jQuery AJAX 数据
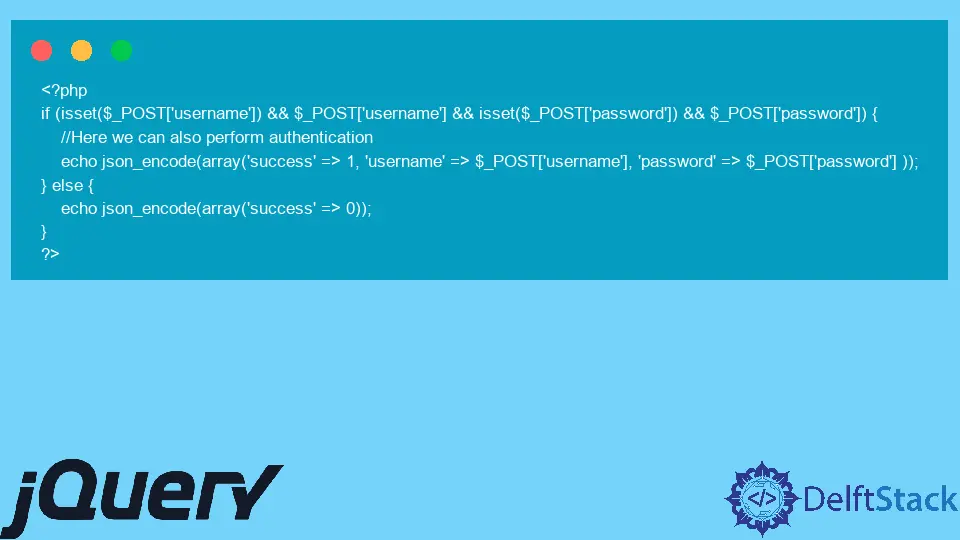
AJAX 数据是发送到服务器并由服务器使用的数据。本教程演示如何在 jQuery 中使用 AJAX 将数据发送到服务器。
jQuery AJAX 数据
使用 AJAX 发送到服务器的数据可以是 JSON 对象、字符串或数组。此数据会在服务器页面上进一步用于任何目的。
我们可以使用数组或 JSON 对象发送单个或多个数据。我们使用下面的语法将多个数据字段与数组一起发送。
data: {username: username, password: password}
该方法可以向服务器发送多个数据字段,我们可以将它们用于进一步的处理。在这种情况下,JSON 对象更方便,我们可以在序列化的 JSON 对象中发送所有数据字段。
让我们尝试一个使用 JSON 对象发送多个数据字段的示例。参见示例:
index.html
:
<!doctype html>
<html>
<head>
<title>jQuery Ajax Data</title>
<script src="https://code.jquery.com/jquery-3.3.1.js" integrity="sha256-2Kok7MbOyxpgUVvAk/HJ2jigOSYS2auK4Pfzbm7uH60=" crossorigin="anonymous"></script>
</head>
<body>
<form id="Login_Form" method="post">
<div>
Enter the Username:
<input type="text" name="username" id="User_Name" />
Enter the Password:
<input type="password" name="password" id="Password" />
<input type="submit" name="loginBtn" id="Login_Button" value="Login" />
</div>
</form>
<script type="text/javascript">
$(document).ready(function() {
$('#Login_Form').submit(function(e) {
e.preventDefault();
$.ajax({
type: "POST",
url: 'demo.php',
data: $(this).serialize(),
success: function(response) {
var JSON_Data = JSON.parse(response);
// user login check
if (JSON_Data.success == "1" && JSON_Data.username == "admin" && JSON_Data.password == "password" )
{
document.write('Login Success');
alert(response);
}
else
{
alert('Invalid User Name and Password!');
}
}
});
});
});
</script>
</body>
</html>
上面的代码使用 $.ajax
和 post
方法将表单数据发送到服务器,服务器将使用这些数据进行登录;使用 data: $(this).serialize(),
语法在序列化对象中发送数据。这是 demo.php
服务器页面。
<?php
if (isset($_POST['username']) && $_POST['username'] && isset($_POST['password']) && $_POST['password']) {
//Here we can also perform authentication
echo json_encode(array('success' => 1, 'username' => $_POST['username'], 'password' => $_POST['password'] ));
} else {
echo json_encode(array('success' => 0));
}
?>
服务器代码检查是否设置了用户名和密码,然后创建一个 JSON 对象以发送回 AJAX。我们在 index.html
页面上验证了 jQuery 中的登录。
请参阅下面动画中代码的输出。
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook