How to Change Image on Hover in JavaScript
-
Use HTML Attributes
onmouseover
andonmouseout
to Change Image on Hover -
Use
event listeners
to Change Image on Hover - Conclusion
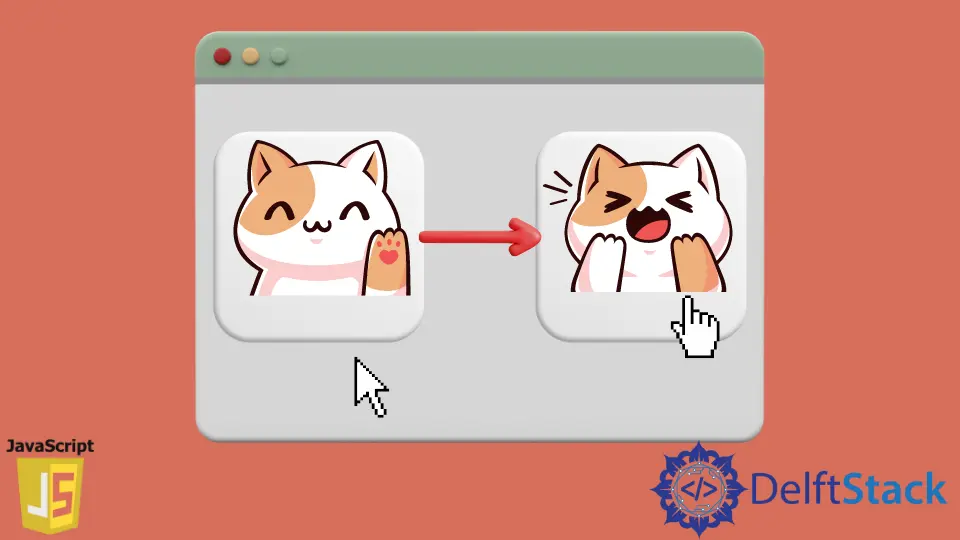
Switching an image on hover brings a stylish touch to a UI. Achieved through simple JavaScript functions, it enhances visual appeal seamlessly.
In web development, creating an engaging and dynamic user interface is crucial for providing an immersive experience. One effective way to achieve this is by incorporating interactive elements, such as image swapping on hover.
There are techniques that allow developers to seamlessly transition between images when a user hovers over a specified element.
Use HTML Attributes onmouseover
and onmouseout
to Change Image on Hover
Changing images on hover enhances the user experience. By employing onmouseover
and onmouseout
events in HTML and JavaScript, a dynamic effect is achieved.
When a user hovers over an image, the onmouseover
event triggers the replacement of the current image with another, providing a visual response. Conversely, the onmouseout
event restores the original image when the user moves the cursor away.
This straightforward technique adds interactivity to web content, improving engagement.
You can use the onmouseover
and onmouseout
attributes to change the src
attribute of an image when the mouse is over it and revert it when the mouse leaves.
Code Snippet:
<img src="original-image.jpg" onmouseover="changeImage('hover-image.jpg')" onmouseout="changeImage('original-image.jpg')" />
<script>
function changeImage(newSrc) {
document.querySelector('img').src = newSrc;
}
</script>
The HTML structure consists of an img
element initially set with a source attribute (src
) pointing to original-image.jpg
.
Two additional attributes, onmouseover
and onmouseout
, are included to trigger JavaScript functions when the mouse hovers over or leaves the image. The onmouseover
attribute calls the changeImage('hover-image.jpg')
function when the mouse is over the image, and the onmouseout
attribute calls changeImage('original-image.jpg')
when the mouse leaves the image.
In the JavaScript part, the changeImage
function is declared, taking a parameter (newSrc
) as the new image source. Within the function, document.querySelector('img').src
is employed to select the img
element and update its source attribute with the provided newSrc
.
Output:
This presented that onmouseover
and onmouseout
with JavaScript can present a simple yet effective method that can be adapted and expanded upon to create more intricate interactive elements in web development.
Use event listeners
to Change Image on Hover
Elevate user interaction by changing images on hover with event listeners. Using JavaScript, mouseover
triggers image swap, instantly providing a visual response when the cursor hovers.
Event listeners
efficiently handle this dynamic behavior, ensuring seamless transitions between images. The result is an engaging and responsive user experience on websites or applications.
You can also use JavaScript event listeners
to have a better separation of HTML and JavaScript.
Code Snippet:
<img id="myImage" src="original-image.jpg" />
<script>
var image = document.getElementById('myImage');
image.addEventListener('mouseover', function() {
image.src = 'hover-image.jpg';
});
image.addEventListener('mouseout', function() {
image.src = 'original-image.jpg';
});
</script>
JavaScript event listeners
are employed in the following manner: First, an image
variable is declared using document.getElementById('myImage')
to reference the image element.
Subsequently, a mouseover
event listener is set up to respond when the mouse hovers over the image. The function associated with this listener changes the image source to hover-image.jpg
.
Additionally, a mouseout
event listener is configured to trigger when the mouse leaves the image, with the corresponding function reverting the image source to original-image.jpg
.
Output:
Showing that mouseover
and mouseout
event listeners could present a straightforward application, showcasing how an image can transform dynamically based on user interactions.
Conclusion
The methods of using onmouseover
and onmouseout
, and mouseover
and mouseout
events in vanilla JavaScript all provide effective means to dynamically change images on hover.
By combining HTML attributes onmouseover
and onmouseout
with JavaScript, developers can effortlessly implement image swapping on hover. This technique provides a visually appealing way to engage users and enhance the overall user experience on a website.
By incorporating mouseover
and mouseout
event listeners, developers can seamlessly respond to user actions and create dynamic, interactive elements on web pages.