jQuery AJAX Data
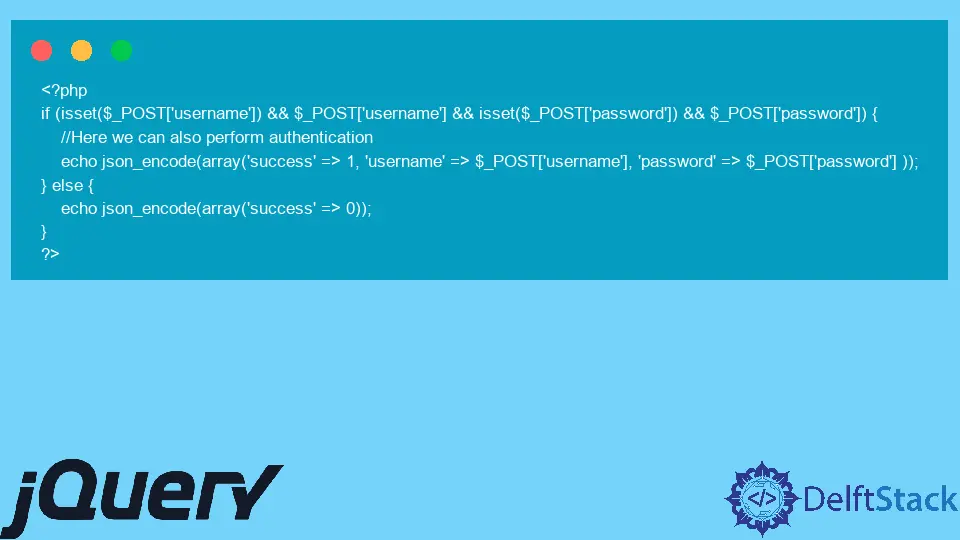
The AJAX data is the data sent to the server and used by the server.
This tutorial demonstrates how to send data to the server using AJAX in jQuery.
jQuery AJAX Data
The data sent to the server using AJAX can be a JSON object, string, or array. This data is further used on the server page for any purpose.
We can send single or multiple data using an array or JSON object. We use the syntax below to send multiple data fields with the array.
data: {username: username, password: password}
This method can send multiple data fields to the server, and we can use them for further processes. In this case, a JSON object is more convenient where we can send all the data fields in a serialized JSON object.
Let’s try an example to send multiple data fields using a JSON object. See example:
index.html
:
<!doctype html>
<html>
<head>
<title>jQuery Ajax Data</title>
<script src="https://code.jquery.com/jquery-3.3.1.js" integrity="sha256-2Kok7MbOyxpgUVvAk/HJ2jigOSYS2auK4Pfzbm7uH60=" crossorigin="anonymous"></script>
</head>
<body>
<form id="Login_Form" method="post">
<div>
Enter the Username:
<input type="text" name="username" id="User_Name" />
Enter the Password:
<input type="password" name="password" id="Password" />
<input type="submit" name="loginBtn" id="Login_Button" value="Login" />
</div>
</form>
<script type="text/javascript">
$(document).ready(function() {
$('#Login_Form').submit(function(e) {
e.preventDefault();
$.ajax({
type: "POST",
url: 'demo.php',
data: $(this).serialize(),
success: function(response) {
var JSON_Data = JSON.parse(response);
// user login check
if (JSON_Data.success == "1" && JSON_Data.username == "admin" && JSON_Data.password == "password" )
{
document.write('Login Success');
alert(response);
}
else
{
alert('Invalid User Name and Password!');
}
}
});
});
});
</script>
</body>
</html>
The code above sends the form data using the $.ajax
and post
method to the server, and the server will use the data for login purposes; the data is sent in a serialized object using data: $(this).serialize(),
syntax. Here is the demo.php
server page.
<?php
if (isset($_POST['username']) && $_POST['username'] && isset($_POST['password']) && $_POST['password']) {
//Here we can also perform authentication
echo json_encode(array('success' => 1, 'username' => $_POST['username'], 'password' => $_POST['password'] ));
} else {
echo json_encode(array('success' => 0));
}
?>
The server code checks if the user name and password are set, then creates a JSON object to send back to AJAX. We authenticated the login in jQuery on the index.html
page.
See the output for the code in the animation below.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook