使用 JavaScript 将 SVG 转换为 PNG
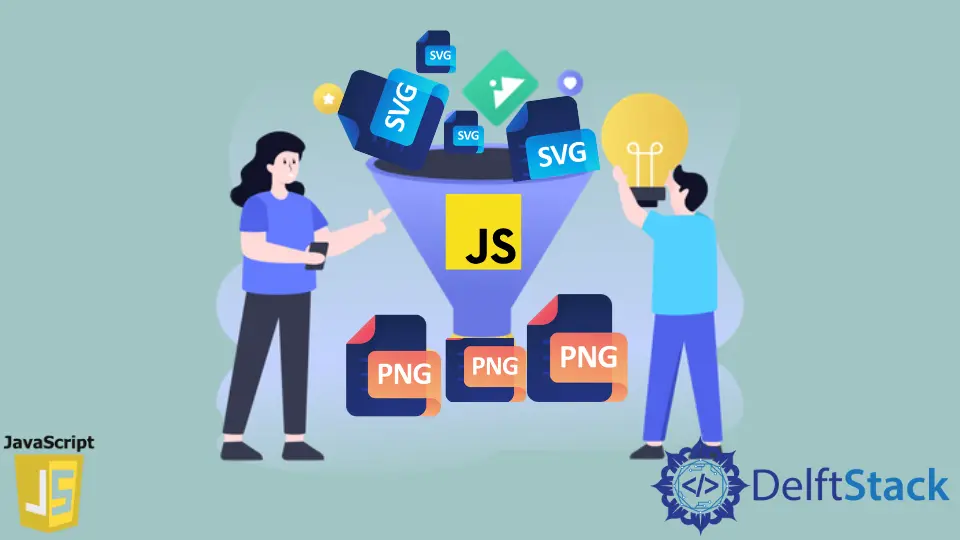
本教程教你如何在 Web 浏览器中将 SVG 转换为 PNG 图像。我们将使用的方法涉及使用 Canvg
并使用 toDataURL
方法将 Canvas 的内容保存为 PNG 图像。
在 JavaScript 中使用 Canvg
将 SVG 字符串转换为图像
Canvg
是一个开源的 JavaScript 解析器和渲染器,可以将 SVG 转换为 Web 浏览器中的图像。SVG 可以是字符串格式或来自 DOM 元素。
在这里,我们将向你展示如何将 SVG 字符串转换为图像。
要开始使用 Canvg
,安装像 XAMPP 这样的本地服务器 因为浏览器中的 Canvg
使用 ES 模块。然后,访问官方 Canvg
GitHub 存储库 并选择你喜欢的下载选项。
同时,我们将选择允许你在网络浏览器中使用 Canvg
的选项。
以下是在 Web 浏览器中使用 Canvg
将 SVG 字符串转换为 PNG 的步骤。
-
在你的服务器中为此项目创建一个文件夹。
-
从
Skypack CDN
导入Canvg
。 -
创建一个
Canvg
初始化变量并将其值设置为NULL
。 -
创建一个
onload
函数。 -
在
onload
函数中,执行以下操作:- 从你的 HTML 中选择 Canvas。
- 从 Canvas 中获取 2d context。
- 将
Canvg
初始化变量的新值设置为Canvg
的fromString
方法。 - 将 SVG 代码读入
fromString
方法。 - 调用初始化变量中可用的
start
方法。 - 使用
toDataURL
将图像转换为 PNG。 - 在屏幕上写入图像。
这段代码是上面详述的步骤的实现。
<body>
<canvas id="myCanvas"></canvas>
<script type="module">
// Import Canvg from the Skypack CDN
import { Canvg } from 'https://cdn.skypack.dev/canvg';
// Set an initialization variable to null
let v = null;
// Create a function that fires when the
// web browser loads the file
window.onload = () => {
const canvas = document.querySelector('canvas');
const ctx = canvas.getContext('2d');
// Read the SVG string using the fromString method
// of Canvg
v = Canvg.fromString(ctx, '<svg height="200" width="300"><polygon points="100,10 40,198 190,78 10,78 160,198" style="fill:lime;stroke:navy;stroke-width:5;fill-rule:nonzero;"/></svg>');
// Start drawing the SVG on the canvas
v.start();
// Convert the Canvas to an image
var img = canvas.toDataURL("img/png");
// Write the image on the screen
document.write('<img src="' + img + '"/>');
}
</script>
</body>
输出:
如果你右键单击它并选择在新标签页中打开图像,你可以确认它是一个图像。你会得到以下。
在 JavaScript 中使用 Canvg
将 SVG 文件转换为图像
如果你将 SVG 保存为文件,你会发现这种方法很有用。Canvg
允许你异步读取 SVG 文件,并且你可以将 SVG 作为图像写入屏幕。
以下是使用 Canvg
以 PNG 格式显示 SVG 文件的步骤。
-
在你的服务器中为此项目创建一个文件夹。
-
从
Skypack CDN
导入Canvg
。 -
创建一个
Canvg
初始化变量并将其值设置为NULL
。 -
创建一个异步
onload
函数。 -
在
onload
函数中,执行以下操作:- 从你的 HTML 中选择 Canvas。
- 从 Canvas 中获取 2d context。
- 将
Canvg
初始化变量的新值设置为await
运算符和Canvg
的from
方法。 - 将 SVG 文件读入
from
方法。 - 调用初始化变量中可用的
start
方法。 - 使用
toDataURL
将图像转换为 PNG。 - 在屏幕上写下图像。
此代码是将 SVG 文件转换为 PNG 的实现。
<body>
<canvas id="myCanvas"></canvas>
<script type="module">
// Import Canvg from the Skypack CDN
import { Canvg } from 'https://cdn.skypack.dev/canvg';
// Set an initialization variable to null
let v = null;
// Create a function that fires when the
// web browser loads the file
window.onload = async () => {
const canvas = document.querySelector('canvas');
const ctx = canvas.getContext('2d');
// Read the SVG file using the from method
// of Canvg
v = await Canvg.from(ctx, './cat.svg');
// Start drawing the SVG on the canvas
v.start();
// Convert the Canvas to an image
var img = canvas.toDataURL("img/png");
// Write the image on the screen
document.write('<img src="' + img + '"/>');
}
</script>
</body>
输出:
此外,你可以确认它是 PNG 图像。
在第二个示例中,我们使用了 cat SVG。你可以在 Freesvg 上下载 cat SVG。
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn