Single Quotes vs. Double Quotes in JavaScript
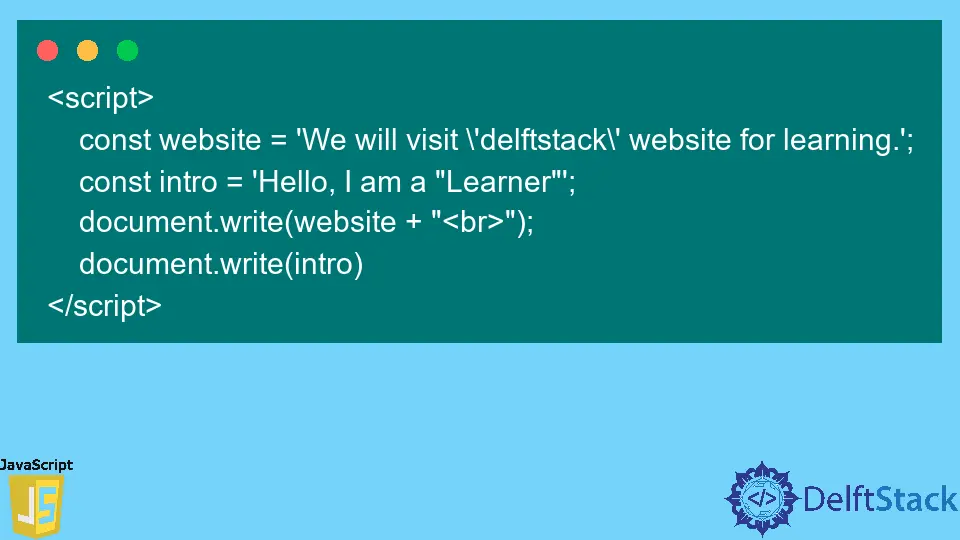
In the world of JavaScript, the choice between single quotes and double quotes can often spark debate among developers. While both options serve the same fundamental purpose of defining string literals, they come with their own sets of nuances.
In this article, we will explore the differences between single quotes and double quotes in JavaScript, how to use them effectively in your code, and the best practices to follow. Understanding these subtle distinctions can help you write cleaner, more efficient code, and enhance your overall coding experience. So, let’s dive in and clarify this common question in JavaScript programming!
The Basics of Quotes in JavaScript
In JavaScript, strings can be defined using either single quotes ('
) or double quotes ("
). Both are valid and interchangeable in most cases, but there are specific scenarios where one may be more advantageous than the other.
For instance, when you need to include a quote character within a string, using the opposite type can simplify your code. If you use double quotes to define your string, you can include single quotes without escaping them, and vice versa. This flexibility can reduce the number of escape characters in your code, making it cleaner and easier to read.
Here’s a simple example:
let singleQuoteString = 'This is a string with a single quote: \'';
let doubleQuoteString = "This is a string with a double quote: \"";
Output:
This is a string with a single quote: '
This is a string with a double quote: "
When deciding which to use, consistency is key. Many developers prefer to stick to one type throughout their code to maintain readability and avoid confusion. However, JavaScript does not enforce a strict rule on which to use, so it ultimately comes down to personal preference or team conventions.
Best Practices for Using Quotes
When writing JavaScript code, following best practices can help you maintain clarity and consistency. Here are some guidelines to consider when deciding between single and double quotes:
-
Consistency is Crucial: Choose one style and stick with it throughout your project. This makes your code easier to read and maintain.
-
Consider JSON: When working with JSON data, remember that JSON requires double quotes for keys and string values. This can influence your choice when dealing with JSON strings in your JavaScript code.
-
Use ESLint: If you’re working in a team or on a larger project, consider using a linter like ESLint to enforce a consistent quoting style. This can automatically flag inconsistencies and help maintain uniformity across your codebase.
-
Escape Characters: If your string contains the same type of quote that you are using to define it, you will need to escape it. For example, if you use single quotes for a string that contains a single quote, you must escape it with a backslash.
Here’s an example of escaping quotes:
let escapedSingleQuote = 'It\'s a sunny day!';
let escapedDoubleQuote = "He said, \"Hello!\"";
Output:
It's a sunny day!
He said, "Hello!"
By following these best practices, you can enhance the readability and maintainability of your JavaScript code, making it easier for yourself and others to understand.
Conclusion
In conclusion, the choice between single quotes and double quotes in JavaScript ultimately comes down to personal preference and coding standards. Both options are valid and can be used interchangeably in most cases. However, understanding the nuances of each can help you write cleaner, more efficient code. Consistency is key, so choose one style and stick with it throughout your project. By following best practices and being mindful of your quoting style, you can improve the readability and maintainability of your JavaScript code. Happy coding!
FAQ
-
What is the difference between single quotes and double quotes in JavaScript?
Single and double quotes can be used interchangeably to define strings in JavaScript, but they have different implications when it comes to including quote characters within strings. -
Can I use both single and double quotes in the same JavaScript file?
Yes, you can use both types of quotes in the same file, but it’s best practice to stick to one style for consistency. -
How do I escape quotes in a JavaScript string?
To escape quotes, use a backslash before the quote character you want to include within the string. For example:let str = 'It\'s a sunny day!';
. -
What should I do if I’m working with JSON data in JavaScript?
When working with JSON, always use double quotes for keys and string values, as this is required by the JSON format. -
Is there a preferred quote style in the JavaScript community?
There is no universally preferred style; it often depends on personal or team preference. However, many developers lean towards using single quotes for JavaScript strings.