchars($last_name);
}
}
?>
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn