How to Fade-In Image Using JavaScript
-
Use the
className
Property to Fade-In Image -
Use the
requestAnimationFrame()
Method to Fade-In Image - Conclusion
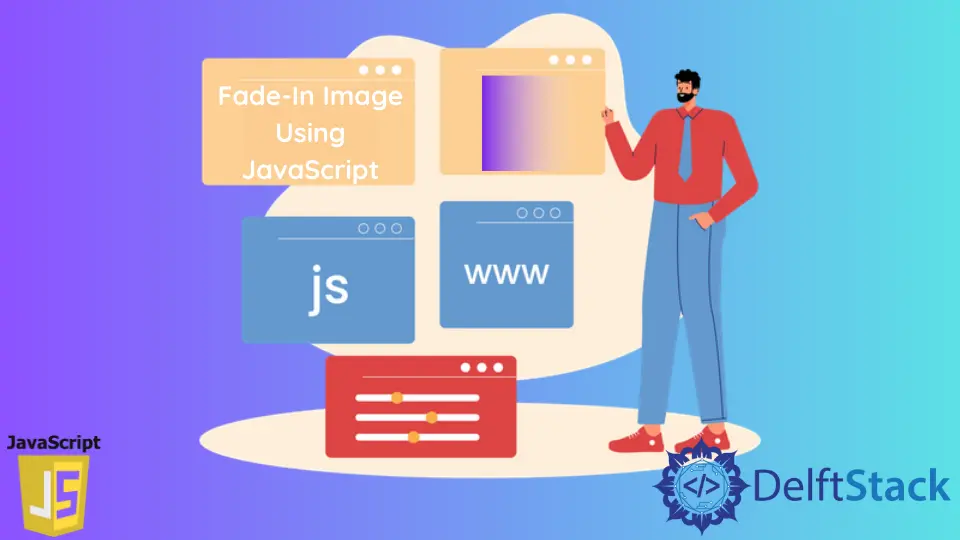
When it comes to creating a smooth fade-in effect for images on a web page, JavaScript provides valuable tools and methods that enhance the user experience. While it’s possible to achieve basic image fading using CSS alone, JavaScript introduces dynamic control and interactivity that CSS alone can’t match.
In this article, we explore two distinct methods for achieving image fade-in effects: the className
property and the requestAnimationFrame()
method. We’ll look into the implementation of each method, demonstrating how they can be used to make your images gracefully appear on the web page, adding an engaging touch to your web design.
Use the className
Property to Fade-In Image
Using the className
property to fade-in an image involves adding or changing a CSS class associated with the image element. This CSS class should contain styles that gradually increase the image’s opacity, creating a smooth fade-in effect.
We will generate an img
tag with a viable src
. A clickable event is present to initiate the fade-in of the image.
The main work here is with the className
property that will refer to a certain CSS block and align that with the img id
instance.
Code - HTML File:
<img id="img" style="width: 200px" src="https://images.unsplash.com/photo-1653450283266-c788c2ca4ab2?ixlib=rb-1.2.1&raw_url=true&q=80&fm=jpg&crop=entropy&cs=tinysrgb&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=872" alt="Image 1">
<br><br>
<button type="button" onclick="myFunction()">Change</button>
In this HTML code, we have an image element with the id attribute set to "img"
and an inline style that specifies the width of the image (200px
). The src
attribute of the image specifies the source URL for the image, and the alt
attribute provides alternative text for the image.
Then, the two line breaks (<br><br>
) create some space between the image and the button. Following the image, there is a button element, and the button is set to be of type "button"
, which means it won’t submit a form if it’s placed inside a form element.
The onclick
attribute specifies a JavaScript function to be executed when the button is clicked. In this case, it calls a function named "myFunction"
.
The purpose of this HTML code is to display an image with the ability to change it when the "Change"
button is clicked.
Code - CSS File:
img {
opacity: 0;
filter: alpha(opacity=40);
}
.animation {
-webkit-transition: 1s;
-moz-transition: 1s;
-o-transition: 1s;
transition: 1s;
opacity: 1;
}
In this CSS code, we set the initial CSS properties for an image. We apply an opacity of 0
and a filter to adjust the opacity for older versions of Internet Explorer, and this essentially makes the image invisible when the page loads.
We then define a CSS class called "animation"
to create a smooth transition effect when changing the image’s opacity. We set transition properties for various web browsers, ensuring cross-browser compatibility with the transition
property.
Additionally, we set the opacity of the image within this class to 1
, which means that when this class is applied to the image, it will gradually become fully visible with a one-second transition duration. This way, when you add the "animation"
class to the image element in your HTML or through JavaScript, it will smoothly fade-in from its initial hidden state.
Code - JavaScript File:
function myFunction() {
document.getElementById('img').className = 'animation';
}
In this JavaScript code, we have a JavaScript function named "myFunction"
, which retrieves the HTML element with the ID 'img'
using document.getElementById('img')
. It then sets the className
property of that element to 'animation'
.
This means that when myFunction()
is executed, it adds the animation
CSS class to the HTML element with the ID img
. As a result, the element will inherit the properties defined in the animation
CSS class, causing a smooth transition of opacity from 0
to 1
, effectively making the element fade-in.
This JavaScript code provides an easy way to trigger the fade-in effect for the specified element by adding the animation
class to it.
Output:
Use the requestAnimationFrame()
Method to Fade-In Image
Using the requestAnimationFrame()
method to fade-in an image involves leveraging this built-in browser function to create a smooth and efficient animation effect.
In this regard, we will have a function fadeIn
that will take the instance of the img
tag. Later, the fadeIn
function will operate on the requestAnimationFrame()
and the setTimeout()
, and a time frame is located based on the current browser detail.
Code - HTML File:
<img id="what" src="https://images.unsplash.com/photo-1653372500616-ff0a2847f7ca?crop=entropy&cs=tinysrgb&fm=jpg&ixlib=rb-1.2.1&q=80&raw_url=true&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=774" draggable="true" ondragstart="drag(event)" width="200" height="150">
<br><br>
<button onclick="myFunction()">Change</button>
In this HTML code, we have an image element with the id
set to "what"
, and it displays an image fetched from a URL. The image is draggable due to the draggable
attribute, and when a drag operation starts, it triggers a JavaScript function called "drag(event)"
through the ondragstart
attribute, and the image is also given a width and height of 200x150
pixels.
Beneath the image, there is a button element. When this button is clicked, it calls a JavaScript function named "myFunction()"
using the onclick
attribute.
This code’s primary purpose is to display the image, enable dragging, and offer a way to trigger an action, which can be defined in the "myFunction()"
JavaScript function, which will be discussed in the following JavaScript code.
Code - CSS File:
img {
opacity: 0;
filter: alpha(opacity=40);
}
In this CSS code, we’re applying styles to all img
elements. We set the initial opacity of these images to 0
, making them completely invisible.
Additionally, we include a filter property using alpha(opacity=40)
for older versions of Internet Explorer. This filter property adjusts the opacity to 40
, ensuring a level of transparency.
The combined effect is that the images are initially hidden, and semi-transparent, and you can later use JavaScript or CSS transitions to control their visibility and create fade-in effects when needed.
Code - JavaScript File:
function fadeIn(el) {
el.style.opacity = 0;
var tick = function() {
el.style.opacity = +el.style.opacity + 0.05;
if (+el.style.opacity < 1) {
var x = (window.requestAnimationFrame && requestAnimationFrame(tick)) ||
setTimeout(tick, 16)
}
};
tick();
}
function myFunction() {
var el = document.getElementById('what');
console.log(el);
fadeIn(el);
}
In this JavaScript code, we have two functions. The first function, named "fadeIn(el)"
, is responsible for creating a smooth fade-in effect for an HTML element passed as an argument (referred to as "el"
).
Initially, it sets the element’s opacity to 0
. Then, it defines an inner function called tick
that incrementally increases the element’s opacity by 0.05
on each iteration.
This inner function is called recursively until the opacity reaches 1
. During the transition, it utilizes the requestAnimationFrame
method for efficient animation, falling back to setTimeout
if necessary.
The second function, "myFunction()
, is invoked when a specific action occurs, typically triggered by user interaction. This function retrieves the HTML element with the ID 'what'
using document.getElementById('what')
, and then it logs the element to the console for inspection.
Finally, it calls the fadeIn(el)
function and passes the element as an argument, initiating the fade-in effect. The code aims to create a smooth transition that gradually reveals the specified element with a fading effect when myFunction()
is called, providing an elegant and gradual visibility change.
Output:
Conclusion
In this article, we’ve explored two methods for achieving image fade-in effects: the className
property and the requestAnimationFrame()
method. Both methods provide powerful means to control the visibility and transitions of images on a web page.
Whether we prefer the simplicity of adding and changing CSS classes or the efficiency of the requestAnimationFrame()
method, the choice ultimately depends on our project’s requirements and our creativity as a web developer. By understanding and utilizing these techniques, you can bring life and interactivity to your web designs, leaving a lasting impression on your site’s visitors.