Pasar encabezados de solicitud en jQuery AJAX
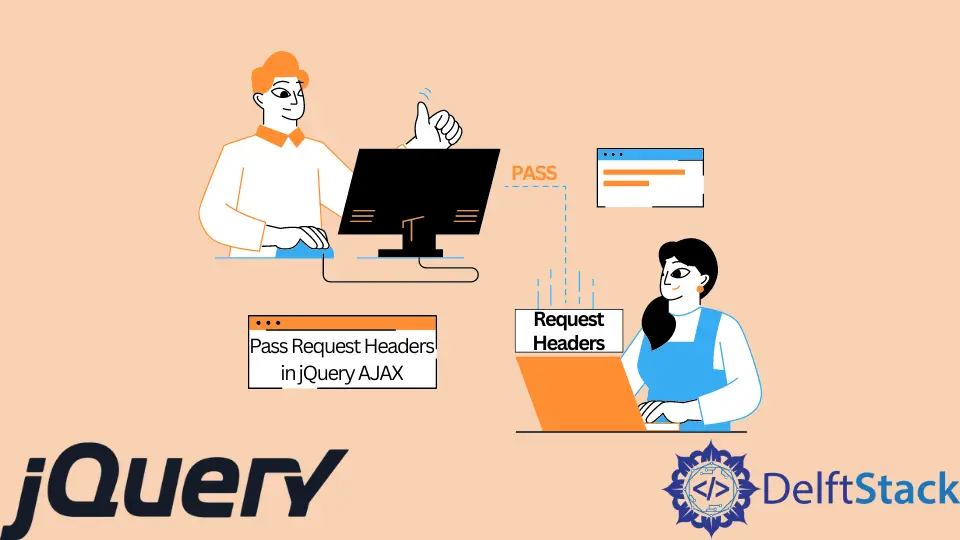
Los encabezados especifican el tipo de respuesta aceptada del servidor en jQuery AJAX. Este tutorial demuestra cómo usar los encabezados en jQuery AJAX.
Pasar encabezados de solicitud en jQuery AJAX
Como se mencionó anteriormente, los encabezados pueden especificar el tipo de respuesta aceptada del servidor en jQuery AJAX. Un encabezado es una opción integrada que se pasa al método ajax()
en jQuery.
Los encabezados son pares clave-valor enviados en la solicitud AJAX utilizando el objeto XMLHttpRequest
. Una solicitud HTTP asíncrona y un encabezado informarán al servidor qué tipo de respuesta aceptar.
También se puede utilizar una función de devolución de llamada para establecer las propiedades del encabezado.
La sintaxis para el encabezado jQuery AJAX es:
$.ajax({ headers : { key : value }})
La sintaxis de la función de devolución de llamada beforeSend
para sobrescribir las propiedades del encabezado es:
$.ajax({beforeSend: function (jqXHR, settings) { jqXHR.setRequestHeader(key, value) );}})
Donde headers : { key : value }
es una opción opcional. Especificará el tipo de respuesta aceptada del servidor al enviar la solicitud al servidor.
El valor predeterminado para los encabezados es {}
, que es un tipo de objeto simple. Las claves para los encabezados son Accept
, Accept-Encoding
, Accept-Language
, Connection
, Cookie
, User-Agent
, Host
, y Order-Number
, etc.
Y beforeSend
es una función opcional que establecerá o sobrescribirá para especificar el tipo de respuesta aceptada del servidor. Aceptará jqXHR
, y los parámetros settings
modificarán el objeto jqXHR
y agregarán un encabezado personalizado utilizando el método setRequestHeader
.
Probemos un ejemplo simple con encabezados AJAX para obtener los datos del servidor:
<!doctype html>
<html lang = "en">
<head>
<meta charset = "utf-8">
<script type = "text/javascript" src = "https://ajax.googleapis.com/ajax/libs/jquery/1.11.2/jquery.min.js"></script>
<title> jQuery Ajax headers </title>
</head>
<body>
<h3> jQuery ajax headers : </h3>
<button id = "Send_Request" > Send the ajax request with headers <button/>
<br>
<p id = "para1" style = "color : green"> </p>
<script type = "text/javascript">
$(document).ready( function () {
$('#Send_Request').click( function(){
var Ajax_Request = $.ajax( { url : 'http://localhost/',
contentType : 'application/json',
dataType : 'json',
headers: {"Accepts": "text/plain; charset=utf-8"}
});
ajxReq.success( function ( Request_Data, Request_Status, jqXHR ) {
$( '#para1' ).append( '<h2> The JSON data: </h2>');
$( '#para1' ).append( '<p> The Request Date : ' + Request_Data.date + '</p>');
$( '#para1' ).append ('<p> The Request Time: ' + Request_Data.time + '</p>');
$( '#para1' ).append( '<p> The Request Status : ' + Request_Status + '</p>');
});
Ajax_Request.error( function ( jqXHR, Text_Status, Error_Message ) {
$( "p" ).append( "The status is :" +Text_Status);
});
});
});
</script>
</body>
</html>
El código anterior intentará enviar una solicitud AJAX con opciones de encabezado "Accepts": "text/plain; charset=utf-8"
. Ver la salida:
Como se muestra en la salida, arroja un error porque, en el encabezado, solicitamos texto sin formato del servidor, pero los datos recibidos son JSON, por lo que arroja un error. Para procesar con éxito la solicitud, debemos establecer el valor del encabezado como JSON.
Intentemos corregir el ejemplo:
<!doctype html>
<html lang = "en">
<head>
<meta charset = "utf-8">
<script type = "text/javascript" src = "https://ajax.googleapis.com/ajax/libs/jquery/1.11.2/jquery.min.js"></script>
<title> jQuery Ajax headers </title>
</head>
<body>
<h3> jQuery ajax headers : </h3>
<button id = "Send_Request" > Send the ajax request with headers <button/>
<br>
<p id = "para1" style = "color : green"> </p>
<script type = "text/javascript">
$(document).ready( function () {
$('#Send_Request').click( function(){
// url from where we want to get the data
var ajxReq = $.ajax( { url : 'http://time.jsontest.com',
contentType : 'application/json',
dataType : 'json',
headers: {"Accept": "application/json"}
});
ajxReq.success( function ( Request_Data, Request_Status, jqXHR ) {
$( '#para1' ).append( '<h2> The JSON data: </h2>');
$( '#para1' ).append( '<p> The Request Date : ' + Request_Data.date + '</p>');
$( '#para1' ).append ('<p> The Request Time: ' + Request_Data.time + '</p>');
$( '#para1' ).append( '<p> The Request Status : ' + Request_Status + '</p>');
});
ajxReq.error( function ( jqXHR, Text_Status, Error_Message ) {
$( "p" ).append( "The status is : " + Text_Status);
});
});
});
</script>
</body>
</html>
El código anterior enviará una solicitud AJAX con encabezados con valor JSON, y el código recuperará los datos JSON del servidor. Ver salida:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook