How to Convert SVG to PNG With JavaScript
-
Use
Canvg
to Convert SVG String to Image in JavaScript -
Use
Canvg
to Convert SVG File to Image in JavaScript
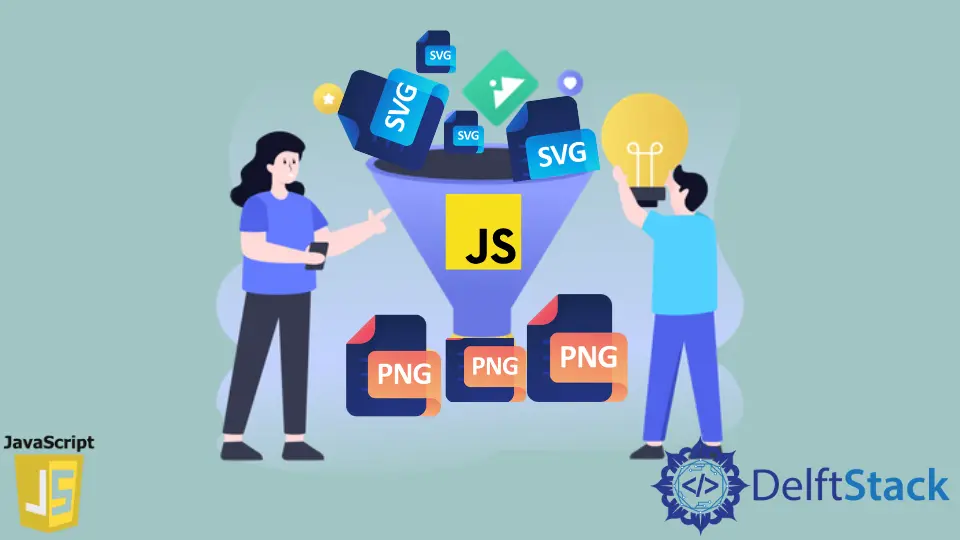
This tutorial teaches how to convert an SVG to a PNG image in your web browser. The approach we’ll use involves using Canvg
and saving the content of the Canvas as a PNG image with the toDataURL
method.
Use Canvg
to Convert SVG String to Image in JavaScript
The Canvg
is an open-source JavaScript parser and renderer that will convert an SVG to an image in your web browser. The SVG can be in a string format or from a DOM element.
Here, we’ll show you how to convert an SVG string to an image.
To get started with Canvg
, install a local server like XAMPP because Canvg
in the browser uses ES Modules. Afterward, visit the official Canvg
GitHub repo and select your preferred download option.
Meanwhile, we’ll go for the option that allows you to use Canvg
in your web browser.
The following are the steps to convert an SVG string to PNG in a web browser with Canvg
.
-
Create a folder in your server for this project.
-
Import
Canvg
fromSkypack CDN
. -
Create a
Canvg
initialization variable and set its value toNULL
. -
Create an
onload
function. -
Within the
onload
function, do the following:- Select the Canvas from your HTML.
- Get the 2d context from the Canvas.
- Set the new value of the
Canvg
initialization variable toCanvg
’sfromString
method. - Read the SVG code into the
fromString
method. - Call on the
start
method available in the initialization variable. - Use the
toDataURL
to convert the image to PNG. - Write the image on the screen.
This code is the implementation of the steps detailed above.
<body>
<canvas id="myCanvas"></canvas>
<script type="module">
// Import Canvg from the Skypack CDN
import { Canvg } from 'https://cdn.skypack.dev/canvg';
// Set an initialization variable to null
let v = null;
// Create a function that fires when the
// web browser loads the file
window.onload = () => {
const canvas = document.querySelector('canvas');
const ctx = canvas.getContext('2d');
// Read the SVG string using the fromString method
// of Canvg
v = Canvg.fromString(ctx, '<svg height="200" width="300"><polygon points="100,10 40,198 190,78 10,78 160,198" style="fill:lime;stroke:navy;stroke-width:5;fill-rule:nonzero;"/></svg>');
// Start drawing the SVG on the canvas
v.start();
// Convert the Canvas to an image
var img = canvas.toDataURL("img/png");
// Write the image on the screen
document.write('<img src="' + img + '"/>');
}
</script>
</body>
Output:
You can confirm it’s an image if you right-click on it and select open image in a new tab. You’ll get the following.
Use Canvg
to Convert SVG File to Image in JavaScript
You’ll find this approach useful if you have your SVG saved as a file. Canvg
allows you to read the SVG file asynchronously, and you can write the SVG to screen as an image.
The following are the steps to display an SVG file in PNG format using Canvg
.
-
Create a folder in your server for this project.
-
Import
Canvg
fromSkypack CDN
. -
Create a
Canvg
initialization variable and set its value toNULL
. -
Create an asynchronous
onload
function. -
Within the
onload
function, do the following:- Select the Canvas from your HTML.
- Get the 2d context from the Canvas.
- Set the new value of the
Canvg
initialization variable to theawait
operator andCanvg
’sfrom
method. - Read the SVG file into the
from
method. - Call on the
start
method available in the initialization variable. - Use the
toDataURL
to convert the image to PNG. - Write the image on the screen.
This code is the implementation of converting an SVG file to PNG.
<body>
<canvas id="myCanvas"></canvas>
<script type="module">
// Import Canvg from the Skypack CDN
import { Canvg } from 'https://cdn.skypack.dev/canvg';
// Set an initialization variable to null
let v = null;
// Create a function that fires when the
// web browser loads the file
window.onload = async () => {
const canvas = document.querySelector('canvas');
const ctx = canvas.getContext('2d');
// Read the SVG file using the from method
// of Canvg
v = await Canvg.from(ctx, './cat.svg');
// Start drawing the SVG on the canvas
v.start();
// Convert the Canvas to an image
var img = canvas.toDataURL("img/png");
// Write the image on the screen
document.write('<img src="' + img + '"/>');
}
</script>
</body>
Output:
Also, you can confirm it’s a PNG image.
In the second example, we used a cat SVG. You can download the cat SVG on Freesvg.
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn