How to Swap Images in JavaScript
-
Swap an Image Using the
onclick
Event in JavaScript - Swap an Image on Button Click in JavaScript
- Swap an Image on Mouse Click in JavaScript
-
Swap Multiple Images Using the
onclick
Event in JavaScript
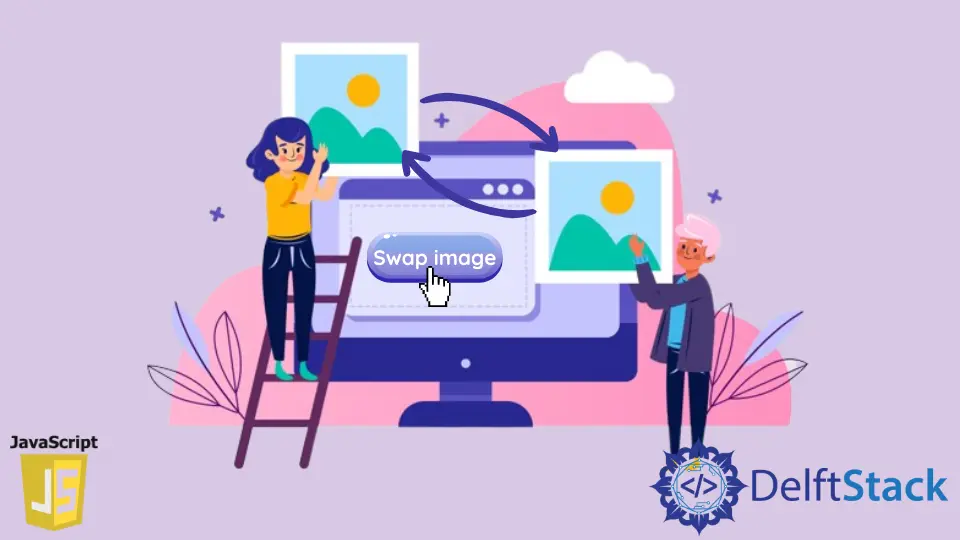
This article will discuss how to swap an image using the onclick
event and other methods in JavaScript.
Swap an Image Using the onclick
Event in JavaScript
Below is the complete code for swapping images using the onclick
event.
<!DOCTYPE html>
<html>
<head>
<title>swap image onclick javascript
</title>
<style type="text/css">
h2 {
text-align: center;
font-size: 50px;
}
img#getImage {
width: 300px;
height: auto;
border: 4px solid #a1a1a1;
}
div {
text-align: center;
}
</style>
</head>
<body>
<div>
<h2>Change image onClick event here...</h2>
<img src="image1.jpg" id="getImage">
</div>
<div>
<input type="button" onclick="imagefun()" value="Swap Image">
</div>
<script>
function imagefun() {
var Image_Id = document.getElementById('getImage');
if (Image_Id.src.match("image1.jpg")) {
Image_Id.src = "image2.jpg";
}
else {
Image_Id.src = "image1.jpg";
}
}
</script>
</body>
</html>
To make this code work for you, you’ll need to insert your image source link in Image_ID.src
. You can access the code using this link.
Firstly, create an HTML code structure, an image tag img
, and a button in the body section. After that, add the image source path and input an id to get the source code; the id name is getImage
, and the image source name is image1
.
Afterward, add a script in the below code and apply the function. Now, add the function in the button tag, which is imagefun
, with the help of the onclick()
event.
In the function, get image src
by id using the JavaScript document.getElementById()
function. Then, add an if...else
condition for the change image onclick
event, so two defined image source codes change with the onclick
event.
Here, parts of the code implement a swap of multiple images. First, the visible code of the image and input button is in the section below.
<div>
<h2>Swap image onClick event here...</h2>
<img src="image1.jpg" id="getImage">
</div>
<div>
<input type="button" onclick="imagefun()" value="Swap Image">
</div>
Displayed here is the JavaScript code.
<script>
function imagefun() {
var Image_Id = document.getElementById('getImage');
if (Image_Id.src.match("image1.jpg")) {
Image_Id.src = "image2.jpg";
}
else {
Image_Id.src = "image1.jpg";
}
}
</script>
Additionally, you can create some implementation for the design structure to better show the image and button at the front end.
<style type="text/css">
h2 {
text-align: center;
font-size: 30px;
}
img#getImage {
width: 300px;
height: auto;
border: 4px solid #a1a1a1;
}
div {
text-align: center;
}
</style>
Swap an Image on Button Click in JavaScript
Similarly, you can swap an image on a button click using JavaScript. An example is provided below.
<div>
<h2>Swap image onClick event here...</h2>
<img src="image1.jpg" id="getImage">
</div>
<div>
<button onclick="imagefun()">Image Swap</button>
</div>
Change only one line in the HTML code and replace the input tag with the button. This is the main code line.
The same codes as above (previous section) will be used. Define the script code, or add a script tag with a function.
Swap an Image on Mouse Click in JavaScript
Alternatively, you can swap an image on mouse click in JavaScript. Below is an example code.
<!DOCTYPE html>
<html>
<head>
<title>javascript change image onclick event
</title>
<style type="text/css">
h2 {
text-align: center;
font-size: 30px;
}
img#getImage {
width: 300px;
height: auto;
border: 4px solid #a1a1a1;
}
div {
text-align: center;
}
</style>
</head>
<body>
<div>
<h2>Swap image onClick event here...</h2>
<img src="image1.jpg" onmouseover="imagefun()" id="getImage">
</div>
<script>
function imagefun() {
var Image_Id = document.getElementById('getImage');
if (Image_Id.src.match("image1.jpg")) {
Image_Id.src = "image2.jpg";
}
else {
Image_Id.src = "image1.jpg";
}
}
</script>
</body>
</html>
Add a mouse hover event in your code. When you click and want to change the image into another one through a mouse, it will automatically change the image on the mouse click event.
Otherwise, add only this line and remove your editor’s old input and button code.
<img src="image1.jpg" onmouseover="imagefun()" id="getImage">
Swap Multiple Images Using the onclick
Event in JavaScript
Here, you can implement two images to swap. Therefore, more images can be added to swap multiple images using the onclick
events.
Now, check the code example below for changing multiple images using the onclick
event.
function imagefun() {
var Image_Id = document.getElementById('getImage');
if (Image_Id.src.match('image1.jpg')) {
Image_Id.src = 'image2.jpg';
Image_Id.src = 'image3.jpg';
} else {
Image_Id.src = 'image1.jpg';
}
}
As an example, an additional image source with script code is added. Afterward, multiple images can be displayed from these codes.
You can add as many pictures as you want to your page.